Handling and Tracking Email Sending Failures in Spring
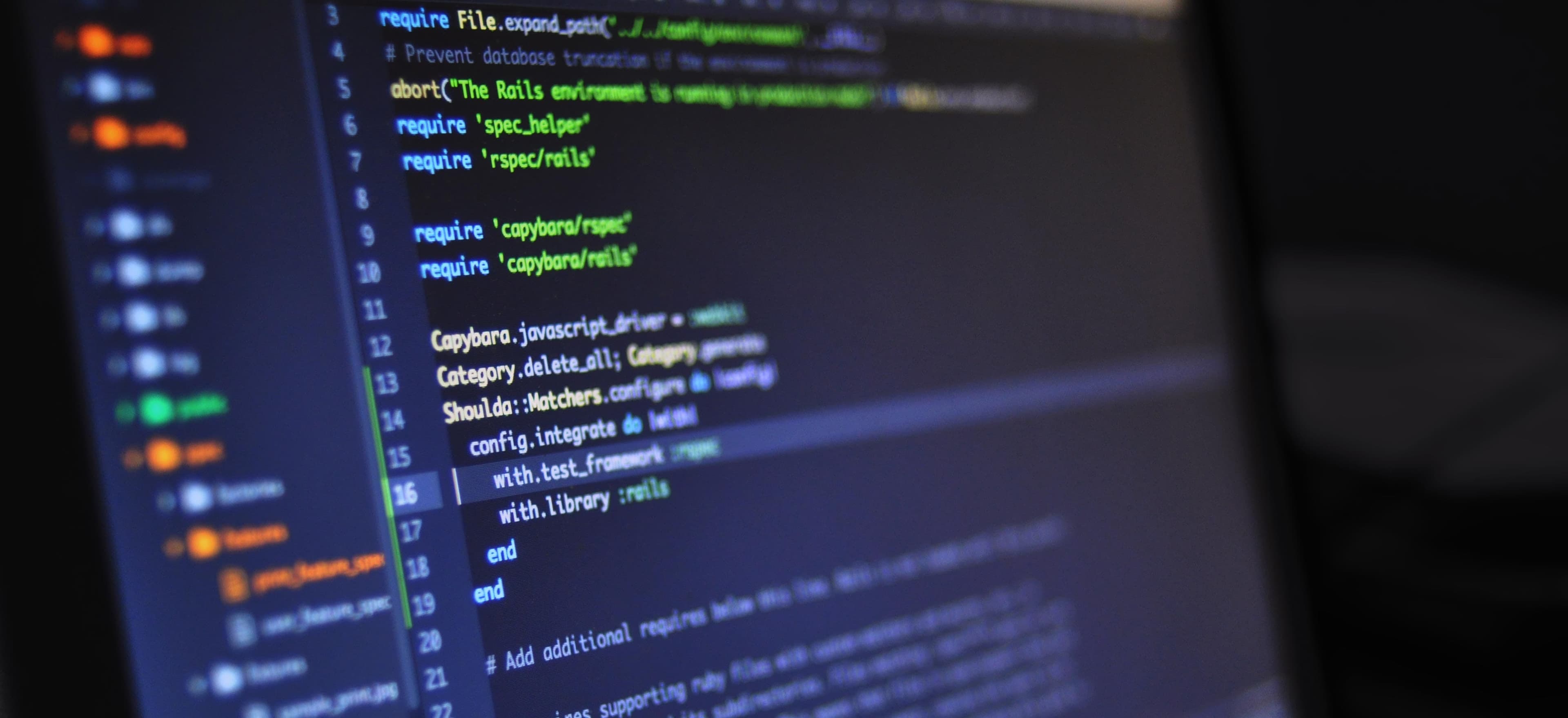
- Published on
Understanding Email Sending Failures in Spring
In any application that involves sending emails, it's imperative to handle and track email sending failures to ensure the reliability of the communication system. In a Spring-based application, this becomes even more crucial to maintain the robustness of the email sending mechanism. This article delves into the best practices for handling and tracking email sending failures in a Spring application.
Handling Failures Using Spring's JavaMailSender
When using Spring's JavaMailSender
to send emails, it's essential to handle potential failures that may occur during the process. These failures can range from network issues to misconfiguration of email properties, ultimately leading to the email not being delivered.
@Autowired
private JavaMailSender javaMailSender;
public void sendEmail(String to, String subject, String body) {
try {
MimeMessage message = javaMailSender.createMimeMessage();
MimeMessageHelper helper = new MimeMessageHelper(message, true);
helper.setTo(to);
helper.setSubject(subject);
helper.setText(body, true);
javaMailSender.send(message);
} catch (MailException ex) {
// Handle the mail sending exception
// Log the error or perform necessary actions
}
}
In the code snippet above, we use JavaMailSender
in combination with MimeMessage
and MimeMessageHelper
to send an email. The try-catch
block around javaMailSender.send(message)
allows us to catch any MailException
that may occur during the sending process. This enables us to handle the exception gracefully, for example, by logging the error or taking appropriate action.
Tracking Failures Using Spring's Actuator
Spring Boot Actuator provides several built-in endpoints for monitoring and managing your application. We can leverage these endpoints to track email sending failures and gain insights into the email sending process.
By enabling the mail
actuator endpoint, we can monitor the status and details of email sending.
management.endpoints.web.exposure.include=info,health,mail
management.endpoint.mail.enabled=true
After enabling the mail
endpoint, accessing the /actuator/mail
endpoint will provide information about the recent email sending attempts, including any failures and their corresponding error messages.
Retry Mechanism for Resending Failed Emails
Implementing a retry mechanism for resending failed emails is a proactive approach to mitigate email sending failures. By integrating Spring Retry, we can automatically retry the email sending process in case of transient failures.
@Retryable(
value = {MailSendException.class},
maxAttempts = 3,
backoff = @Backoff(delay = 1000, maxDelay = 3000)
)
public void sendEmailWithRetry(String to, String subject, String body) {
// Same email sending logic as before
// No need to explicitly handle the exception
}
In the code snippet above, we annotate the sendEmailWithRetry
method with @Retryable
and specify the exception to be retried (MailSendException
in this case), the maximum number of retry attempts, and the backoff parameters. This allows the method to be automatically retried in case of a MailSendException
.
Tracking Retries and Failures
To track the retries and failures of the email sending process, we can incorporate a logging framework like Logback or Log4j. By logging the retry attempts and failure details, we gain visibility into the behavior of the retry mechanism.
<logger name="org.springframework.retry" level="DEBUG"/>
In the above configuration for Logback, we set the log level for org.springframework.retry
package to DEBUG
to observe the retry attempts and related information.
Final Thoughts
Handling and tracking email sending failures in a Spring application is crucial for maintaining a reliable communication system. By proactively handling failures, leveraging Spring Actuator for monitoring, implementing a retry mechanism, and tracking retries and failures, we can ensure the robustness of the email sending process.
Incorporating these best practices not only improves the reliability of email communication but also provides insights into the behavior of the email sending mechanism, facilitating effective troubleshooting and proactive maintenance.
By following these guidelines, developers can enhance the resilience of their Spring-based email sending functionality and ensure smooth communication within their applications.
Checkout our other articles