Unraveling JAAS with JSF: Secure Your WebApp Effortlessly
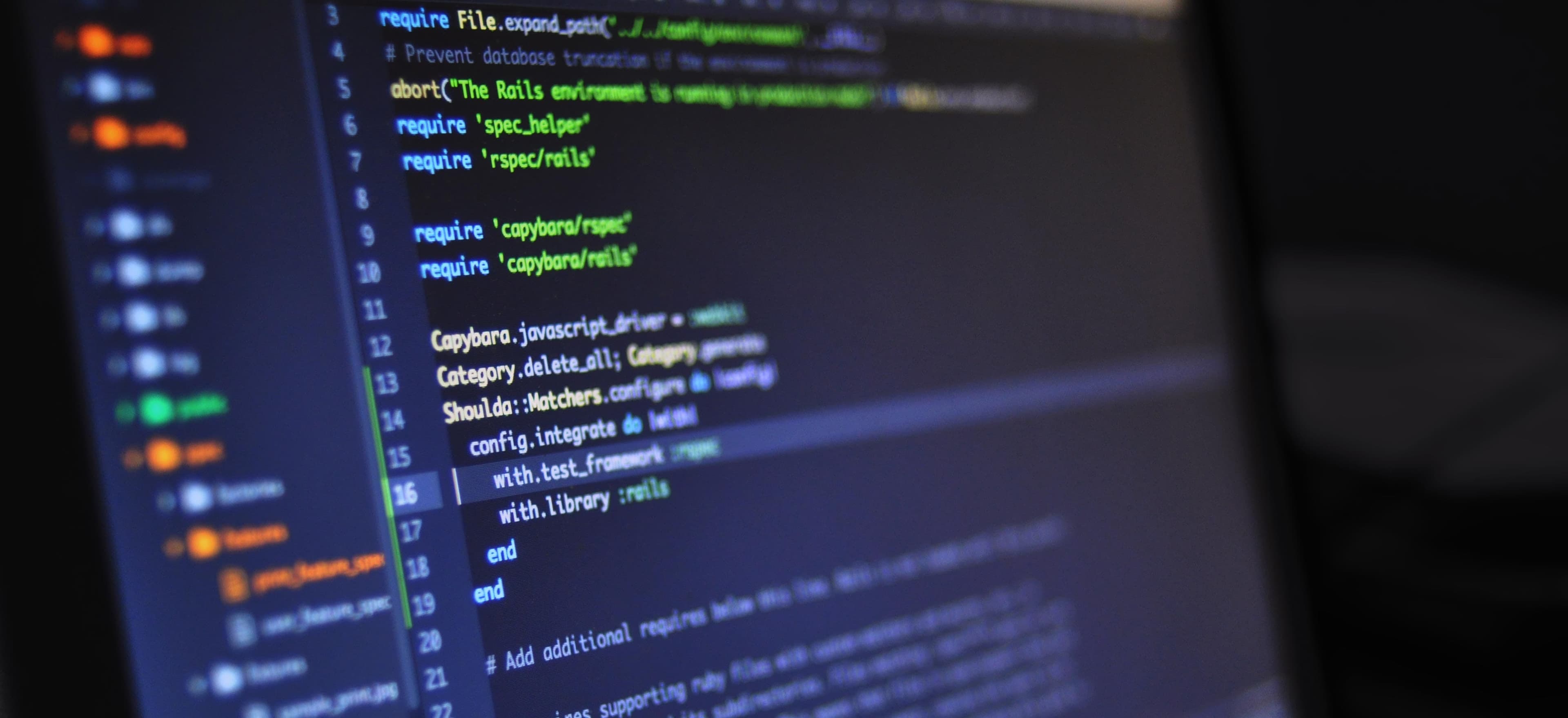
- Published on
Secure Your Java Web Application with JAAS and JSF
Securing a web application is a crucial aspect of software development. In the world of Java, Java Authentication and Authorization Service (JAAS) provides a way to implement user authentication and authorization within Java applications. When combined with JavaServer Faces (JSF), a robust and secure web application can be built effortlessly. In this blog post, we will explore the integration of JAAS with JSF to secure a Java web application.
What is JAAS?
Java Authentication and Authorization Service (JAAS) is a Java security framework that allows applications to securely interact with users, servers, and other services. JAAS provides a way to authenticate users and enforce access control upon them.
Integrating JAAS with JSF
To integrate JAAS with JSF, we need to follow a series of steps:
-
Configure JAAS Login Module: Create a Login Module to define how the authentication process should be handled. This can be achieved using JAAS configuration files.
-
Implement Custom JAAS Login Module: Develop a custom Login Module that specifies the logic for authenticating users. This can involve interactions with a database, LDAP, or any other data source.
-
Utilize JSF for User Interface: Build the web application using JavaServer Faces for the user interface. JSF provides components and libraries for building interactive and secure web applications.
-
Implement JAAS Authentication in JSF Managed Beans: Integrate JAAS authentication within JSF managed beans to enforce user authentication and authorization.
Configuring JAAS Login Module
Let's first define a JAAS configuration file (jaas.config
) for our web application:
SampleApplication {
com.example.custom.CustomLoginModule required;
};
In this configuration, SampleApplication
is the name of our application, and com.example.custom.CustomLoginModule
is the fully qualified name of the custom Login Module class. The required
keyword indicates that the Login Module is required for authentication.
Developing Custom Login Module
Now, let's implement the custom Login Module. This Login Module will authenticate users based on custom logic, such as checking credentials against a database.
package com.example.custom;
import javax.security.auth.callback.Callback;
import javax.security.auth.callback.CallbackHandler;
import javax.security.auth.callback.NameCallback;
import javax.security.auth.callback.PasswordCallback;
import javax.security.auth.callback.UnsupportedCallbackException;
import javax.security.auth.login.FailedLoginException;
import javax.security.auth.login.LoginException;
import javax.security.auth.spi.LoginModule;
public class CustomLoginModule implements LoginModule {
// Initialization, authentication, and other methods can be implemented here
}
In the CustomLoginModule
class, the initialize
, login
, and other methods will define the logic for authenticating users.
Building Web Application with JSF
With JAAS configured and the custom Login Module implemented, we can now focus on building the web application using JavaServer Faces. JSF provides a powerful framework for creating the user interface of the application.
Integrating JAAS Authentication in JSF Managed Beans
Here's an example of integrating JAAS authentication within a JSF managed bean to ensure that only authenticated users can access certain parts of the application:
package com.example.beans;
import javax.faces.bean.ManagedBean;
import javax.faces.context.FacesContext;
import javax.security.auth.Subject;
import javax.security.auth.login.LoginContext;
import javax.security.auth.login.LoginException;
@ManagedBean
public class LoginBean {
private String username;
private String password;
public String login() {
try {
LoginContext lc = new LoginContext("SampleApplication", new CustomCallbackHandler(username, password));
lc.login();
Subject subject = lc.getSubject();
// Code to navigate to the secure part of the application
return "secured/home?faces-redirect=true";
} catch (LoginException e) {
// Handle failed login
return "login?faces-redirect=true";
}
}
}
In this managed bean, the login
method initiates the JAAS login process. If successful, the user is redirected to the secure part of the application; otherwise, they are redirected back to the login page.
Summary
In this blog post, we have explored the integration of JAAS with JSF to secure a Java web application. By configuring JAAS Login Module, implementing a custom Login Module, building the web application with JSF, and integrating JAAS authentication within JSF managed beans, we can ensure a robust and secure web application.
Implementing JAAS with JSF provides a seamless way to incorporate authentication and authorization into Java web applications. By following these steps, developers can enhance the security of their web applications while providing a smooth user experience.
To learn more about JAAS, JSF, and Java web application security, check out the following resources:
- JAAS Documentation
- JSF Official Website
- OWASP Java Security
In conclusion, integrating JAAS with JSF empowers developers to secure their web applications effortlessly, ensuring the protection of sensitive data and maintaining user trust.
By incorporating JAAS and JSF, developers can deliver web applications that not only excel in functionality but also embody the highest standards of security. Cheers to developing secure Java web applications with confidence!