Decoding the Mystery: Why Integer.valueOf(127) == true?
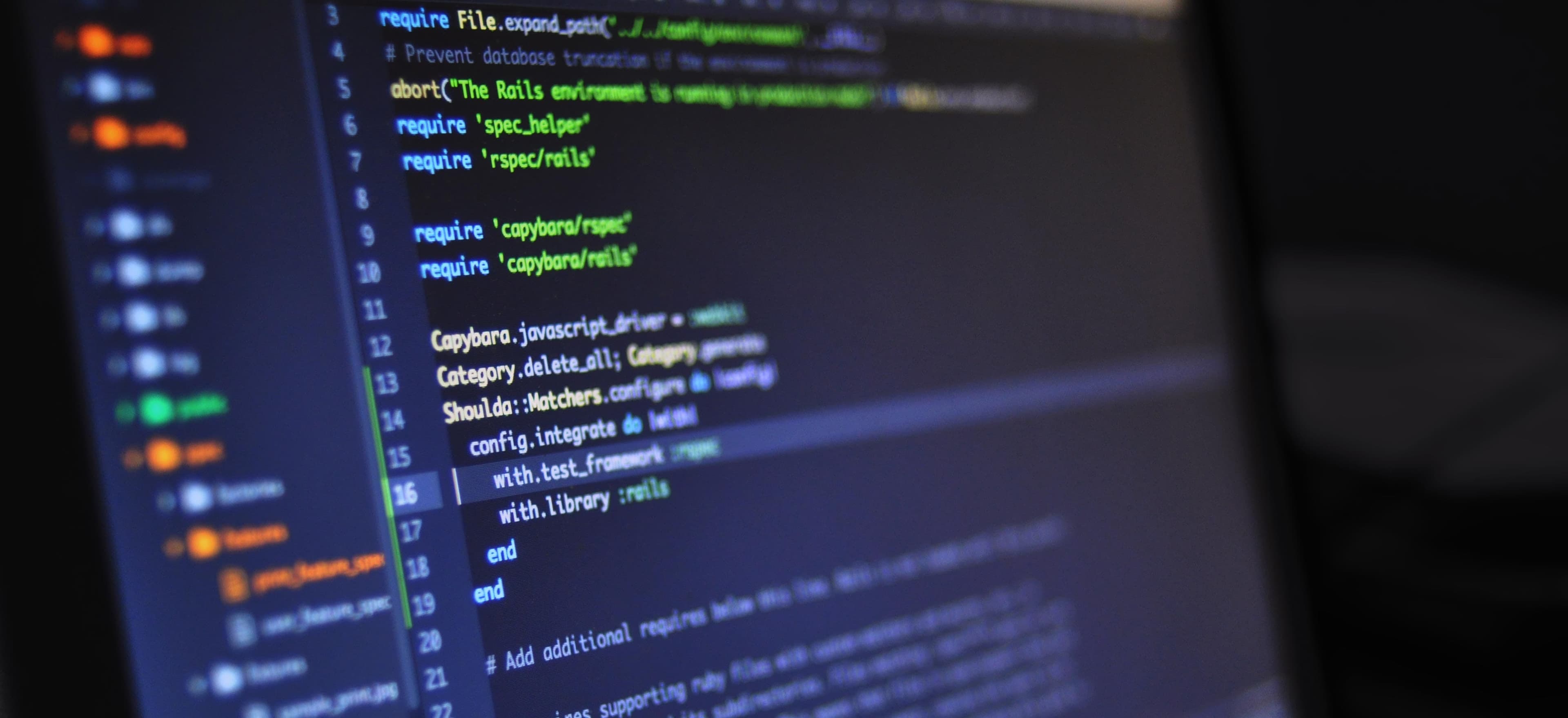
- Published on
Decoding the Mystery: Why Integer.valueOf(127) == Integer.valueOf(127)
Returns true
in Java?
In Java, we often come across puzzling scenarios that may seem counterintuitive at first glance. One such instance revolves around the comparison of Integer objects using the ==
operator. In this blog post, we delve into the intricacies of autoboxing and caching mechanisms to unravel the mystery of why Integer.valueOf(127) == Integer.valueOf(127)
yields true
, while the same comparison with a value beyond a certain range behaves differently.
Autoboxing in Java
In Java, autoboxing is the automatic conversion of primitive types to their corresponding wrapper classes. For example, when an int
is assigned to an Integer
, autoboxing comes into play.
Consider the following code snippet:
Integer a = 10; // Autoboxing: int to Integer
Here, the compiler automatically converts the primitive int
value 10
to its corresponding Integer
object using autoboxing.
Integer.valueOf() Method
The Integer.valueOf(int)
method returns an Integer
instance representing the specified int
value. It internally utilizes a caching mechanism by maintaining a cache of frequently used Integer
objects within the range of -128
to 127
. Thus, when called with integers within this range, it returns the cached Integer
instance, leading to unexpected comparison results.
Let's examine the following code snippet:
Integer a = 127;
Integer b = 127;
System.out.println(a == b); // Output: true
In this case, both a
and b
refer to the same Integer
object due to caching, hence the ==
comparison returns true
.
The Mystery Unveiled
Now, let's address the mystery concerning Integer.valueOf(127) == Integer.valueOf(127)
yielding true
. The reason lies in the caching strategy employed by Integer.valueOf()
for values within the range of -128
to 127
.
When Integer.valueOf(127)
is called, it returns a reference to the cached instance representing the value 127
. Subsequently, when Integer.valueOf(127)
is called again, the same cached instance is retrieved, leading to the comparison yielding true
.
However, if we extend the range beyond the cache boundaries, say to 128
, the caching mechanism does not come into play, resulting in the creation of distinct Integer
instances for the same value. Consequently, the comparison Integer.valueOf(128) == Integer.valueOf(128)
will return false
.
Code Demonstration
Let's illustrate the behavior with a code example:
Integer a = 127;
Integer b = 127;
System.out.println(a == b); // Output: true
Integer c = 128;
Integer d = 128;
System.out.println(c == d); // Output: false
In the above code snippet, a
and b
share the same cached Integer
instance due to the caching mechanism, leading to the ==
comparison resulting in true
. Conversely, c
and d
do not fall within the cached range, resulting in distinct Integer
instances and the comparison returning false
.
Caveats and Best Practices
While the caching behavior may be optimized for memory efficiency and certain use cases, it is crucial to exercise caution when using the ==
operator for comparing Integer
objects. For meaningful comparisons, employing the equals()
method is recommended to ensure the comparison is based on the actual numerical values rather than object references.
The Closing Argument
In Java, the intricacies of autoboxing, caching mechanisms, and object comparison can lead to unexpected results, as demonstrated by the puzzling behavior of Integer.valueOf(127) == Integer.valueOf(127)
yielding true
. Understanding the underlying mechanisms and the impact of caching on object creation and comparison is vital for writing robust and predictable Java code.
By unraveling the mystery behind the behavior of Integer.valueOf()
and the ==
operator, we have shed light on a fascinating aspect of Java's internal workings, empowering developers to write more informed and reliable code.
In conclusion, while Java’s caching mechanism provides optimizations, it’s essential to grasp its implications for object comparison and exercise caution when relying on the ==
operator for comparing Integer
objects.
Additional Resources
To delve deeper into the nuances of autoboxing, caching, and object comparison in Java, consider exploring the following resources:
- Java Autoboxing and Unboxing
- Understanding Integer Caching in Java
- Effective Java by Joshua Bloch
Stay curious, keep unraveling the mysteries, and happy coding!