Overcoming December's Java Challenges: A Tech Trek
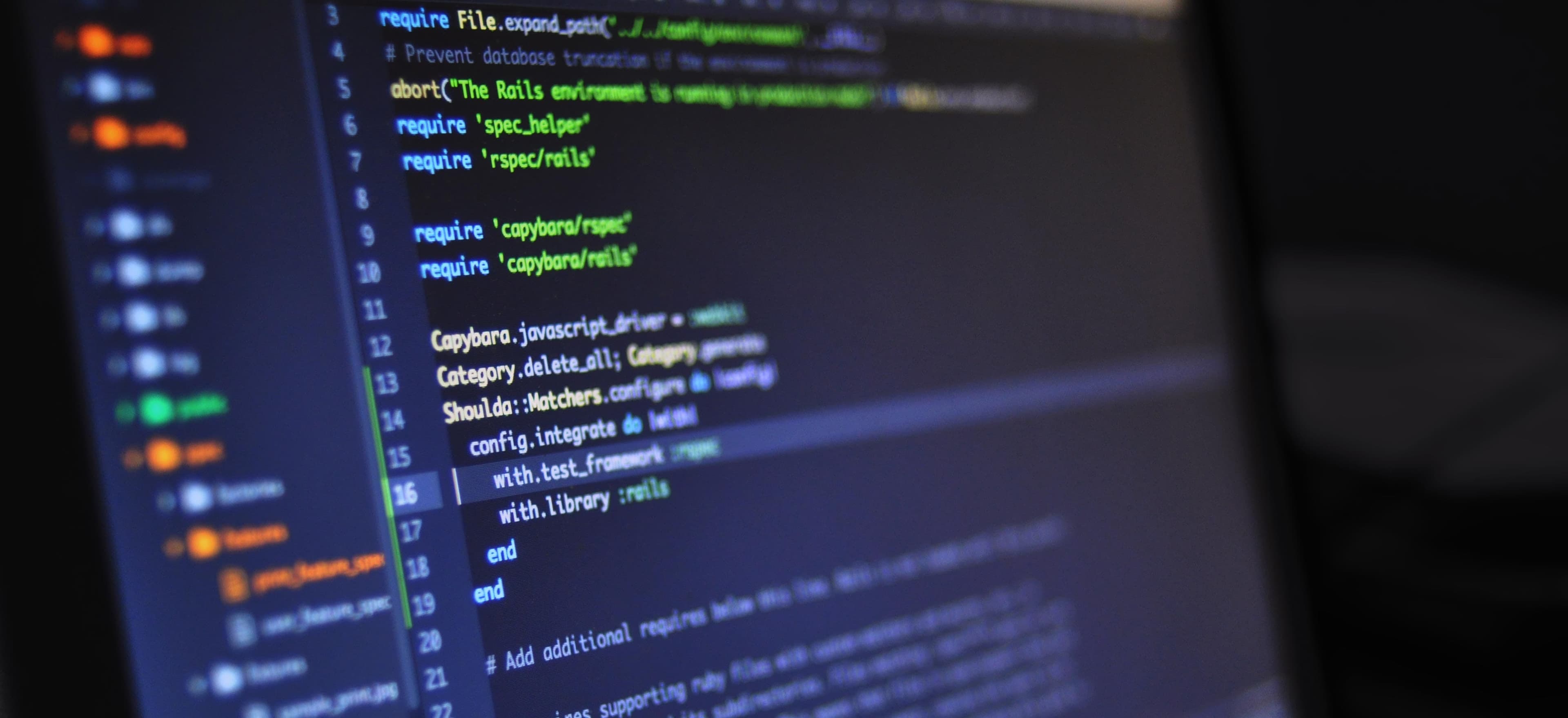
- Published on
Overcoming December's Java Challenges: A Tech Trek
As we wrap up another eventful year in the tech world, it's crucial to continually enhance our knowledge and skills. In the realm of Java, staying abreast of the latest developments, mastering complex concepts, and honing problem-solving abilities are key. Join me on this tech trek as we conquer some of December's challenging Java topics.
Embracing Java 14 Features
Java 14 introduced several intriguing features that can greatly enhance your coding experience. One such feature is the instanceof
pattern matching. This new feature allows you to streamline the process of casting and conditional logic.
Let's delve into an example to understand the benefits of this feature:
// Before Java 14
if (obj instanceof String) {
String s = (String) obj;
// work with s
}
// With Java 14
if (obj instanceof String s) {
// work with s directly
}
The latter approach not only reduces redundancy but also enhances readability. It's imperative to stay updated with the latest Java features and incorporate them into your codebase to boost efficiency and maintainability.
Mastering Java Multithreading
Multithreading is an integral aspect of Java programming, allowing concurrent execution of tasks. However, it also brings forth challenges such as race conditions and deadlocks.
Race Conditions: These occur when multiple threads access shared data concurrently, and at least one of them modifies the data. Utilizing synchronized blocks or java.util.concurrent
constructs like Atomic
classes can alleviate this issue.
Deadlocks: When two or more threads are blocked forever, each waiting for the other to release a resource, a deadlock arises. Employing a strategy such as imposing a strict ordering of resource acquisition or utilizing methods like tryLock
can help prevent deadlocks.
Understanding and effectively mitigating these challenges will elevate your proficiency in Java multithreading, enabling you to develop robust and responsive applications.
Grappling with Java Memory Management
Memory management is crucial in Java, as it directly impacts an application's performance and resource utilization. It's imperative to comprehend concepts such as garbage collection, memory leaks, and optimizing memory usage.
Garbage Collection: Java's automatic memory management system alleviates the burden of manual memory deallocation. However, understanding the different types of garbage collectors, tuning their configurations, and analyzing their impact on your application is essential for efficient memory management.
Memory Leaks: Identifying and rectifying memory leaks is paramount. Utilizing tools like Java VisualVM or profilers such as YourKit can aid in detecting and resolving memory leaks, thereby enhancing the stability and performance of your applications.
Strategically managing memory in Java applications is pivotal for achieving optimal performance and scalability.
Leveraging Java Frameworks and Libraries
In today's software development landscape, leveraging frameworks and libraries can significantly expedite the development process and enhance the functionality of your applications.
Spring Framework: The Spring framework offers a comprehensive suite of features for developing robust Java applications. From inversion of control (IoC) and aspect-oriented programming (AOP) to Spring Data and Spring Security, this framework empowers developers to create scalable and secure applications with ease.
Guava Library: Google's Guava library provides a plethora of utilities and extension classes that augment the Java core libraries. Whether it's working with collections, caching, or concurrency, Guava simplifies and enriches Java development.
Incorporating popular Java frameworks and libraries enables you to capitalize on existing robust solutions, empowering you to focus on implementing business logic and value-added features.
Embracing Clean Code and Best Practices
Writing clean, maintainable code is indispensable in Java development. Adhering to best practices and design principles not only enhances code readability and maintainability but also fosters collaboration within development teams.
Effective Naming Conventions: Meaningful and consistent naming of variables, methods, and classes improves code comprehension and maintainability.
Applying Design Patterns: Leveraging design patterns such as Singleton, Factory, and Observer enhances code modularity and reusability, fostering a more structured and organized codebase.
Striving for clean code not only elevates the quality of your codebase but also facilitates seamless integration of new features and debugging, contributing to an efficient and scalable code architecture.
Final Thoughts
As we navigate through December's Java challenges, it's crucial to remain proactive in advancing our Java proficiency. By embracing new features, mastering multithreading, optimizing memory management, leveraging frameworks and libraries, and embracing clean code practices, we fortify our Java prowess and prepare ourselves for the exciting tech journey ahead.
Let's continue our tech trek, armed with enhanced Java skills, as we step into the new year filled with boundless opportunities and rewarding technological endeavors.
Remember, the journey of mastering Java is a continuous pursuit, and with perseverance and diligent learning, we can conquer the ever-evolving landscape of Java development.
So gear up, embrace the challenges, and embark on this exhilarating Tech Trek towards Java mastery!