Heaps Algorithm Demystified: Solving Permutation Puzzles
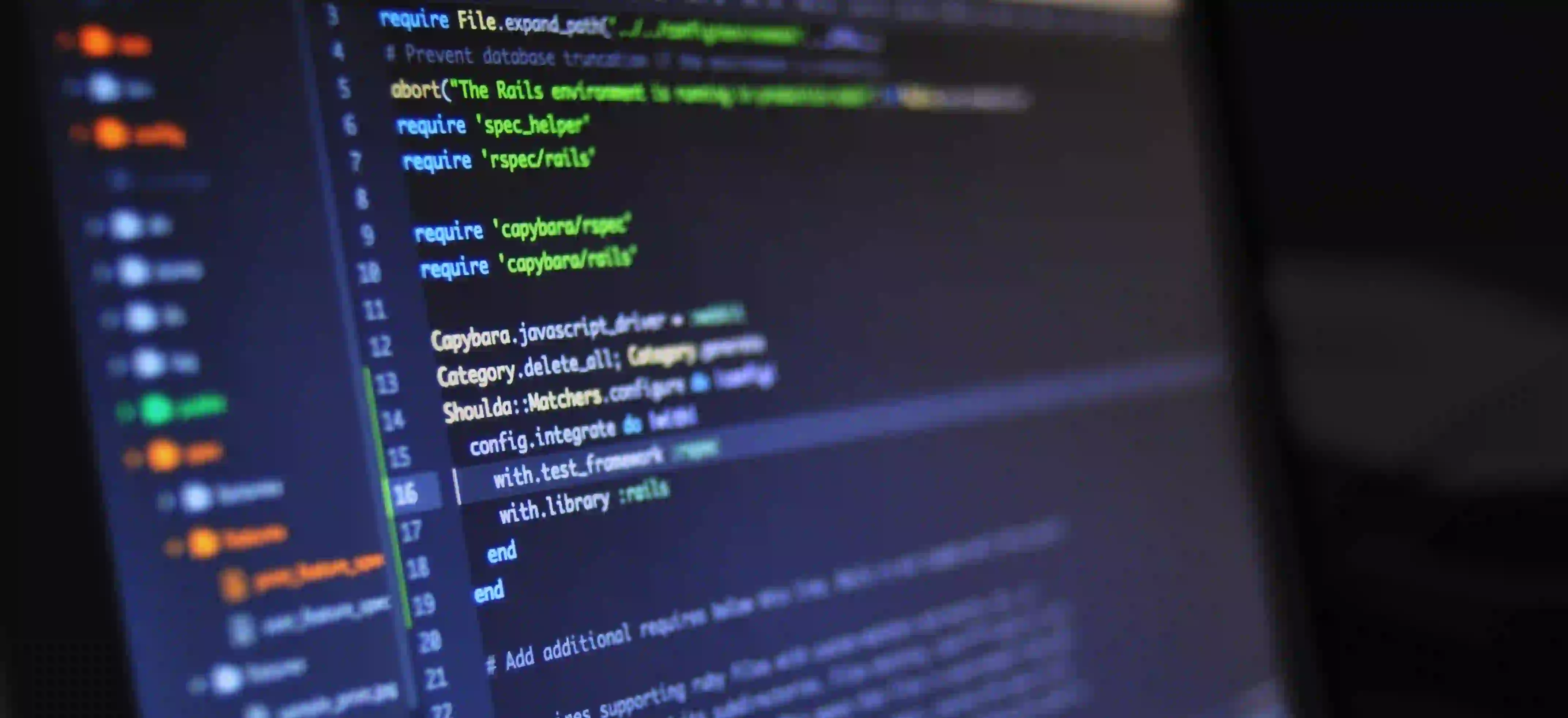
Heaps Algorithm Demystified: Solving Permutation Puzzles
Permutation puzzles are a common problem in various domains of computer science, from generating all possible arrangements of a set of elements to solving anagrams and cryptography. One popular algorithm for generating permutations is Heap's algorithm, known for its elegance and efficiency. In this blog post, we will dissect Heap's algorithm, understand its inner workings, and discuss its implementation in the Java programming language.
Understanding Permutations
Before delving into Heap's algorithm, let's take a moment to understand what permutations are. In mathematics, a permutation of a set is an arrangement of its elements in a specific order. For example, given the set 3, permutations include 3, 2, 3, and so on. Generating all possible permutations of a set is a common problem encountered in computer science.
Heap's Algorithm: The Overview
Heap's algorithm, devised by B. R. Heap in 1963, is a method for generating all possible permutations of a set. Its beauty lies in its simplicity and efficiency, making it a popular choice among programmers. The algorithm generates permutations in-place, meaning it does not require additional space proportional to the input size.
The Java Implementation
Let's now explore a Java implementation of Heap's algorithm for generating permutations. Below is the Java code implementing the Heap's algorithm for generating permutations of an array of integers.
public class HeapPermutation {
// Method to swap elements at indices i and j in the array
private void swap(int[] array, int i, int j) {
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
// Method to print the array
private void printArray(int[] array) {
for (int value : array) {
System.out.print(value + " ");
}
System.out.println();
}
// Method to generate permutations using Heap's algorithm
public void heapPermutation(int[] array, int size, int n) {
if (size == 1) {
printArray(array);
} else {
for (int i = 0; i < size; i++) {
heapPermutation(array, size - 1, n);
if (size % 2 == 1) {
swap(array, 0, size - 1);
} else {
swap(array, i, size - 1);
}
}
}
}
// Main method to test the Heap's algorithm
public static void main(String[] args) {
int[] array = {1, 2, 3};
int n = array.length;
HeapPermutation permutation = new HeapPermutation();
permutation.heapPermutation(array, n, n);
}
}
The HeapPermutation
class contains three methods: swap
, printArray
, and heapPermutation
. The swap
method swaps the elements at two indices in the array, the printArray
method prints the array, and the heapPermutation
method generates permutations using Heap's algorithm. In the main
method, we create an instance of HeapPermutation
and call the heapPermutation
method to generate and print all permutations of the array 3.
Understanding the Code
The swap
Method
The swap
method is a simple utility used to swap two elements in the array. It takes the array and two indices i
and j
as parameters and swaps the elements at those indices.
The printArray
Method
The printArray
method iterates through the array and prints its elements separated by a space.
The heapPermutation
Method
The heart of the algorithm lies in the heapPermutation
method. This method recursively generates permutations using Heap's algorithm. When the size of the permutation is 1, it prints the array. Otherwise, it generates permutations for the subarray and swaps elements based on a conditional check.
Why Heap's Algorithm?
Heap's algorithm stands out for its elegance and efficiency. Unlike many other permutation algorithms, Heap's algorithm generates permutations in-place, without requiring additional space. This makes it highly efficient, especially for large input sizes.
Heap's algorithm also has a time complexity of O(n!), where n is the number of elements in the input array. Despite the factorial time complexity, Heap's algorithm often outperforms other permutation algorithms due to its efficient in-place nature.
In Conclusion, Here is What Matters
Heap's algorithm is a remarkable method for generating permutations, known for its simplicity and efficiency. Its elegant recursive approach enables it to generate permutations in-place, making it an excellent choice for solving permutation puzzles in Java.
In this blog post, we dissected Heap's algorithm, explored its Java implementation, and gained an understanding of its inner workings. Armed with this knowledge, you can employ Heap's algorithm to solve various permutation problems in your Java projects. Happy permuting!
To further expand your understanding of permutation algorithms, you may want to delve into other permutation-related algorithms such as Johnson-Trotter algorithm and Steinhaus-Johnson-Trotter algorithm.
Now that you've grasped the inner workings of Heap's algorithm, you're well-equipped to tackle permutation problems with confidence in your Java projects. Happy coding!
Disclaimer: In certain scenarios, depending on the specific requirements and constraints, there might be alternative algorithms or specific optimizations more suitable than Heap's algorithm.
Whether you're solving anagrams, generating all possible combinations, or working on a permutation-based problem, comprehending Heap's algorithm can deepen your understanding of how to efficiently tackle permutation-related challenges in Java and beyond. Happy coding!