Overcoming Common ListenAbleFuture Callback Hurdles
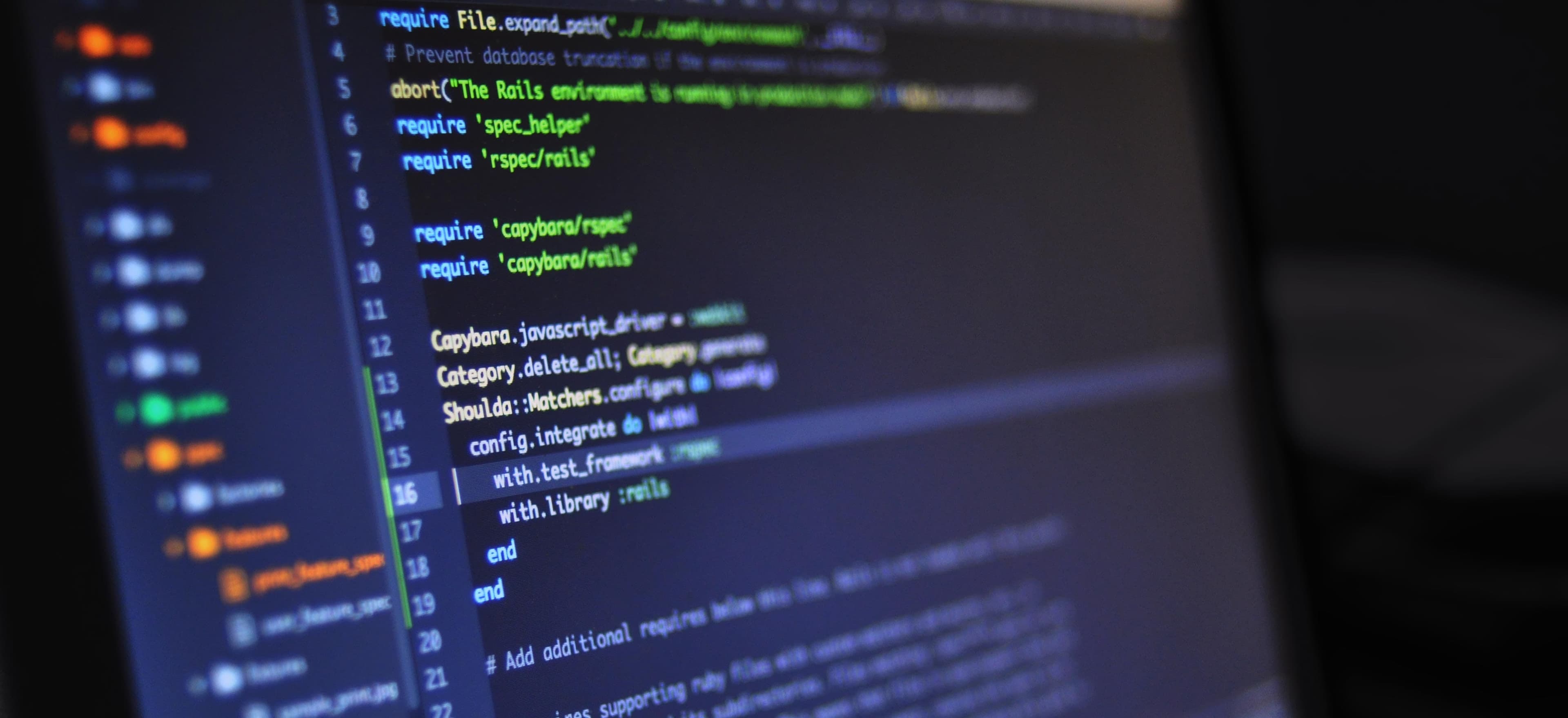
- Published on
Overcoming Common ListenableFuture
Callback Hurdles
When working with asynchronous programming in Java, especially in modern frameworks like Spring or Guava, you might come across the ListenableFuture
interface. This versatile interface allows you to work with asynchronous computation and handle the callbacks when the computation is completed. However, like any powerful tool, there are common hurdles that developers encounter when using ListenableFuture
callbacks.
In this article, we'll discuss these common hurdles and provide solutions to overcome them, helping you to leverage the full potential of ListenableFuture
in your Java projects.
Understanding ListenableFuture
Before diving into the common hurdles, let's first understand what ListenableFuture
is and its significance in the Java ecosystem. ListenableFuture
is part of the Guava library and is also integrated in the Spring Framework through the ListenableFutureCallback
interface. Essentially, it represents the result of an asynchronous computation and allows you to register callbacks to be executed once the computation is complete.
Example Code:
Let's start by looking at a simple example of using ListenableFuture
in Java:
import com.google.common.util.concurrent.ListenableFuture;
import com.google.common.util.concurrent.ListeningExecutorService;
import com.google.common.util.concurrent.MoreExecutors;
import java.util.concurrent.Executors;
public class ListenableFutureExample {
public static void main(String[] args) {
ListeningExecutorService service = MoreExecutors.listeningDecorator(Executors.newFixedThreadPool(10));
ListenableFuture<Integer> future = service.submit(() -> 5);
future.addListener(() -> {
try {
System.out.println("Result: " + future.get());
} catch (Exception e) {
e.printStackTrace();
}
}, service);
}
}
In this example, we create a ListenableFuture
using a ListeningExecutorService
and submit a simple computation that returns the integer 5
. We then add a listener to the future to handle the result once the computation is complete.
Common ListenableFuture
Callback Hurdles
1. Unhandled Exceptions
One of the common issues when working with ListenableFuture
is dealing with unhandled exceptions. It's essential to handle exceptions properly to prevent them from bubbling up and causing unexpected behavior in your application.
Solution:
To handle exceptions in ListenableFuture
callbacks, you can use the Futures
utility class provided by Guava. The addCallback
method allows you to define separate callbacks for success and failure scenarios, making it easier to handle exceptions in a structured manner.
import com.google.common.util.concurrent.Futures;
import com.google.common.util.concurrent.FutureCallback;
import com.google.common.util.concurrent.ListenableFuture;
import com.google.common.util.concurrent.MoreExecutors;
import java.util.concurrent.Executors;
public class ExceptionHandlingExample {
public static void main(String[] args) {
ListenableFuture<Integer> future = ... // obtain ListenableFuture
Futures.addCallback(future, new FutureCallback<Integer>() {
public void onSuccess(Integer result) {
System.out.println("Result: " + result);
}
public void onFailure(Throwable t) {
System.err.println("Error: " + t.getMessage());
}
}, MoreExecutors.directExecutor());
}
}
In this example, we use the Futures.addCallback
method to define separate onSuccess
and onFailure
callbacks, providing better control over handling exceptions.
2. Chaining ListenableFuture
Instances
Another common hurdle is chaining multiple ListenableFuture
instances to execute sequentially or in a specific order. This is often necessary when one asynchronous computation depends on the result of another.
Solution:
The Futures
utility class also provides a solution for chaining ListenableFuture
instances using the transform
and transformAsync
methods. These methods allow you to create a new ListenableFuture
that depends on the outcome of the original future, enabling you to chain asynchronous computations in a structured manner.
import com.google.common.util.concurrent.Futures;
import com.google.common.util.concurrent.ListenableFuture;
public class ChainingExample {
public static void main(String[] args) {
ListenableFuture<Integer> originalFuture = ... // obtain original ListenableFuture
ListenableFuture<String> transformedFuture = Futures.transform(originalFuture, input -> {
// perform transformation and return a new value
return "Transformed result: " + input;
}, MoreExecutors.directExecutor());
}
}
Here, we use the Futures.transform
method to create a new ListenableFuture
that depends on the result of the original future. This allows for seamless chaining of asynchronous computations.
My Closing Thoughts on the Matter
In conclusion, ListenableFuture
is a powerful interface for working with asynchronous computation in Java. By understanding common hurdles and utilizing the solutions provided by the Guava library, you can effectively handle callbacks, manage exceptions, and chain asynchronous computations with ease.
Asynchronous programming is becoming increasingly important in modern Java development, and mastering tools like ListenableFuture
is essential for building robust and efficient applications.
Now that you have a better understanding of ListenableFuture
and how to overcome common callback hurdles, you can leverage its capabilities to write more resilient and responsive Java applications.
If you're interested in diving deeper into asynchronous programming with Java, you may find this article on Java CompletableFuture useful for further exploration.
Happy coding!
Checkout our other articles