Mastering Docker: A Java Developer's Guide to Success
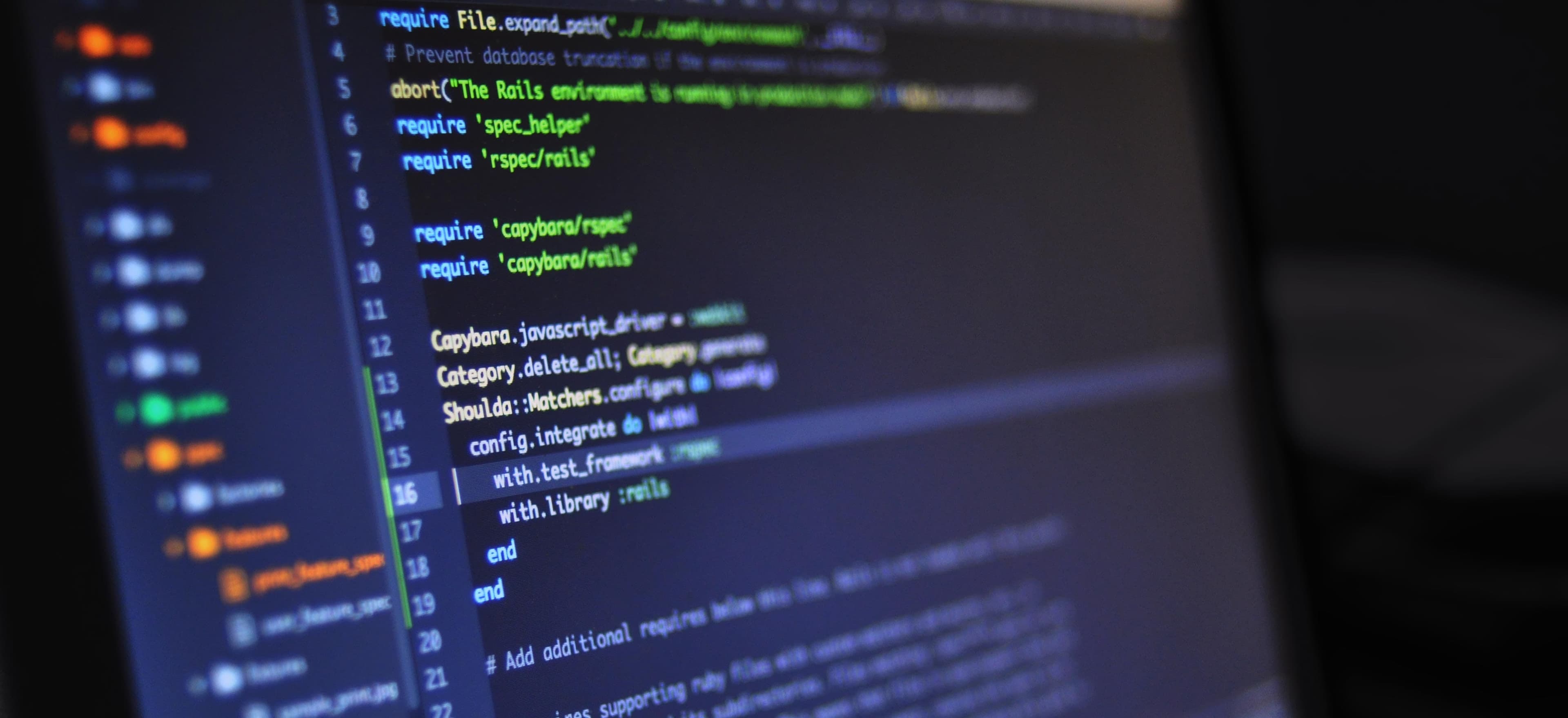
- Published on
Mastering Docker: A Java Developer's Guide to Success
Are you a Java developer looking to streamline your development process and improve the scalability and flexibility of your applications? Look no further than Docker. In this blog post, we will explore how Java developers can leverage Docker to streamline their development workflow, improve application scalability, and simplify deployment.
What is Docker?
At its core, Docker is a platform for developing, shipping, and running applications using containerization. Containers allow developers to package up an application with all of its dependencies, such as libraries and other binaries, and ship it as a single unit. This approach provides consistency across environments, from development to production, and allows for seamless scaling and deployment.
Why Java Developers Should Embrace Docker
As a Java developer, you may already be familiar with the challenges of managing dependencies and ensuring that your applications run consistently across different environments. Docker solves these challenges by providing a standard way to package and run applications, regardless of the underlying infrastructure.
By adopting Docker, Java developers can create lightweight, portable containers that encapsulate their applications and all necessary dependencies. This not only simplifies the development process but also ensures that the application will run the same way in any environment.
Getting Started with Docker and Java
Installation
To get started with Docker, you'll need to install Docker on your local machine. Once Docker is installed, you can begin creating and running containers for your Java applications.
Writing a Dockerfile
The first step in containerizing a Java application is to create a Dockerfile
. This file contains instructions for building a Docker image, which serves as a lightweight, standalone, and executable software package that includes everything needed to run a piece of software, including the code, a runtime, libraries, environment variables, and more.
Here's an example of a simple Dockerfile
for a Java application:
# Use the official OpenJDK image as the base image
FROM openjdk:11
# Set the working directory in the container
WORKDIR /app
# Copy the application JAR file into the container
COPY target/my-java-app.jar /app
# Specify the command to run the application
CMD ["java", "-jar", "my-java-app.jar"]
In this Dockerfile
, we use the official OpenJDK image as the base image, set the working directory, copy the application JAR file into the container, and specify the command to run the application. This Dockerfile
will be used to build a Docker image for our Java application.
Building and Running the Docker Image
Once the Dockerfile
is ready, you can build a Docker image using the docker build
command:
docker build -t my-java-app .
After the image is built, you can run a container based on that image using the docker run
command:
docker run my-java-app
By following these simple steps, you can containerize your Java application and run it within a Docker container.
Leveraging Docker Compose for Java Development
As your Java application grows in complexity, you may find yourself needing to run multiple containers, such as a database, alongside your Java application. Docker Compose is a tool for defining and running multi-container Docker applications. With Docker Compose, you can use a single YAML file to configure your application's services, networks, and volumes.
Here's an example docker-compose.yml
file for running a Java application alongside a MySQL database:
version: '3.8'
services:
app:
build: .
ports:
- "8080:8080"
depends_on:
- db
db:
image: mysql:5.7
environment:
MYSQL_ROOT_PASSWORD: password
MYSQL_DATABASE: my_database
MYSQL_USER: user
MYSQL_PASSWORD: password
ports:
- "3306:3306"
In this example, we define two services: app
and db
. The app
service is built from the current directory (.
), maps port 8080 on the host to port 8080 in the container, and depends on the db
service. The db
service uses the official MySQL 5.7 image, sets environment variables for the MySQL instance, and maps port 3306 on the host to port 3306 in the container.
By using Docker Compose, Java developers can easily define and run multi-container applications, simplifying the development and testing process.
Integration with Continuous Integration/Continuous Deployment (CI/CD) Pipelines
Docker plays a crucial role in CI/CD pipelines by providing a consistent environment for building, testing, and deploying applications. When integrated with tools like Jenkins, Travis CI, or GitLab CI/CD, Docker can help automate the entire software delivery process.
By utilizing Docker in CI/CD pipelines, Java developers can ensure that their applications are tested and deployed in consistent environments, leading to improved reliability and faster release cycles.
Final Considerations
In conclusion, Docker is a powerful tool for Java developers, offering a standardized, consistent, and scalable approach to building, shipping, and running applications. By containerizing Java applications with Docker, developers can streamline their development process, improve scalability, and simplify deployment.
Docker's integration with Java development provides an efficient way to manage dependencies, run multi-container applications, and integrate with CI/CD pipelines, ultimately leading to more reliable and efficient software delivery.
Incorporating Docker into your Java development workflow can bring about a paradigm shift, making your development process more efficient, scalable, and reliable. Embrace Docker today, and elevate your Java development to new heights.