Securing Cloud-Native Apps: The JWT Dilemma Unraveled
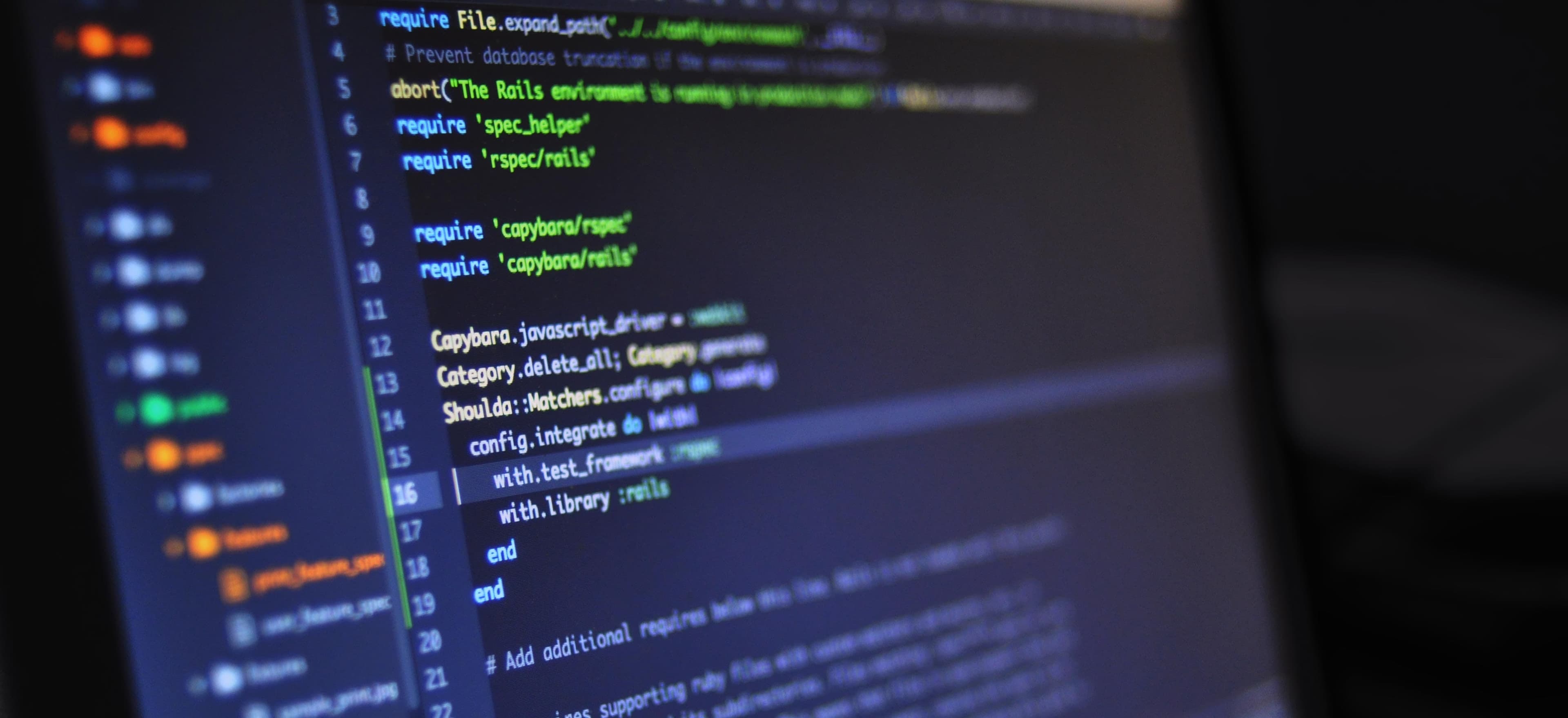
- Published on
Securing Cloud-Native Apps: The JWT Dilemma Unraveled
The Roadmap
Brief Overview:
In the ever-evolving landscape of application development, the advent of cloud-native applications has revolutionized the way software is designed, developed, and deployed. These applications, harnessing the power of cloud computing, are inherently dynamic, scalable, and modular. However, ensuring the security of cloud-native apps poses a unique set of challenges. One solution that has gained prominence in this domain is the use of JSON Web Tokens (JWT) for securing API services.
Purpose of the Article:
The purpose of this article is to dissect the complexities of using JWT for securing cloud-native applications. We will explore the challenges faced in implementing JWT, discuss the potential pitfalls, and provide best practices for secure implementation.
What Are Cloud-Native Applications?
Definition and Characteristics:
Cloud-native applications are built specifically to leverage the elasticity and scalability of cloud environments. These applications are designed with modularity, resilience, and agility in mind, breaking down large, monolithic systems into smaller, independent services. They are often packaged in containers, orchestrated dynamically, and are designed for continuous delivery.
Security Challenges:
The dynamic nature of cloud-native applications introduces new security challenges, such as securing service-to-service communication, ensuring proper authentication and authorization, and managing external access protocols amid the distributed and microservices-based architecture.
The Role of JWT in Securing Cloud-Native Apps
Introduction to JWT:
JSON Web Tokens are a compact, URL-safe means of representing claims to be transferred between two parties. A JWT consists of three parts: the header, the payload, and the signature. It is preferred for securing web applications due to its stateless nature and ease of integration.
Advantages:
- Stateless Authentication: JWTs enable stateless API authentication, eliminating the need to store session information on the server.
- Scalability: They are well-suited for distributed and microservices-based architectures, promoting scalability.
Common Use Cases:
- Single Sign-On (SSO): JWTs are commonly used in single sign-on systems where a user’s identity is shared across multiple services.
- Service-to-Service Authentication: JWTs facilitate secure communication between microservices, enabling secure verification of the calling service's identity.
The JWT Dilemma
Introduction to the Dilemma:
While JWT offers several advantages, its implementation in cloud-native applications poses inherent complexities. Challenges like token revocation, key management, and improper validation can lead to security vulnerabilities and compromises.
Security Risks:
- Weak Signatures: Improperly implemented JWTs can be vulnerable to signature manipulation, leading to unauthorized access.
- Insufficient Validation: Inadequate validation of the JWT's claims and signatures can result in exploitation of security vulnerabilities.
Implementing JWT Securely
Best Practices:
- Strong Signing Algorithms: Utilize robust signing algorithms, such as RS256, to ensure the security and integrity of JWTs.
- Token Expiry Management: Implement well-defined strategies to manage token expiry and renewal, ensuring that expired tokens are promptly invalidated.
Token Management:
Token management can address key challenges in JWT implementation. Strategies like short-lived tokens, token blacklisting, and token introspection can enhance security and mitigate risks. Leveraging external identity providers for token management can also be an effective strategy.
Example with Code Snippets:
// Sample code for generating a JWT token using jjwt library
String secretKey = "your_secret_key";
String token = Jwts.builder()
.setSubject("user123")
.claim("role", "admin")
.setIssuedAt(new Date())
.setExpiration(new Date(System.currentTimeMillis() + 3600000))
.signWith(SignatureAlgorithm.HS256, secretKey)
.compact();
// Sample code for JWT validation
try {
Jws<Claims> claims = Jwts.parser()
.setSigningKey(secretKey)
.parseClaimsJws(token);
// Successfully parsed - token is valid
} catch (JwtException e) {
// Invalid token
}
Real-World Application: Case Study
Intro to the Case Study:
Consider a cloud-native e-commerce platform that leverages microservices architecture. The platform faced challenges in ensuring secure authentication and authorization across its distributed services.
Challenges and Solutions:
The platform encountered difficulties in managing token revocation and ensuring the integrity of the JWT signatures. By implementing short-lived tokens, robust key management, and strict validation practices, the platform successfully addressed these challenges.
Insights and Outcomes:
The adoption of JWT significantly enhanced the platform’s security posture. It streamlined user authentication, fortified service-to-service communication, and provided operational agility, leading to improved security and operational efficiency.
The Last Word
Recap:
In conclusion, securing cloud-native applications presents unique challenges, and JWT has emerged as a valuable tool in addressing these challenges. However, the implementation of JWT necessitates careful consideration of best practices to mitigate potential risks.
Final Thoughts:
As cloud-native architecture continues to evolve, the role of JWT in securing these applications remains pivotal. By adhering to best practices and leveraging advanced token management strategies, organizations can fortify their cloud-native applications against potential security threats.
Further Reading and Resources
Complementary Technologies:
In addition to JWT, technologies such as OAuth and OpenID Connect complement the security framework of cloud-native applications, providing comprehensive identity and access management capabilities.
Learning Resources:
- JWT Official Documentation
- Cloud-Native Security Best Practices
- Securing Microservices with JWT