OAuth2 Tokens: Securing REST APIs from Token Theft
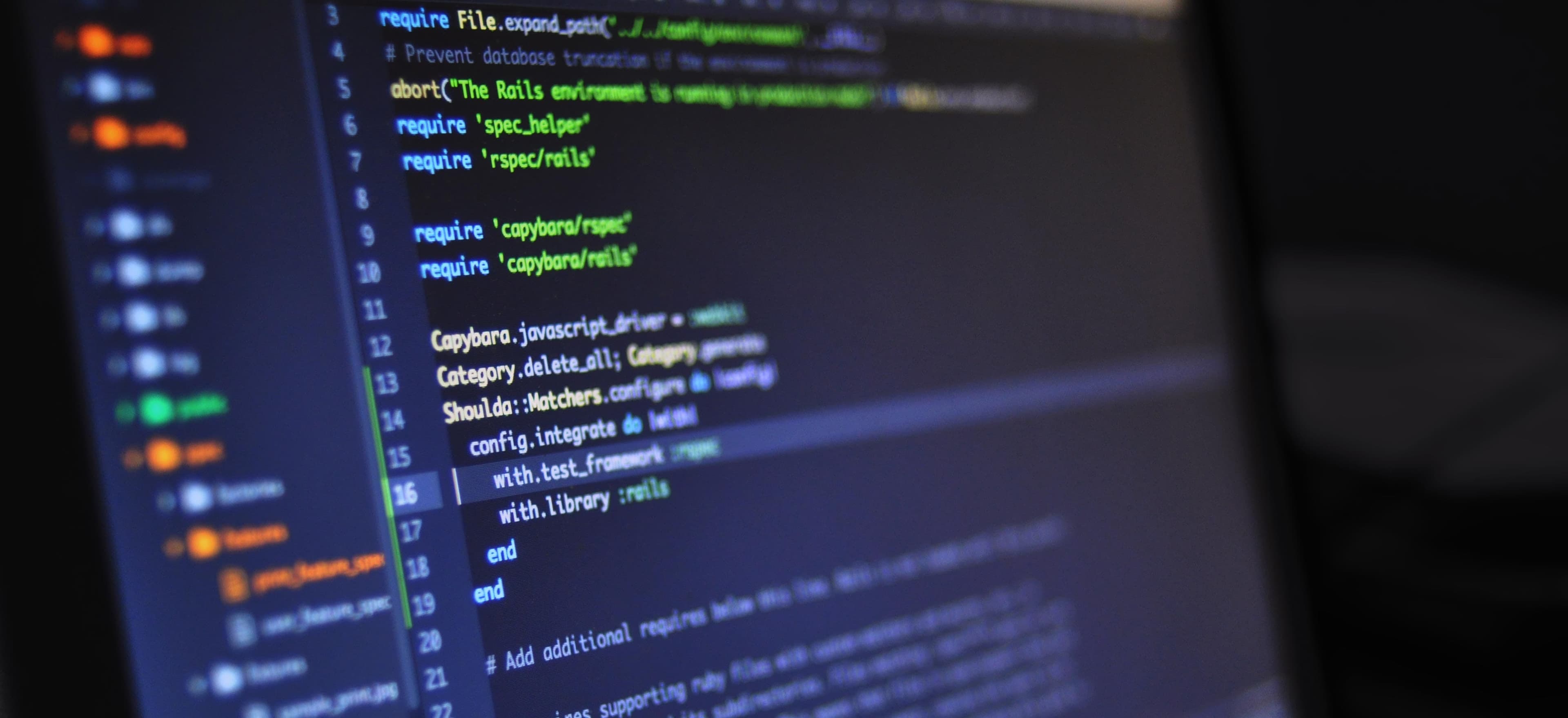
- Published on
Securing REST APIs from Token Theft using OAuth2 Tokens
In today's fast-paced world, security is paramount in all aspects of software development. When it comes to building REST APIs, safeguarding access to resources is crucial to prevent unauthorized access and data breaches. One of the most widely adopted methods for securing REST APIs is through the use of OAuth2 tokens.
In this article, we will delve into the world of OAuth2 tokens, understanding their significance in securing REST APIs, and best practices for preventing token theft.
Understanding OAuth2 Tokens
OAuth2 is an authorization framework that enables applications to obtain limited access to a user's data on a server. OAuth2 tokens serve as the key to access protected resources using various flows such as authorization code, implicit, client credentials, and resource owner password credentials.
Types of OAuth2 Tokens
Access Tokens
- Access tokens are used to access protected resources and typically have a limited lifespan.
- They are scoped to specific resources and are obtained after successful authentication and authorization.
Refresh Tokens
- Refresh tokens are used to obtain new access tokens once the current access token expires.
- They have a longer lifespan compared to access tokens and should be securely stored.
Preventing Token Theft
Use HTTPS
Always ensure that your REST API endpoints are accessible over HTTPS. This prevents man-in-the-middle attacks and eavesdropping, reducing the risk of token theft.
Token Encryption
Encrypting tokens before transmitting them adds an extra layer of security. Using techniques such as JSON Web Tokens (JWT) and encryption algorithms ensures that tokens are secure in transit and at rest.
Token Validation
Before accepting and processing an access token, validate its authenticity and integrity. This can include checking the token signature, issuer, and expiration to mitigate the risk of accepting tampered or invalid tokens.
Implement Rate Limiting
Enforce rate limiting to prevent brute force attacks on token endpoints. This limits the number of requests a client can make within a given timeframe, reducing the risk of unauthorized token acquisition.
Secure Token Storage
Whether storing tokens in databases or in-memory caches, ensure that they are securely stored. Utilize encryption and access controls to prevent unauthorized access to tokens.
Token Revocation
Implement mechanisms to revoke access tokens if suspicious activity is detected or if a user revokes access. This prevents stolen tokens from being misused.
Token Rotation
Periodically rotate access and refresh tokens to limit their lifespan and reduce the impact of token theft. This can be achieved by implementing token refresh flows and issuing new tokens upon expiration.
Role-Based Access Control
Incorporate role-based access control (RBAC) to restrict access to resources based on users' roles and permissions. This ensures that even if a token is compromised, the unauthorized access to sensitive resources is mitigated.
Sample Code Illustration
Let's consider a hypothetical scenario where an OAuth2 token is being utilized to access a protected resource in a Java-based REST API.
@RestController
public class ProtectedResourceController {
@GetMapping("/protected/resource")
public ResponseEntity<String> getProtectedResource(@RequestHeader("Authorization") String accessToken) {
if (isValidAccessToken(accessToken)) {
// Logic to fetch and return the protected resource
return ResponseEntity.ok("Protected resource content");
} else {
return ResponseEntity.status(HttpStatus.UNAUTHORIZED).build();
}
}
private boolean isValidAccessToken(String accessToken) {
// Logic to validate the access token
// Example: Token validation against issuer, signature, and expiration
return TokenValidator.validate(accessToken);
}
}
In the above code snippet, the ProtectedResourceController
demonstrates the validation of the access token before serving the protected resource. The isValidAccessToken
method invokes a TokenValidator
to ensure the integrity and validity of the access token.
Bringing It All Together
OAuth2 tokens play a pivotal role in securing REST APIs from unauthorized access and data breaches. By leveraging best practices such as token encryption, validation, revocation, and role-based access control, developers can mitigate the risk of token theft and enhance the overall security of their REST APIs.
Remember, securing REST APIs is an ongoing process, and staying updated with the latest security standards and best practices is paramount to safeguarding sensitive data and resources.
Incorporating OAuth2 tokens effectively ensures a robust layer of security for your REST APIs, paving the way for safe and reliable interactions between clients and resources. Always prioritize security in your development endeavors, and OAuth2 tokens will serve as the guardians of your valuable assets.
To delve further into OAuth2 security, learn about OpenID Connect for identity layer built on top of OAuth2.
Checkout our other articles