Creating a Jaw-Dropping JavaFX Form with Tron Effect
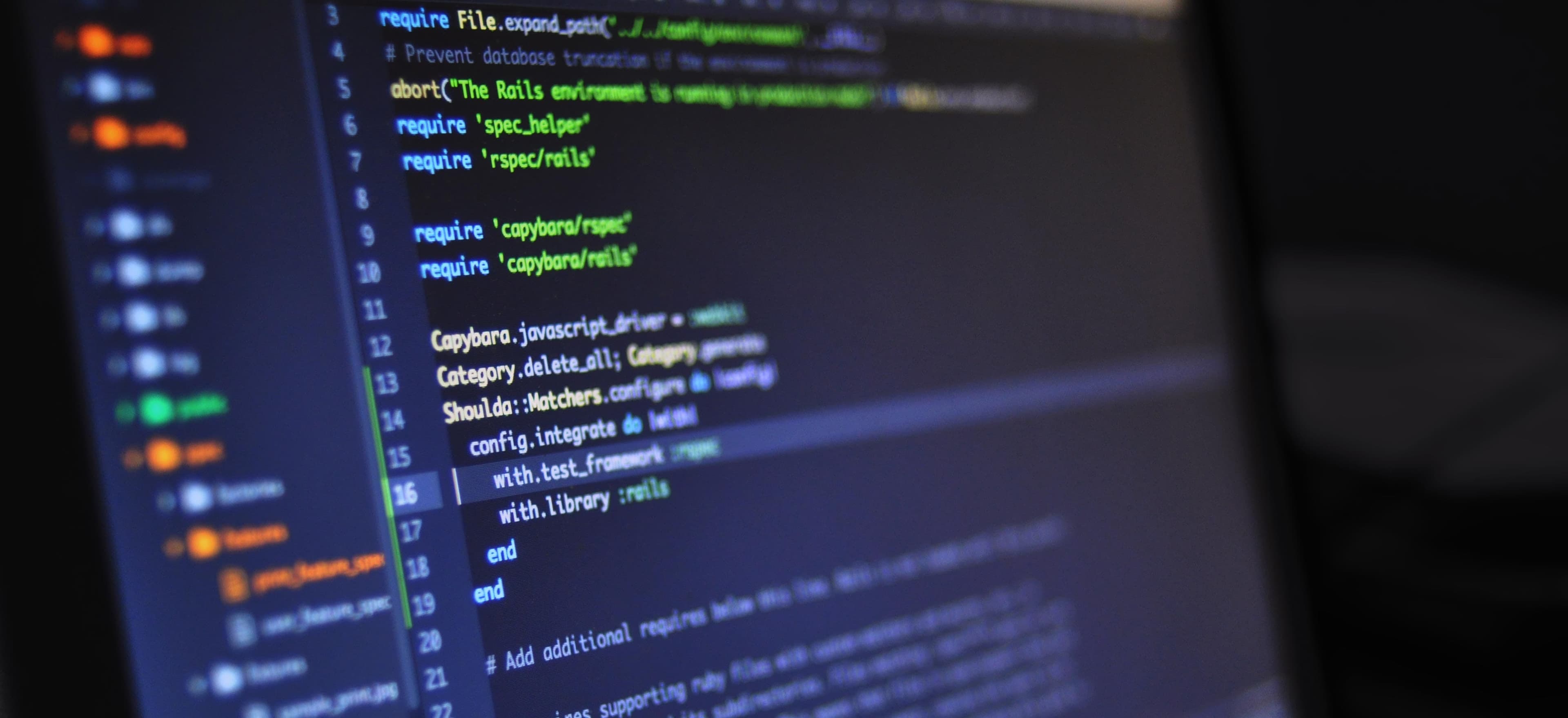
- Published on
In today's digital age, creating visually stunning user interfaces has become a significant part of software development. JavaFX, with its rich set of tools and libraries, provides developers with the necessary means to craft captivating user interfaces. In this tutorial, we will delve into the process of building a JavaFX form that incorporates a mesmerizing Tron effect. This effect will not only enhance the overall aesthetics but also showcase the potential of JavaFX in creating immersive user experiences.
Setting Up the Project
Before we dive into the code, let's set up our JavaFX project. We'll be using Maven to manage dependencies and build the project structure. Ensure that you have the Java Development Kit (JDK) and Maven installed on your system.
Create a new Maven project using the following command:
mvn archetype:generate -DgroupId=com.example -DartifactId=javaFX-tron-form -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
Once the project is created, navigate to the project directory and open it in your preferred Integrated Development Environment (IDE).
Adding JavaFX Dependencies
To start with JavaFX, we need to include its dependencies in the project's pom.xml
file. Add the following within the <dependencies>
section:
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-controls</artifactId>
<version>16</version>
</dependency>
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-fxml</artifactId>
<version>16</version>
</dependency>
After adding the dependencies, save the pom.xml
file and let Maven resolve the dependencies by running the command:
mvn clean install
Creating the JavaFX Form
Now, let's move on to creating our JavaFX form. We'll start by defining the UI layout in an FXML file. Create a new file named form.fxml
in the src/main/resources
directory and populate it with the following code:
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.control.*?>
<?import javafx.scene.layout.*?>
<?import javafx.geometry.Insets?>
<BorderPane fx:controller="com.example.FormController"
xmlns:fx="http://javafx.com/fxml"
prefWidth="400" prefHeight="300">
<center>
<VBox alignment="CENTER" spacing="10">
<TextField promptText="Username" />
<PasswordField promptText="Password" />
<Button text="Login" />
</VBox>
</center>
</BorderPane>
In this FXML layout, we've defined a simple form with a username TextField
, a password PasswordField
, and a Button
for login. The entire UI is wrapped in a BorderPane
, which will be the root element of our form.
Implementing the Controller
Next, we'll create the controller class that will handle the logic and event handling for our form. Create a new Java class named FormController
in the com.example
package and implement it as follows:
package com.example;
import javafx.fxml.FXML;
import javafx.scene.control.PasswordField;
import javafx.scene.control.TextField;
public class FormController {
@FXML
private TextField usernameField;
@FXML
private PasswordField passwordField;
public void login() {
String username = usernameField.getText();
String password = passwordField.getText();
// Implement your authentication logic here
}
}
In the controller class, we've annotated the TextField
and PasswordField
with @FXML
to indicate that they will be injected from the FXML file. We've also defined a login
method that will be called when the login Button
is clicked. Inside the login
method, you can implement the authentication logic as per your requirements.
Adding the Tron Effect
Now comes the most exciting part – adding the Tron effect to our JavaFX form. We'll use the Glow
effect to achieve the distinctive neon glow reminiscent of the Tron movie. Update the FXML layout to include the Glow
effect as shown below:
<VBox alignment="CENTER" spacing="10">
<effect>
<Glow level="0.7" />
</effect>
<TextField promptText="Username" />
<PasswordField promptText="Password" />
<Button text="Login" />
</VBox>
By applying the Glow
effect to the entire VBox
, we create a captivating visual impact that sets our form apart.
Running the Application
To see our JavaFX form in action, we need to create the main application class. Create a new Java class named MainApp
in the com.example
package and implement the entry point like this:
package com.example;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Scene;
import javafx.stage.Stage;
import javafx.scene.Parent;
import java.io.IOException;
public class MainApp extends Application {
@Override
public void start(Stage stage) throws IOException {
Parent root = FXMLLoader.load(getClass().getResource("/form.fxml"));
Scene scene = new Scene(root);
stage.setScene(scene);
stage.setTitle("Tron Form");
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
With the main application class in place, we can run the application to see our stunning JavaFX form with the mesmerizing Tron effect.
Bringing It All Together
In this tutorial, we've explored the process of building a JavaFX form with a captivating Tron effect. By leveraging JavaFX's capabilities, we've created a visually appealing user interface that showcases the potential of JavaFX in creating immersive experiences. The combination of FXML for layout definition, controller classes for logic and event handling, and the application class for the entry point, highlights the elegance and modularity of JavaFX development.
Now that you've gained insight into integrating mesmerizing visual effects into JavaFX applications, take your creativity to the next level by exploring further customization and effects to craft interfaces that truly captivate the user.
As you continue to enhance your JavaFX skills, be sure to stay updated with the latest advancements and best practices in Java application development. Always strive to expand your knowledge and stay ahead in the ever-evolving world of software development.
Start creating your own jaw-dropping JavaFX forms, incorporating mesmerizing visual effects, and leave a lasting impression on your users with immersive and captivating user interfaces.
So, what are you waiting for? Let your creativity flow and mesmerize the world with your JavaFX creations! Happy coding!
For more on building captivating JavaFX user interfaces, check out Oracle's official documentation and JavaFX in Action.
Checkout our other articles