Mastering Method Overload: Avoiding Too Many Parameters!
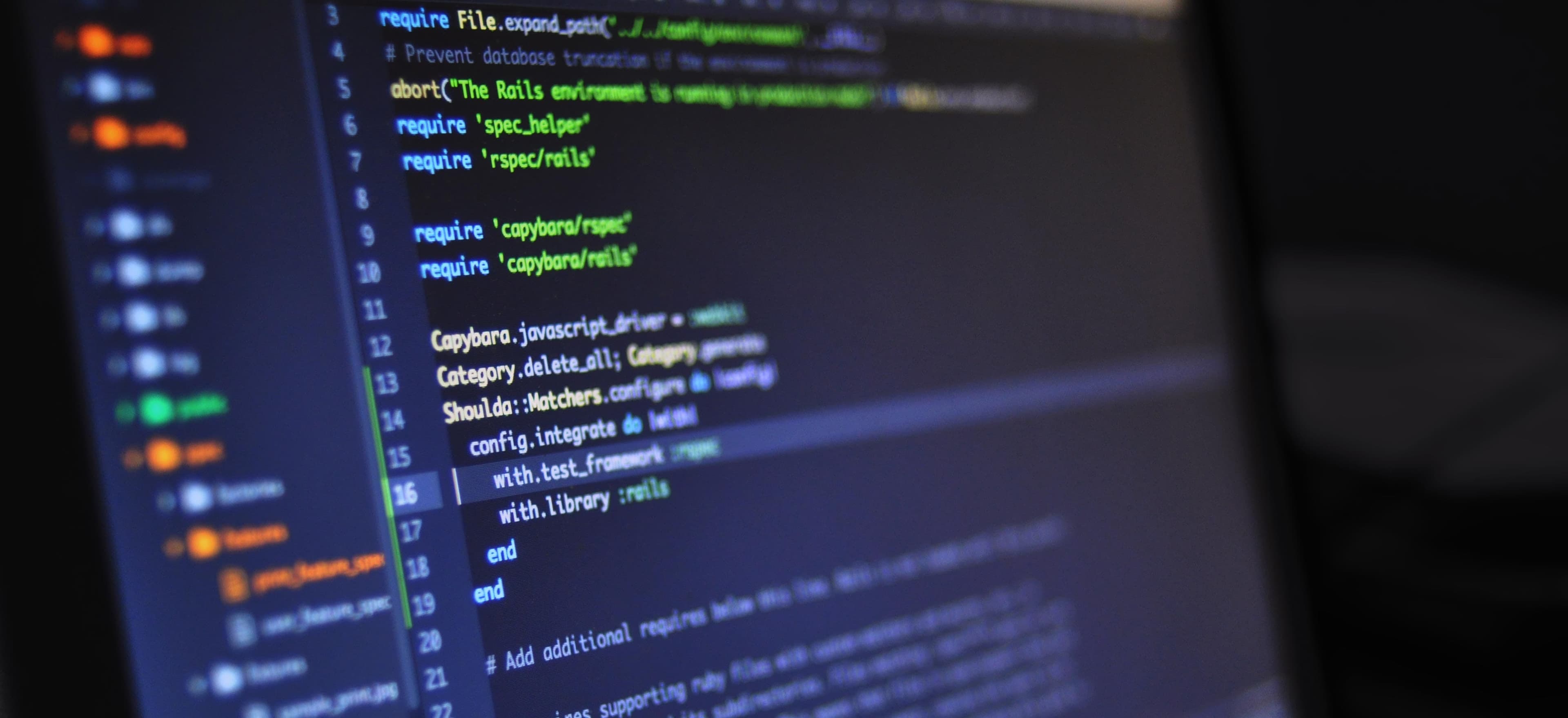
- Published on
Mastering Method Overload: Avoiding Too Many Parameters
When it comes to writing clean and maintainable code in Java, method overload is a powerful tool. It allows us to define multiple methods with the same name but different parameters, enabling us to create cleaner and more readable code. However, overusing method overload can lead to a cluttered and confusing codebase. In this article, we will explore how to effectively use method overload in Java, and more importantly, how to avoid the pitfalls of having too many parameters.
The Power of Method Overload
Method overload, also known as method overloading, is a feature that allows a class to have two or more methods having the same name, if their parameter lists are different. This enables us to create multiple methods with the same name but different behavior based on the parameters they accept.
Here's an example to illustrate the concept:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public double add(double a, double b) {
return a + b;
}
}
In this example, we have two add
methods in the Calculator
class. One accepts two integers, and the other accepts two doubles. This allows us to perform addition for both integers and doubles using the same method name, making the code more intuitive and readable.
The Pitfalls of Too Many Parameters
While method overload can improve code readability and maintainability, excessive use of method overload with too many parameters can lead to confusion and maintenance issues. It's essential to strike a balance and know when to use method overload judiciously.
Consider the following example:
public class Shape {
public void drawRectangle(int x, int y, int width, int height) {
// ...
}
public void drawCircle(int x, int y, int radius) {
// ...
}
public void drawOval(int x, int y, int radius1, int radius2) {
// ...
}
}
In this example, we have three methods with similar parameters. As the number of parameters increases, it becomes more challenging to remember the order and meaning of each parameter. This can lead to errors and confusion, especially when maintaining and modifying the code.
Strategies for Effective Method Overload
1. Use When Behavior Is Similar
Method overload is most effective when the behavior of the overloaded methods is similar. It should provide a variation based on the parameters without significantly changing the core functionality. This ensures that each method is intuitive and easy to understand.
2. Limit the Number of Parameters
As a best practice, limit the number of parameters for each method. If a method requires more than three or four parameters, consider refactoring it to accept an object that encapsulates the parameter values. This not only improves readability but also makes the code more maintainable.
3. Consider Default Parameters in Java 8 and Later
Starting from Java 8, default parameters are supported using the @Deprecated
annotation. This allows you to provide default values for method parameters, reducing the need for method overload in some cases. However, use this feature judiciously, as excessive use of default parameters can also lead to confusion.
4. Embrace Builder Pattern for Complex Parameters
When dealing with complex parameter combinations, consider using the Builder pattern to construct objects with varying parameters. This helps in simplifying the creation of objects with many optional parameters and can eliminate the need for method overload in certain scenarios.
Best Practices for Method Overload
Now that we've explored the potential pitfalls and strategies for effective method overload, let's summarize some best practices to keep in mind when using method overload in Java:
-
Clarity and Readability: Always prioritize code readability. If method overload improves readability and makes the code more intuitive, it's a good use case. Otherwise, consider other alternatives such as using a different method name.
-
Limited Parameters: Limit the number of parameters for each method, aiming for simplicity and ease of use. When the number of parameters starts to feel overwhelming, consider refactoring using objects or the Builder pattern.
-
Similar Behavior: Ensure that the overloaded methods have similar behavior, providing variations based on the parameters. This helps maintain consistency and makes the codebase easier to understand.
My Closing Thoughts on the Matter
Method overload is a powerful feature in Java that, when used effectively, can lead to cleaner and more intuitive code. However, it's crucial to be mindful of the potential drawbacks, particularly when it comes to managing a large number of parameters. By following best practices, such as limiting the number of parameters and ensuring clarity and readability, developers can harness the benefits of method overload while avoiding the associated pitfalls.
In conclusion, understanding when and how to use method overload is essential to maintain a clean and maintainable codebase. By applying the strategies and best practices outlined in this article, developers can master method overload and write more efficient and maintainable Java code.
Remember, mastering method overload is not just about creating multiple methods with the same name—it's about creating cleaner, more intuitive code that stands the test of time.