Solving the Mystery: Creating Your First Gradle Plugin
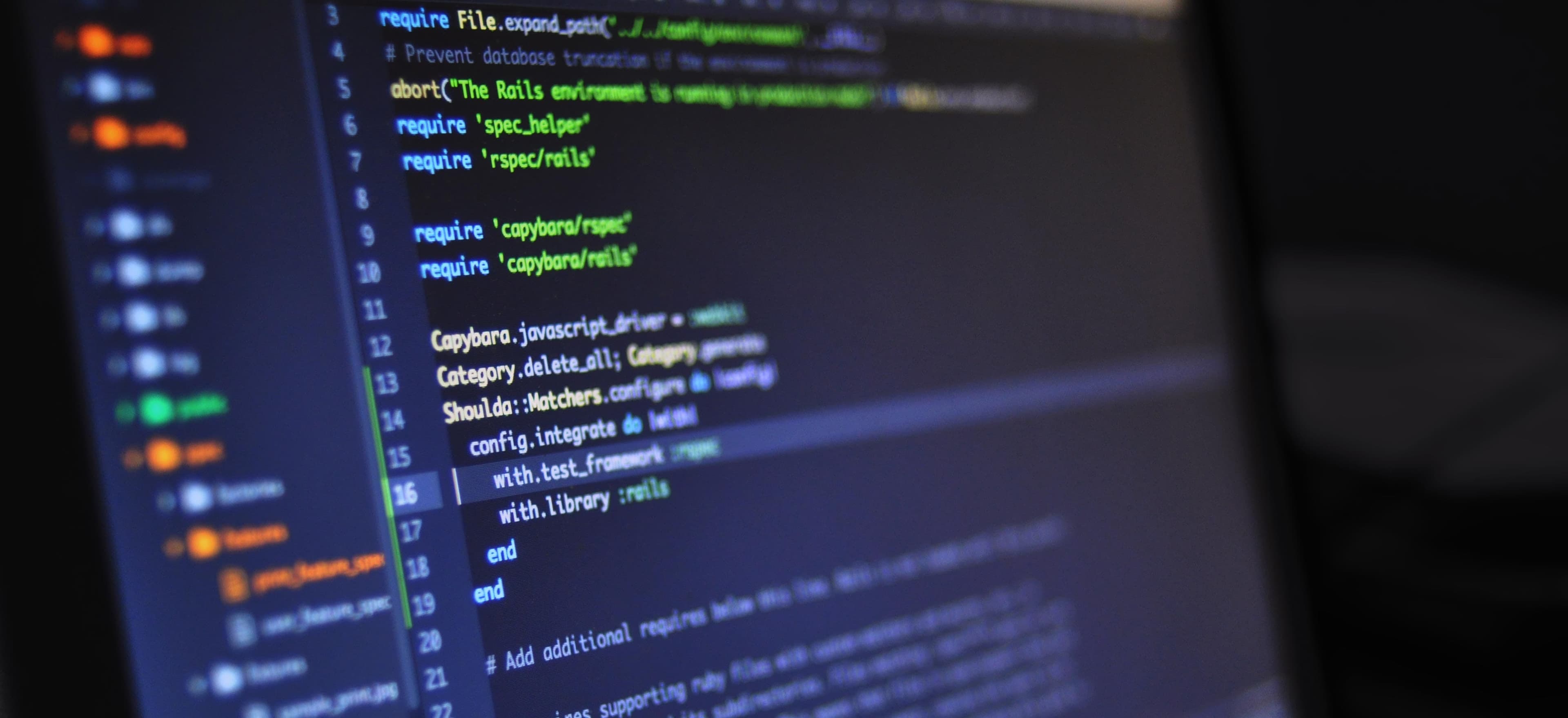
- Published on
Solving the Mystery: Creating Your First Gradle Plugin
So, you've been diligently working with Java and the Gradle build tool for some time now. You've mastered the art of creating build scripts, managing dependencies and tasks, and you're probably even an expert in customizing the build process using custom tasks and plugins. But now, you've come across a new challenge – creating your very own Gradle plugin.
Fear not! In this post, we'll unravel the mystery of creating your first Gradle plugin using Java. By the end, you'll be equipped with the knowledge and confidence to dive into the world of plugin development and extend Gradle's functionality to suit your specific needs.
Understanding Gradle Plugins
Before we embark on our journey to create a Gradle plugin, let's take a moment to understand what a Gradle plugin is and why you might want to create one.
In the context of Gradle, a plugin is a piece of code that adds specific functionality to your build. It may define new tasks, configure settings, or introduce entirely new concepts to the build process. Plugins are a powerful way to extend the capabilities of Gradle and encapsulate reusable build logic.
The Java Gradle Plugin
Java, being a highly modular and extensible language, provides a robust framework for creating Gradle plugins. By leveraging the Gradle API and the flexibility of Java, we can build plugins that seamlessly integrate with the Gradle build process and provide tailored solutions for various scenarios.
Getting Started
Now that we have a clear understanding of what a Gradle plugin is and why it's valuable, let's dive into the practical aspects of creating one. In this section, we'll go through the step-by-step process of building a simple Gradle plugin using Java.
Setting Up Your Development Environment
To begin, ensure that you have a Java development environment set up on your machine. You can use any Java IDE of your choice, such as IntelliJ IDEA, Eclipse, or NetBeans. Additionally, make sure you have Gradle installed on your system. If not, you can download and install Gradle following the official documentation.
Creating the Plugin Project
Start by creating a new Gradle project for your plugin. You can use the following Gradle build script to set up the project structure:
plugins {
id 'java-gradle-plugin'
}
group = 'com.yourcompany'
version = '1.0.0'
java {
toolchain {
languageVersion = JavaLanguageVersion.of(11)
}
}
repositories {
mavenCentral()
}
dependencies {
implementation localGroovy()
}
In this build script, we specify the use of the java-gradle-plugin
plugin, set the group and version for the project, configure the Java version, and define the dependencies, including the localGroovy()
dependency, which provides access to the Groovy runtime for writing Gradle scripts.
Creating the Plugin Class
Next, create a new Java class that will serve as the entry point for your plugin. This class should extend the org.gradle.api.Plugin
interface and implement the apply
method, where the logic of your plugin will reside:
package com.yourcompany.gradle;
import org.gradle.api.Plugin;
import org.gradle.api.Project;
public class MyGradlePlugin implements Plugin<Project> {
@Override
public void apply(Project project) {
// Plugin logic goes here
}
}
In this example, we've created a basic MyGradlePlugin
class that implements the Plugin
interface and applies the plugin logic within the apply
method. This is where you'll define the behavior of your plugin, such as adding custom tasks, configuring the project, or any other functionality you want to introduce to the build.
Applying the Plugin to a Project
Once you've defined the plugin class, you can apply it to a Gradle project. To do this, you need to build the plugin JAR and include it in the classpath of the target project.
To build the plugin JAR, add the following task to your plugin's build script:
task buildPlugin(type: Jar) {
from sourceSets.main.output
}
Now, you can run the buildPlugin
task to generate the plugin JAR file.
To use the plugin in another project, add the following line to the target project's build script:
buildscript {
repositories {
mavenLocal()
mavenCentral()
}
dependencies {
classpath 'com.yourcompany:your-plugin:1.0.0'
}
}
apply plugin: 'com.yourcompany.mygradleplugin'
Replace com.yourcompany:your-plugin:1.0.0
with the actual coordinates of your plugin JAR, and com.yourcompany.mygradleplugin
with the fully qualified name of your plugin class. Once applied, your plugin's logic will be executed as part of the target project's build process.
Testing the Plugin
Testing your plugin is crucial to ensuring its correctness and reliability. Gradle provides a robust testing framework that allows you to write unit tests for your plugin's logic.
Create a new test class for your plugin, following this example:
package com.yourcompany.gradle;
import org.gradle.testfixtures.ProjectBuilder;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class MyGradlePluginTest {
@Test
public void testPluginExecution() {
MyGradlePlugin myPlugin = new MyGradlePlugin();
Project project = ProjectBuilder.builder().build();
myPlugin.apply(project);
// Add assertions to validate the behavior of your plugin
}
}
In this test class, we create a new instance of our plugin, build a test Gradle project using ProjectBuilder
, apply the plugin to the project, and then add assertions to validate the expected behavior of the plugin.
Running the tests will ensure that your plugin behaves as intended and help catch any potential issues early in the development process.
Key Takeaways
In this post, we've embarked on a journey to demystify the process of creating a Gradle plugin using Java. Armed with this newfound knowledge, you're now ready to harness the power of Gradle plugin development and build customized solutions tailored to your specific use cases.
Creating a Gradle plugin in Java opens up a world of possibilities for extending Gradle's functionality, encapsulating reusable build logic, and simplifying complex build processes. With the foundational understanding and practical insights gained from this guide, you're well-equipped to take your Gradle skills to the next level and conquer new frontiers in build automation.
So, go forth and unleash the full potential of Gradle plugin development with Java, and may your build processes be ever efficient, elegant, and tailored to perfection! Happy coding!
Remember, the journey doesn't end here. Keep exploring, building, and refining your skills in Gradle plugin development to become a true master of build orchestration.
Happy coding, and happy Gradle plugin crafting!
This post was brought to you by YourCompany, empowering developers to revolutionize their build processes with custom Gradle plugins.
Remember, the journey doesn't end here. Keep exploring, building, and refining your skills in Gradle plugin development to become a true master of build orchestration.
Happy coding, and happy Gradle plugin crafting!