Unveiling Hidden Bugs with Static Code Analysis
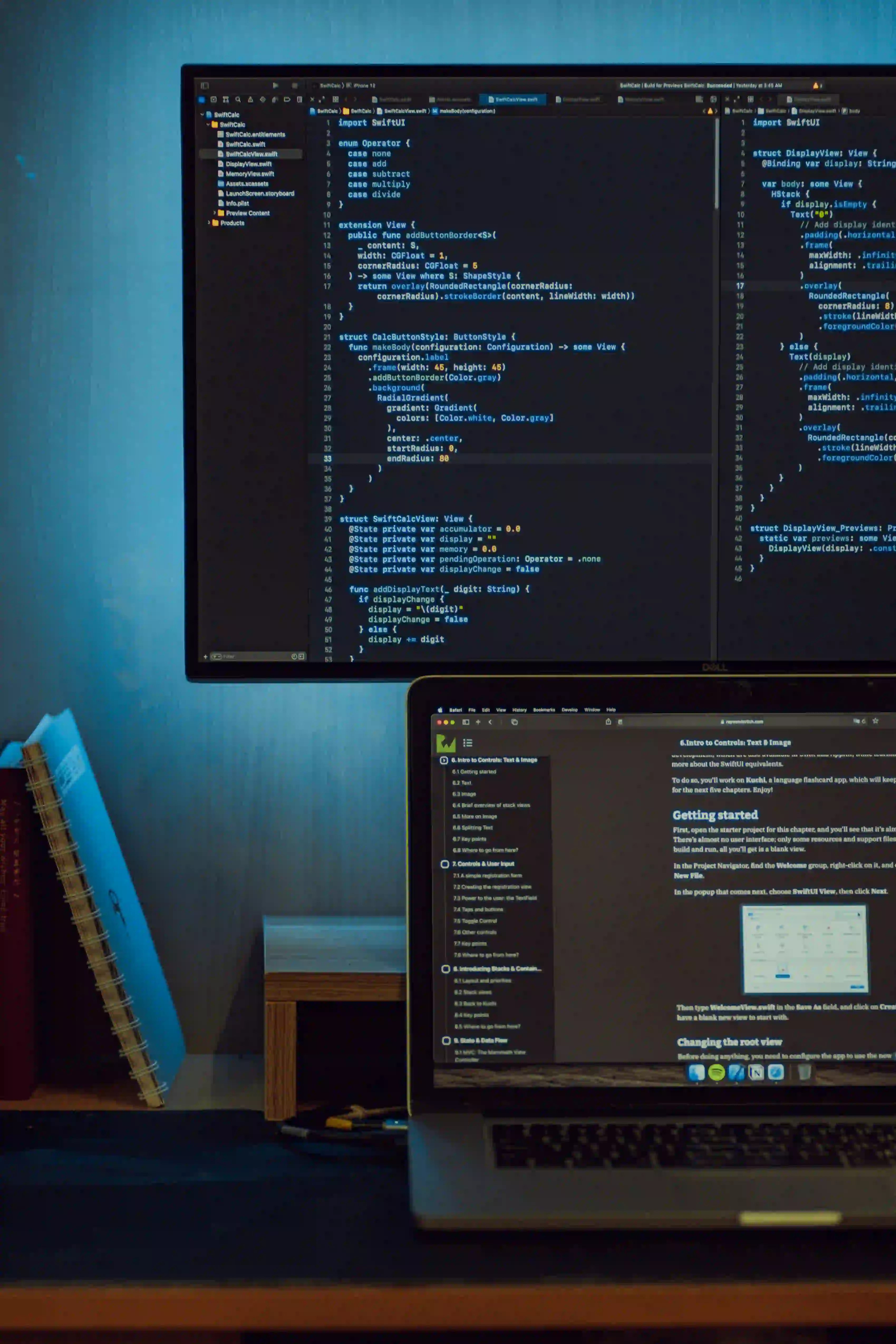
Unveiling Hidden Bugs with Static Code Analysis
Static code analysis is a technique that can help developers find bugs and security vulnerabilities in their code before it's even executed. By analyzing the code without actually running it, static analysis tools can catch potential issues early in the development process. In this blog post, we'll explore the concept of static code analysis, its benefits, and how it can be used to unveil hidden bugs in Java code.
What is Static Code Analysis?
Static code analysis is the process of analyzing code without executing it. This analysis can be performed by using specialized tools that examine the code for potential issues such as coding errors, security vulnerabilities, and other bugs.
Why Use Static Code Analysis?
Using static code analysis has several benefits:
- Early Bug Detection: Bugs are caught early in the development cycle, reducing the cost and effort needed to fix them.
- Consistent Code Quality: It helps maintain a high level of code quality across the codebase.
- Security: Identifying security vulnerabilities before deployment helps in building secure applications.
Using Static Code Analysis in Java
In the world of Java, there are several static code analysis tools available. One popular tool is FindBugs, which can analyze Java bytecode and identify potential bugs. Let's take a look at an example of using FindBugs to unveil hidden bugs in Java code.
public class Example {
public void doSomething(int[] values) {
for (int i = 0; i <= values.length; i++) {
// do something with values
}
}
}
In this example, FindBugs can identify a potential ArrayIndexOutOfBoundsException because the loop condition i <= values.length
should be i < values.length
. This simple mistake could lead to a runtime error, but static code analysis helps to catch it before the code is even executed.
Key Benefits of Using Static Code Analysis in Java
- Identifying Null Pointer Dereferences: Static analysis tools can flag potential null pointer exceptions, helping developers avoid these common runtime errors.
- Maintaining Coding Standards: Tools like Checkstyle can be used to enforce coding standards, ensuring a consistent code style across the project.
- Resource Leaks Detection: Static analysis tools can detect potential resource leaks, such as unclosed streams or connections, which can lead to performance issues.
Integrating Static Code Analysis into the Development Process
To reap the benefits of static code analysis, it needs to be integrated into the development process. This can be achieved by incorporating the analysis tools into the build process using build automation tools such as Maven or Gradle.
Here's an example of how FindBugs can be integrated into a Maven project:
<build>
<plugins>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>findbugs-maven-plugin</artifactId>
<version>3.0.5</version>
<configuration>
<effort>Max</effort>
<threshold>Low</threshold>
</configuration>
<executions>
<execution>
<goals>
<goal>check</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
In this example, the FindBugs Maven plugin is added to the build process. When the project is built, FindBugs will analyze the code and report any potential issues based on the specified configuration.
Benefits of Integration
- Automated Checks: Static code analysis is performed automatically as part of the build process, ensuring that all new code additions are analyzed.
- Consistent Analysis: With integration into the build process, all developers benefit from the same analysis tool and configuration.
Wrapping Up
In conclusion, static code analysis is a valuable tool for unveiling hidden bugs in Java code. By leveraging static analysis tools like FindBugs and integrating them into the development process, developers can catch potential issues early, maintain code quality, and build more secure and robust applications. Incorporating static code analysis into the development workflow is an investment that pays off by reducing the likelihood of bugs and enhancing the overall quality and security of the codebase.
To delve deeper into static code analysis and its impact on Java development, check out XYZ's comprehensive guide on Best Practices for Static Code Analysis in Java. Additionally, to stay updated on the latest trends and tools in Java development, explore ABC's insights on Improving Code Quality with Static Analysis Tools.
Remember, empowering your development process with static code analysis is a proactive step towards delivering reliable and high-quality software. So, embrace the power of static code analysis and let it pave the way for robust and bug-free Java applications!