5 Challenges in Creating a Weather Forecast App
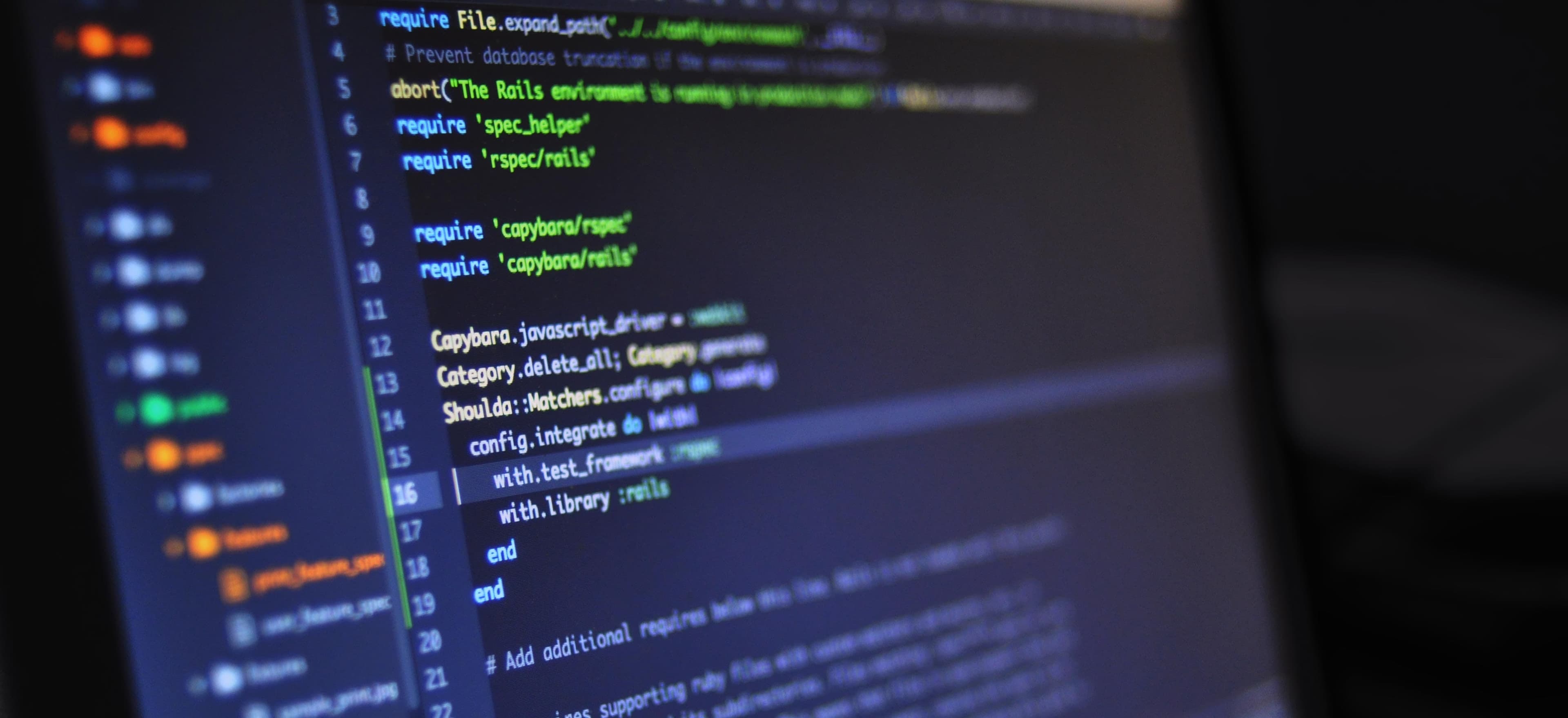
- Published on
5 Challenges in Creating a Weather Forecast App
In today's era, weather forecast applications are some of the most widely used applications by people all over the world. With the increasing demand and competition in the market, developing a weather forecast app comes with its own set of challenges. In this blog post, we will explore the top 5 challenges in creating a weather forecast app and discuss how to tackle them using Java.
1. Data Integration and API Consumption
Integrating with various weather data providers and consuming their APIs to retrieve accurate and timely weather data is a critical challenge. In Java, this can be addressed by leveraging libraries like Retrofit or Apache HttpClient to make HTTP requests and handle API responses efficiently. For example, using Retrofit makes it easy to define and execute API requests, automatically serialize/deserialize data, and handle errors.
// Using Retrofit to create a service for weather API
interface WeatherApiService {
@GET("weather/{city}")
Call<WeatherResponse> getWeatherData(@Path("city") String city);
}
2. Data Processing and Caching
Processing the retrieved weather data, such as parsing complex JSON/XML responses and caching the data for offline access, presents a significant challenge. Java provides libraries like Gson and Jackson for JSON parsing, while libraries like Ehcache or Caffeine can be utilized for efficient in-memory caching. By integrating these libraries, developers can handle data processing and caching seamlessly.
// Parsing JSON response using Gson
Gson gson = new Gson();
WeatherData weather = gson.fromJson(jsonResponse, WeatherData.class);
// Using Ehcache for caching weather data
Cache<String, WeatherData> weatherCache = cacheManager.getCache("weatherCache");
weatherCache.put(city, weather);
3. User Interface and Data Visualization
Designing an intuitive user interface to present the weather forecast information in a visually appealing and easily understandable manner is crucial. JavaFX can be employed for creating rich and interactive user interfaces. Additionally, libraries like JFreeChart or JavaFX Charts can be utilized for data visualization, enabling developers to display weather trends and forecasts through graphical representations.
// Creating a line chart using JavaFX Charts
LineChart<Number, Number> lineChart = new LineChart<>(xAxis, yAxis);
lineChart.setTitle("Weather Forecast");
// Add data series and styling
4. Location-Based Services and Permissions
Incorporating location-based services and obtaining user permissions to access their location data adds complexity to the app development process. Java's Location API and Android's LocationManager can be integrated to retrieve the device's location, while requesting and handling runtime permissions using the Android Permissions API ensures a seamless user experience.
// Requesting location permissions in Android
if (ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION)
!= PackageManager.PERMISSION_GRANTED) {
// Request the permission
ActivityCompat.requestPermissions(this,
new String[]{Manifest.permission.ACCESS_FINE_LOCATION},
REQUEST_LOCATION_PERMISSION);
}
5. Battery Consumption and Background Updates
Efficiently managing battery consumption while providing timely weather updates in the background is a significant challenge. Employing job scheduling techniques with Java's JobScheduler or WorkManager can help in optimizing background updates, ensuring minimal impact on the device's battery life while keeping the weather data up to date.
// Implementing periodic background updates using WorkManager
PeriodicWorkRequest weatherUpdateRequest =
new PeriodicWorkRequest.Builder(WeatherUpdateWorker.class, 1, TimeUnit.HOURS)
.setConstraints(Constraints.NONE)
.build();
WorkManager.getInstance(context).enqueue(weatherUpdateRequest);
In conclusion, creating a weather forecast app involves overcoming several challenges related to data integration, processing, user interface, location services, and battery consumption. By leveraging the capabilities of Java and integrating relevant libraries and APIs, developers can navigate these challenges effectively, resulting in a robust and reliable weather forecast application.
References:
- Retrofit: Retrofit
- Gson: Gson
- Ehcache: Ehcache
- JavaFX Charts: JavaFX Charts
- Android Location: Location API
- WorkManager: WorkManager