Troubleshooting SecurityContextHolder in Spring Security
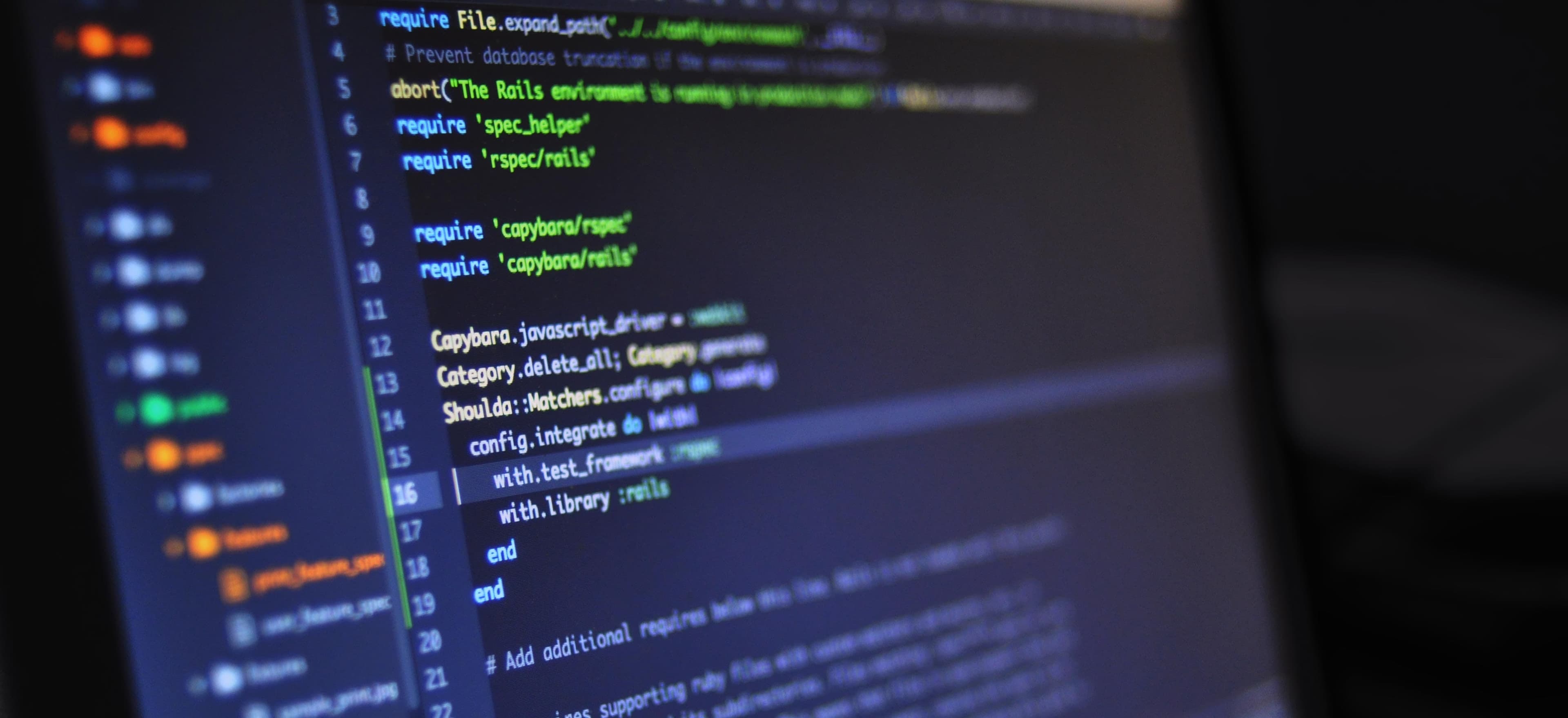
- Published on
Troubleshooting SecurityContextHolder in Spring Security
Spring Security is a powerful framework that provides comprehensive security solutions for Java applications. One of the key components of Spring Security is the SecurityContextHolder
, a crucial abstraction that holds the security context of the current thread. While SecurityContextHolder
simplifies security management, it can sometimes pose challenges, especially when dealing with complex security configurations. In this article, we will explore common issues related to SecurityContextHolder
and how to troubleshoot them effectively.
Understanding SecurityContextHolder
Before delving into troubleshooting, let's briefly discuss the role of SecurityContextHolder
in Spring Security. The SecurityContextHolder
is essentially a holder for the SecurityContext
specific to the current execution thread. The SecurityContext
contains the details of the currently authenticated principal (user) and related security information.
In a typical Spring Security-enabled application, SecurityContextHolder
is utilized to access the SecurityContext
and retrieve the information about the current user, authentication details, and authorities.
Common Issues with SecurityContextHolder
1. Null SecurityContext
One of the most common issues encountered with SecurityContextHolder
is the presence of a null SecurityContext
. This can occur when attempting to access the SecurityContext
in a thread where it has not been properly initialized or populated. It may lead to NullPointerExceptions or unexpected behavior in the application.
2. Incorrect SecurityContext Population
Another issue arises when the SecurityContext
is not populated with the expected authentication details. This can happen due to misconfigurations in the authentication process, improper usage of authentication mechanisms, or manual manipulation of the SecurityContext
without ensuring consistency.
3. Unexpected User Information
At times, developers may encounter situations where accessing user information from the SecurityContextHolder
yields unexpected or incorrect results. This could be due to incorrect retrieval methods, improper propagation of the security context, or inconsistencies in the authentication flow.
Troubleshooting SecurityContextHolder Issues
Now that we understand the common issues related to SecurityContextHolder
, let's delve into troubleshooting strategies to address these issues effectively.
1. Verify Correct Configuration of Security Components
Start by ensuring that the security components in your application, such as AuthenticationManager
, UserDetailsService
, and SecurityConfigurer
, are configured correctly. Misconfigurations in these components can lead to issues with the SecurityContextHolder
.
Example:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService).passwordEncoder(passwordEncoder());
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
In this example, we ensure that the UserDetailsService
and password encoder are properly configured to provide correct authentication details to populate the SecurityContext
.
2. Check for Proper Security Context Propagation
Verify that the security context is properly propagated across different threads and components where it is required. This is crucial, especially in asynchronous or multi-threaded scenarios, to ensure that the SecurityContextHolder
is consistently populated with the correct security context.
3. Debug Null SecurityContext Issues
If you encounter null SecurityContext
issues, consider debugging the application to trace the flow of the security context initialization and population. This can involve inspecting the configuration, security filter chain, and authentication process to identify potential causes of the null SecurityContext
.
4. Review Concurrent Thread Safety
Ensure that the usage of SecurityContextHolder
is thread-safe, particularly in scenarios where multiple threads may access or modify the security context concurrently. Use appropriate synchronization mechanisms or consider delegating security context management to Spring-managed components.
5. Logging and Error Handling
Implement detailed logging and error handling to capture unexpected behavior related to SecurityContextHolder
. Logging the interactions with the SecurityContextHolder
and related components can provide valuable insights into the root causes of issues.
The Bottom Line
In conclusion, the SecurityContextHolder
in Spring Security plays a pivotal role in managing the security context of Java applications. While it simplifies security management, issues related to SecurityContextHolder
can arise due to misconfigurations, improper propagation, and inconsistent usage. By understanding the common issues and employing effective troubleshooting strategies, developers can ensure the reliable and secure functioning of SecurityContextHolder
in their Spring Security-enabled applications.
For further understanding, you can refer to the official Spring Security documentation and Baeldung's Spring Security guides.