Java 8 Lambdas: Sorting Performance Pitfalls
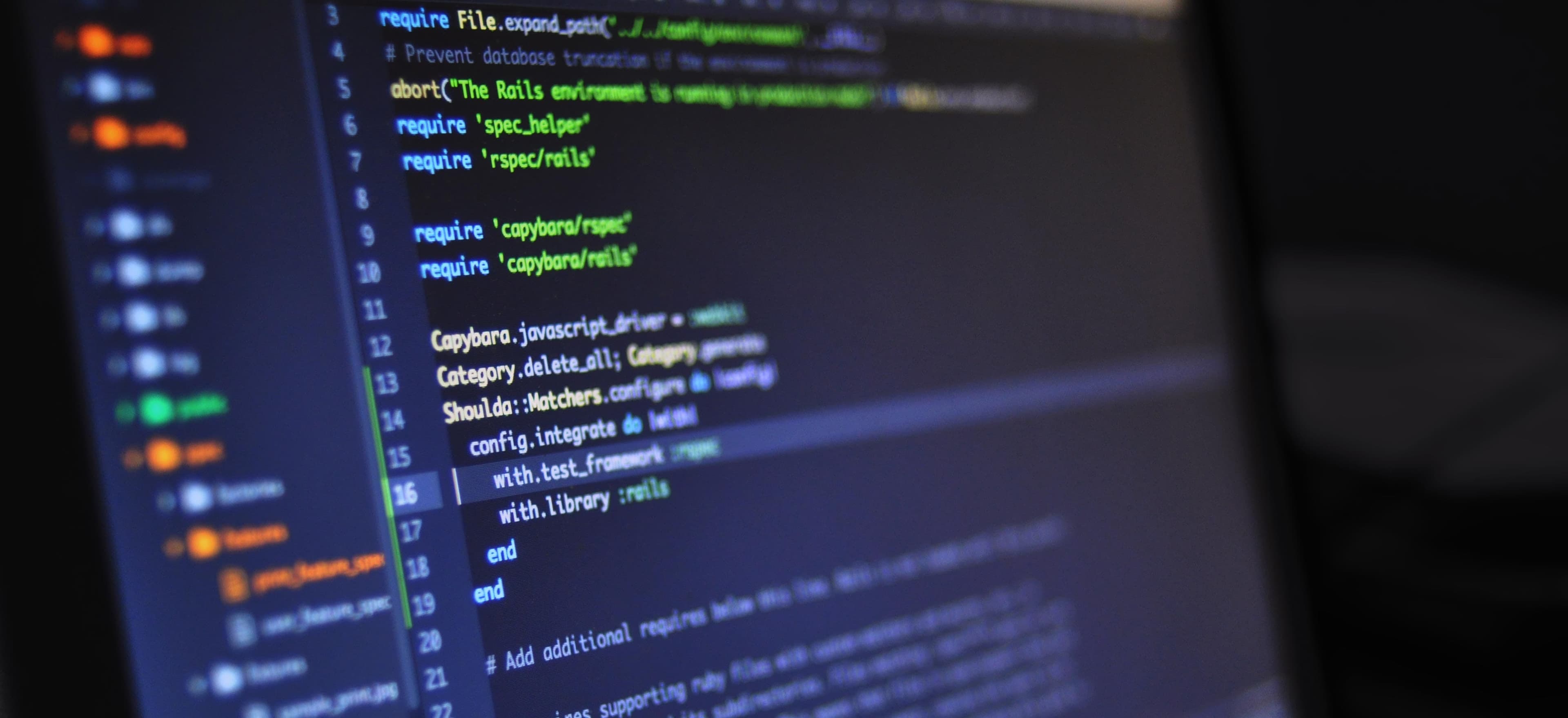
- Published on
Java 8 Lambdas: Sorting Performance Pitfalls
Java 8 introduced the concept of lambdas, which brought a significant change in the way Java developers write code. Lambdas allow for concise, functional-style programming, making code more readable and expressive. However, when it comes to sorting collections using lambdas, there are performance pitfalls that developers should be aware of. In this blog post, we will explore these pitfalls and discuss best practices for sorting collections using lambdas in Java.
Understanding Java 8 Lambdas
Lambdas in Java 8 provide a way to represent a piece of code as an object. They are particularly useful for writing concise and readable code, especially when dealing with functional interfaces, which have only one abstract method. In the context of sorting, lambdas allow developers to define custom comparison logic without the need to create separate comparator classes.
The Pitfalls of Sorting with Lambdas
While lambdas offer a more streamlined approach to sorting, there are performance implications that developers should consider, especially when working with large collections.
One common pitfall is the overhead of creating and using lambda expressions compared to traditional comparator-based sorting. When using lambdas for sorting, each invocation of the lambda expression results in the creation of a function object. This incurs additional memory and runtime overhead, which can impact the performance, particularly in scenarios where sorting is performed frequently or on large datasets.
Another pitfall is related to the lack of type information when using lambdas. In the context of sorting, this can lead to potential runtime errors if the types of objects being sorted are not handled carefully within the lambda expression.
Best Practices for Sorting with Lambdas
Use Comparator.comparing for Simple Comparisons
When performing simple comparisons, it's best to use the Comparator.comparing
method, which allows for a more efficient and readable approach to sorting using lambdas. The Comparator.comparing
method takes a lambda expression that extracts the key to be compared, resulting in more concise code without sacrificing performance.
List<Person> people = //...
people.sort(Comparator.comparing(Person::getLastName));
Be Mindful of Autoboxing Overhead
When working with primitive types and autoboxing, there can be significant overhead associated with using lambdas for sorting. It's important to be mindful of this overhead, especially when dealing with large collections of primitive types. In such cases, using traditional comparator-based sorting may be more efficient.
Consider Caching Comparator Instances
In scenarios where the same comparison logic is reused across multiple sorting operations, it's beneficial to cache comparator instances to avoid the overhead of creating new function objects repetitively. This approach can help improve performance, especially when sorting is performed frequently with the same comparison logic.
Comparator<Person> lastNameComparator = Comparator.comparing(Person::getLastName);
people.sort(lastNameComparator);
// Use lastNameComparator for other sorting operations
In Conclusion, Here is What Matters
Java 8 lambdas have undoubtedly improved the readability and expressiveness of Java code, but they also introduce performance considerations when used for sorting collections. By understanding the pitfalls and adopting best practices, developers can leverage the benefits of lambdas while mitigating the associated performance overhead.
In summary, when it comes to sorting with lambdas in Java:
- Use
Comparator.comparing
for simple comparisons - Be mindful of autoboxing overhead for primitive types
- Consider caching comparator instances for reuse
By following these best practices, developers can write efficient and expressive sorting logic using lambdas in Java. Understanding the trade-offs and making informed decisions based on the specific requirements of the application is crucial for leveraging the power of lambdas while maintaining optimal performance.
For further reading on lambdas and sorting in Java, refer to the official Java documentation and other resources available on the topic.
Remember, while lambdas offer conciseness and expressiveness, it's important to weigh the trade-offs and make informed decisions based on the specific context of your application.
Happy coding!