Beat the Clock: Mastering Product Deliverables with Deadlines
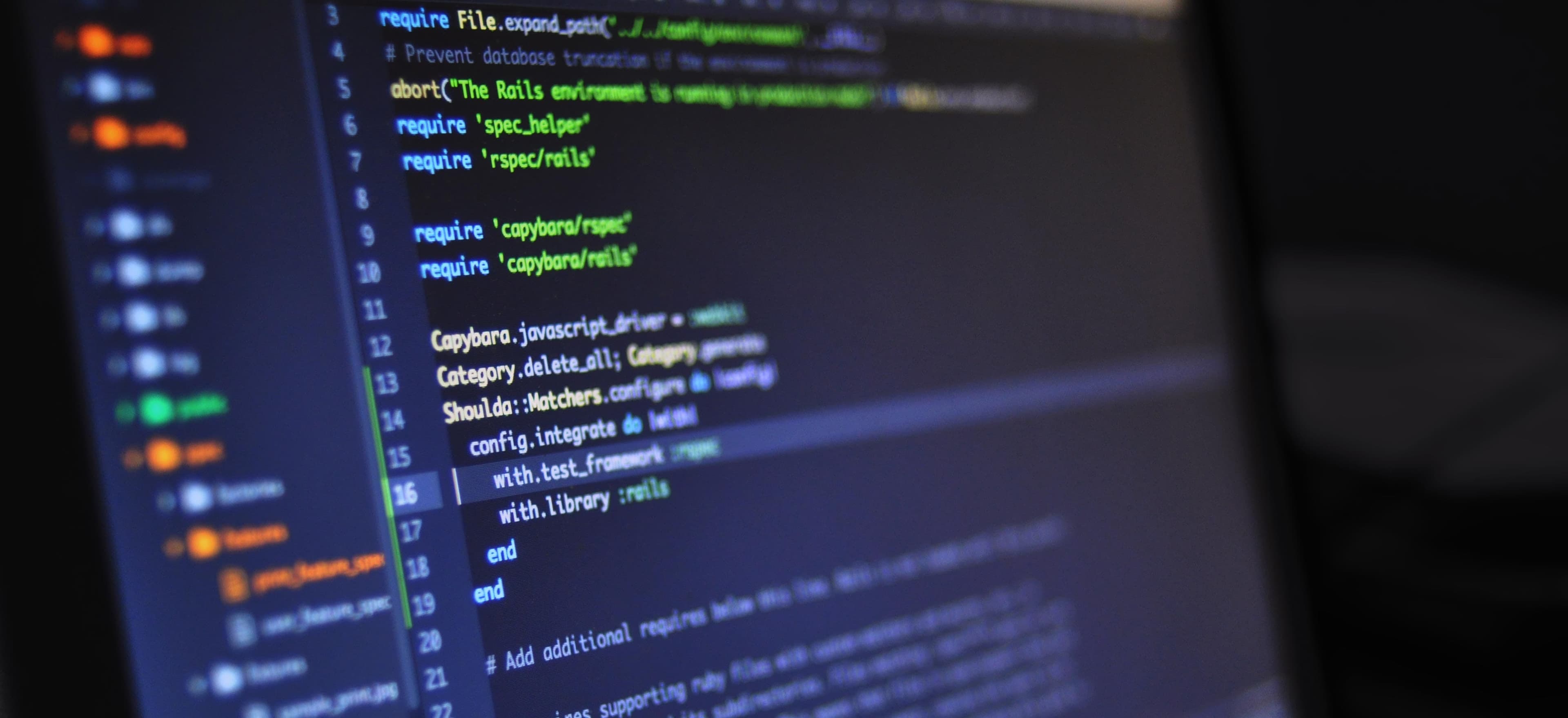
- Published on
How to Excel in Meeting Product Deliverables with Java
Product deliverables are crucial for the success of any software project. Meeting deadlines is essential, and when it comes to Java programming, there are several strategies to ensure timely and efficient delivery. In this post, we'll explore some tips and best practices for mastering product deliverables with Java.
Use of Agile Methodology for Project Management
Agile methodology is widely used in software development to manage projects efficiently. Agile promotes iterative development, collaboration, and quick responses to change. Java, with its vast ecosystem of frameworks and libraries, is well-suited for Agile development.
Example: Utilizing Jira for Agile Project Management
public class AgileProject {
public static void main(String[] args) {
JiraProjectManagement jira = new JiraProjectManagement();
jira.addUserStory("As a user, I want to be able to login to the system.");
jira.assignTaskToDeveloper("Implement user login functionality");
jira.setTaskPriority("High");
jira.startSprint("Sprint 1");
}
}
In this example, we see the use of Jira for managing user stories, assigning tasks, setting priorities, and starting sprints – all essential components of Agile project management.
Tapping into Continuous Integration and Deployment (CI/CD)
Continuous Integration and Deployment play a pivotal role in ensuring that the codebase remains stable and deployable at all times. Various tools like Jenkins, Travis CI, and CircleCI provide a seamless integration facility with Java projects, enabling automated builds, testing, and deployment.
Example: Implementing CI/CD with Jenkins
public class JenkinsPipeline {
public static void main(String[] args) {
JenkinsConfig config = new JenkinsConfig();
config.configurePipeline("MyJavaAppPipeline");
config.setTriggerOnCommit(true);
config.setBuildAndTestStages("build.gradle");
config.setDeployStage("deploy.sh");
config.enableEmailNotifications("dev-team@example.com");
}
}
Here, we demonstrate the setup of a Jenkins pipeline for a Java application, including triggering the build on commit, defining build and test stages, deploying using a shell script, and enabling email notifications.
Embracing Test-Driven Development (TDD)
Test-Driven Development (TDD) is a powerful approach to ensure high code quality and timely deliverables. By writing tests before the actual code, developers can validate functionality and catch issues early in the development cycle.
Example: Applying TDD with JUnit
public class StringManipulationTest {
@Test
public void testStringLength() {
StringManipulation sm = new StringManipulation();
assertEquals(5, sm.getStringLength("Hello"));
}
@Test
public void testStringConcatenation() {
StringManipulation sm = new StringManipulation();
assertEquals("Hello, World!", sm.concatenateStrings("Hello", "World!"));
}
}
In this example, we use JUnit to perform test-driven development for a StringManipulation
class, testing methods for string length and concatenation.
Leveraging Containerization with Docker
Docker simplifies the process of packaging, deploying, and running applications within containers. Java applications can benefit from Docker's platform-agnostic containers, ensuring consistent behavior across different environments.
Example: Containerizing a Java Application with Docker
FROM openjdk:11
ADD target/my-java-app.jar my-java-app.jar
ENTRYPOINT ["java", "-jar", "my-java-app.jar"]
In this Dockerfile, we define the container image for a Java application, specifying the base JDK image, adding the application JAR, and setting the entry point for execution.
Harnessing Parallel Processing with Java Streams
Java 8 introduced Streams, which provide a powerful way to process data in parallel. Leveraging parallel processing can significantly enhance the performance of data-intensive operations in Java applications.
Example: Parallel Processing with Java Streams
List<String> data = Arrays.asList("apple", "banana", "cherry", "date", "elderberry");
long count = data.parallelStream()
.filter(s -> s.startsWith("a"))
.count();
System.out.println("Count: " + count);
In this code snippet, we use Java Streams to perform parallel filtering and counting of elements in a list, showcasing the improved performance through parallel processing.
Bringing It All Together
Meeting product deliverables within deadlines is a challenging yet essential aspect of software development. By employing Agile methodology, continuous integration and deployment, test-driven development, containerization, and parallel processing, Java developers can ensure the timely and efficient delivery of high-quality software products.
Remember, mastering product deliverables is not just about coding—it's also about adopting the right methodologies and utilizing the best tools to streamline the development process.
Start incorporating these best practices into your Java development workflow, and witness the transformation in meeting product deliverables with precision and efficiency.
Now, it's your turn to excel in mastering product deliverables with Java!
Checkout our other articles