Boosting Business: Solving Slow Testing With Frameworks
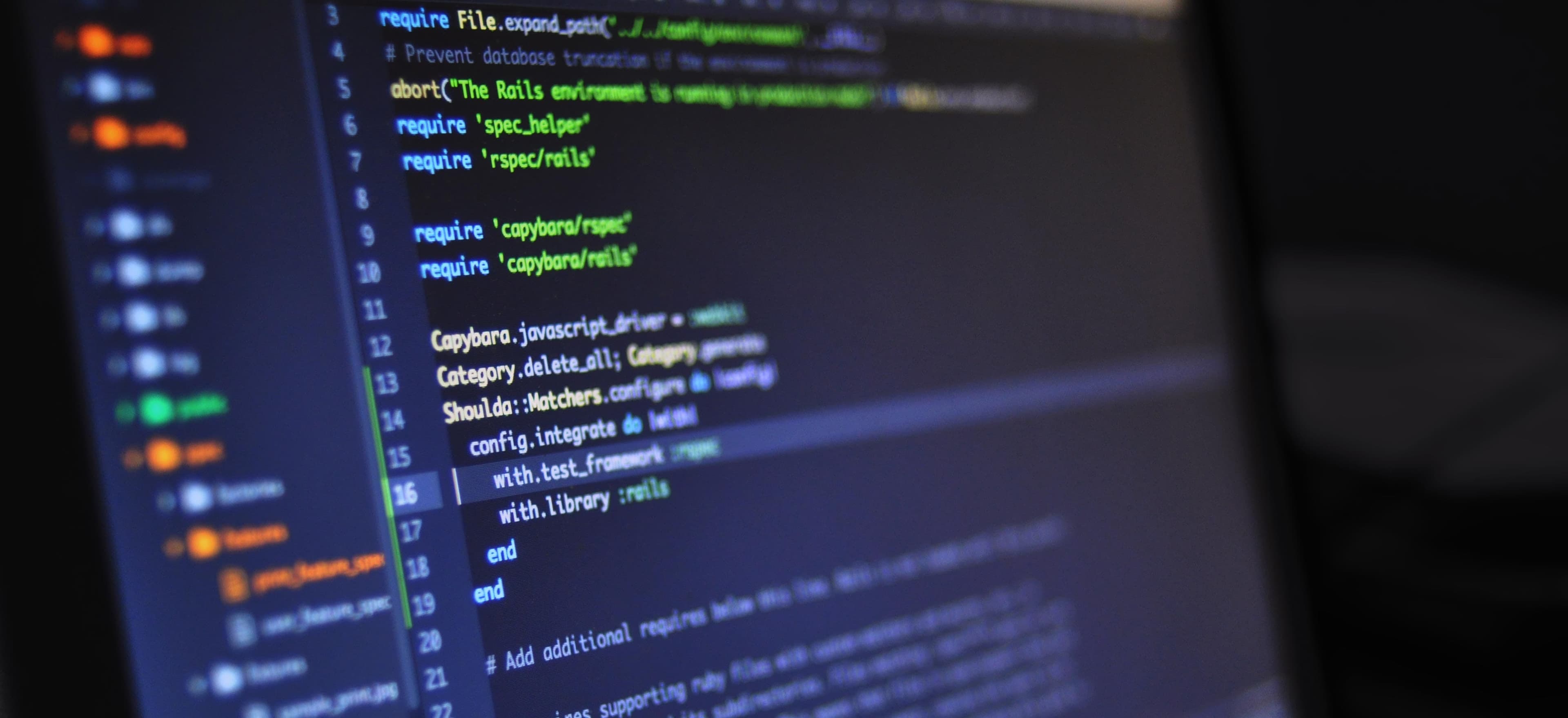
- Published on
Boosting Business: Solving Slow Testing With Frameworks
In the fast-paced world of software development, speed and efficiency are essential. One common challenge that developers face is slow testing, which can significantly hinder the development process and impact business outcomes. In this blog post, we will explore how leveraging testing frameworks can help to solve the problem of slow testing, ultimately boosting business productivity.
Understanding the Challenge
Slow testing can be attributed to a variety of factors including complex test setups, repetitive test routines, and inefficient test frameworks. As the codebase grows, the time taken for running tests can increase exponentially, negatively impacting the development cycle. This can lead to delayed releases, reduced productivity, and ultimately, a negative impact on the business.
The Role of Testing Frameworks
Testing frameworks play a crucial role in streamlining the testing process by providing a structured approach to writing and executing tests. They offer a wide range of features such as test organization, assertion libraries, and setup/teardown functionalities. By utilizing testing frameworks effectively, developers can write efficient tests that run faster and provide valuable feedback in a shorter time frame.
Choosing the Right Framework
When it comes to selecting a testing framework, the choice should be based on the specific needs of the project, the programming language being used, and the testing requirements. In the Java ecosystem, several testing frameworks are available, each with its own strengths and use cases. Two popular choices are JUnit and TestNG.
JUnit
JUnit is a widely-used testing framework for Java, known for its simplicity and ease of use. It provides annotations for test methods, assertions for verification, and lifecycle methods for setup and teardown. Let's take a look at a simple example of a JUnit test:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class MyUnitTest {
@Test
public void testAddition() {
int result = Calculator.add(3, 5);
assertEquals(8, result);
}
}
In this example, we have a basic test method that verifies the functionality of an add
method in a Calculator
class. The assertEquals
method is used to assert that the expected result matches the actual result.
TestNG
TestNG is another powerful testing framework for Java, offering additional features such as parameterized tests, dependency management, and powerful execution models. Here's an example of a TestNG test:
import org.testng.annotations.Test;
import static org.testng.Assert.assertEquals;
public class MyTestNGTest {
@Test
public void testSubtraction() {
int result = Calculator.subtract(8, 3);
assertEquals(result, 5);
}
}
In this example, we have a similar test that uses TestNG's assertion method assertEquals
to verify the functionality of a subtract
method.
Improving Test Performance
Now that we have an understanding of how testing frameworks work, let's explore how they can help in improving test performance.
Parallel Test Execution
One of the key features provided by testing frameworks is the ability to execute tests in parallel. This can significantly reduce the overall test execution time, especially when dealing with a large test suite. By running tests concurrently, developers can obtain faster feedback on the quality of their code, accelerating the development process.
Test Isolation and Mocking
Testing frameworks enable effective isolation of tests, ensuring that each test runs independently without interference from other tests. Additionally, frameworks like Mockito can be integrated to create mock objects for dependencies, eliminating the need for heavy setup operations and enhancing test speed.
Test Data Management
Efficient test data management is crucial for optimizing test performance. Testing frameworks offer mechanisms for data-driven testing, allowing multiple sets of input data to be used across a single test, ultimately reducing the need for redundant test code and accelerating the testing process.
Integrating Continuous Integration
To further enhance testing efficiency, integrating testing frameworks with a robust continuous integration (CI) system is essential. CI platforms such as Jenkins, Travis CI, or CircleCI can automate the execution of tests, providing rapid feedback on code changes and ensuring that quality is maintained throughout the development lifecycle. This integration plays a vital role in supporting a culture of continuous improvement and rapid delivery within the organization.
Closing Remarks
Slow testing can serve as a significant bottleneck in the software development process, impacting the overall productivity and success of a business. By leveraging testing frameworks such as JUnit and TestNG, along with best practices such as parallel test execution, test isolation, and effective data management, developers can accelerate the testing process and boost business outcomes. Additionally, integrating testing frameworks with a robust CI system can further enhance the efficiency and reliability of the testing process, contributing to the overall success of the business.
In conclusion, testing frameworks are invaluable tools for addressing the challenge of slow testing, providing a pathway to more efficient and productive development practices.
By implementing these strategies, businesses can ensure that their software development processes remain agile, impactful, and conducive to growth and success.
To learn more about implementing and optimizing testing frameworks in Java, check out this in-depth guide from JavaWorld.
For a comprehensive understanding of integrating testing frameworks with continuous integration systems, refer to this informative article.