Mastering Domain Logic: Avoid Common Mistakes in Design
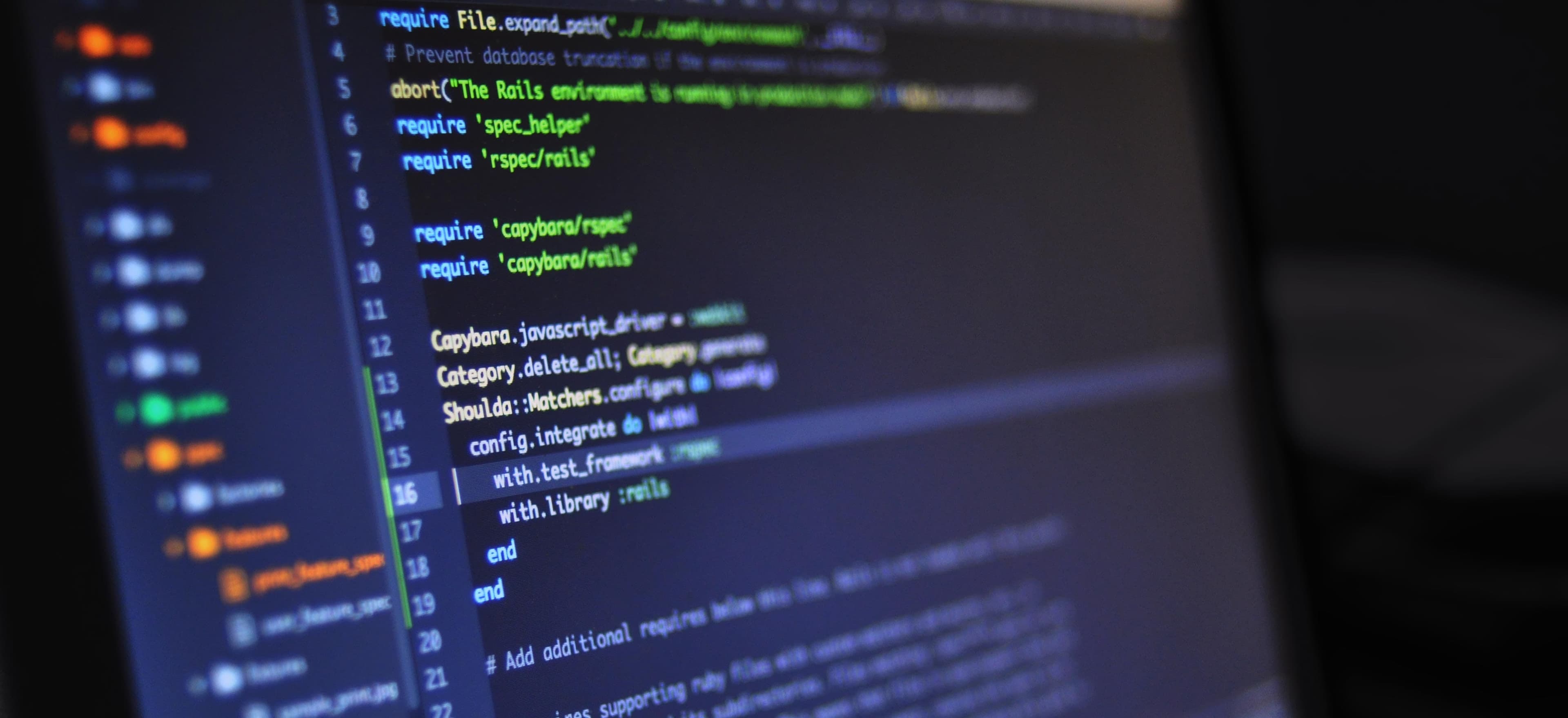
- Published on
Mastering Domain Logic: Avoid Common Mistakes in Design
When it comes to building software applications, especially in Java, mastering domain logic is fundamental for creating a well-structured, maintainable, and scalable system. Domain logic represents the core business rules and behaviors of an application, and it's crucial to get it right from the outset to avoid common pitfalls that can lead to technical debt and inefficiencies in the long run.
Understanding Domain Logic
In the context of software development, domain logic refers to the specific rules, validations, and workflows that govern a particular business domain. This includes the way data is processed, transformed, and validated within the application. In Java, domain logic is typically encapsulated within domain objects, which are the building blocks of the domain model.
Common Mistakes in Domain Logic Design
Anemic Domain Model
One of the common mistakes in domain logic design is creating an anemic domain model, where domain objects are reduced to simple data containers with little or no behavior. This often leads to bloated service layers and procedural code, violating the principles of object-oriented design.
// Anemic Domain Model Anti-pattern
public class Order {
private Long id;
private List<Item> items;
// Getters and setters
}
public class OrderService {
public void processOrder(Order order) {
// Perform business logic using procedural code
}
}
Violating Single Responsibility Principle (SRP)
Another pitfall is violating the Single Responsibility Principle by mixing domain logic with infrastructure or presentation concerns. Domain objects should focus solely on encapsulating domain logic without being coupled to persistence, validation, or presentation mechanisms.
Inadequate Validation
Inadequate or improper validation within the domain logic can lead to inconsistent or invalid state transitions, compromising the integrity of the application's data. It's important to enforce business rules and constraints at the domain level to ensure data correctness.
Procedural Code in Domain Methods
Embedding procedural code within domain methods instead of leveraging cohesive behavior can hinder maintainability and testability. Domain methods should embody the business operations and behaviors specific to the domain concept they represent.
public class Invoice {
public void calculateTotal() {
// Procedural calculation logic
}
}
Best Practices for Mastering Domain Logic
Embrace Domain-Driven Design (DDD)
Domain-Driven Design provides a set of principles and patterns for effectively capturing domain logic in software systems. By following DDD principles such as ubiquitous language, bounded contexts, and aggregate roots, you can ensure that domain logic is well-modeled and aligned with the business domain.
Rich Domain Model
Favor a rich domain model over an anemic one by encapsulating both data and behavior within domain objects. This approach promotes better encapsulation, reduces procedural code, and fosters a more expressive and maintainable domain model.
// Rich Domain Model
public class Order {
private Long id;
private List<Item> items;
public void processOrder() {
// Encapsulate business logic within the domain method
}
}
Separation of Concerns
Adhere to the principle of separation of concerns by isolating domain logic from infrastructure and presentation layers. Use domain services and repositories to handle cross-cutting concerns while keeping the domain model clean and focused on business logic.
Domain Events and Asynchronous Processing
Utilize domain events and asynchronous processing to decouple and orchestrate complex domain logic. Domain events enable the modeling of asynchronous, loosely coupled interactions between domain objects, promoting flexibility and scalability.
Test-Driven Development (TDD)
Apply Test-Driven Development to validate and refine domain logic incrementally. Write unit tests that capture the expected behavior of domain objects and use them as a feedback mechanism to drive the design and implementation of domain logic.
Final Thoughts
Mastering domain logic in Java requires a deep understanding of the business domain, a commitment to object-oriented principles, and a focus on creating a cohesive, expressive domain model. By avoiding common mistakes in domain logic design and embracing best practices such as Domain-Driven Design, rich domain models, separation of concerns, and test-driven development, you can build robust and maintainable software systems that accurately reflect the complexities of the business domain.
To delve deeper into the world of Java, domain logic, and best practices in software design, check out our Java Development Guide for comprehensive insights and tutorials.
Remember, the mastery of domain logic is not just about writing code, but also about crafting a coherent and elegant representation of the real-world problem your software is solving.