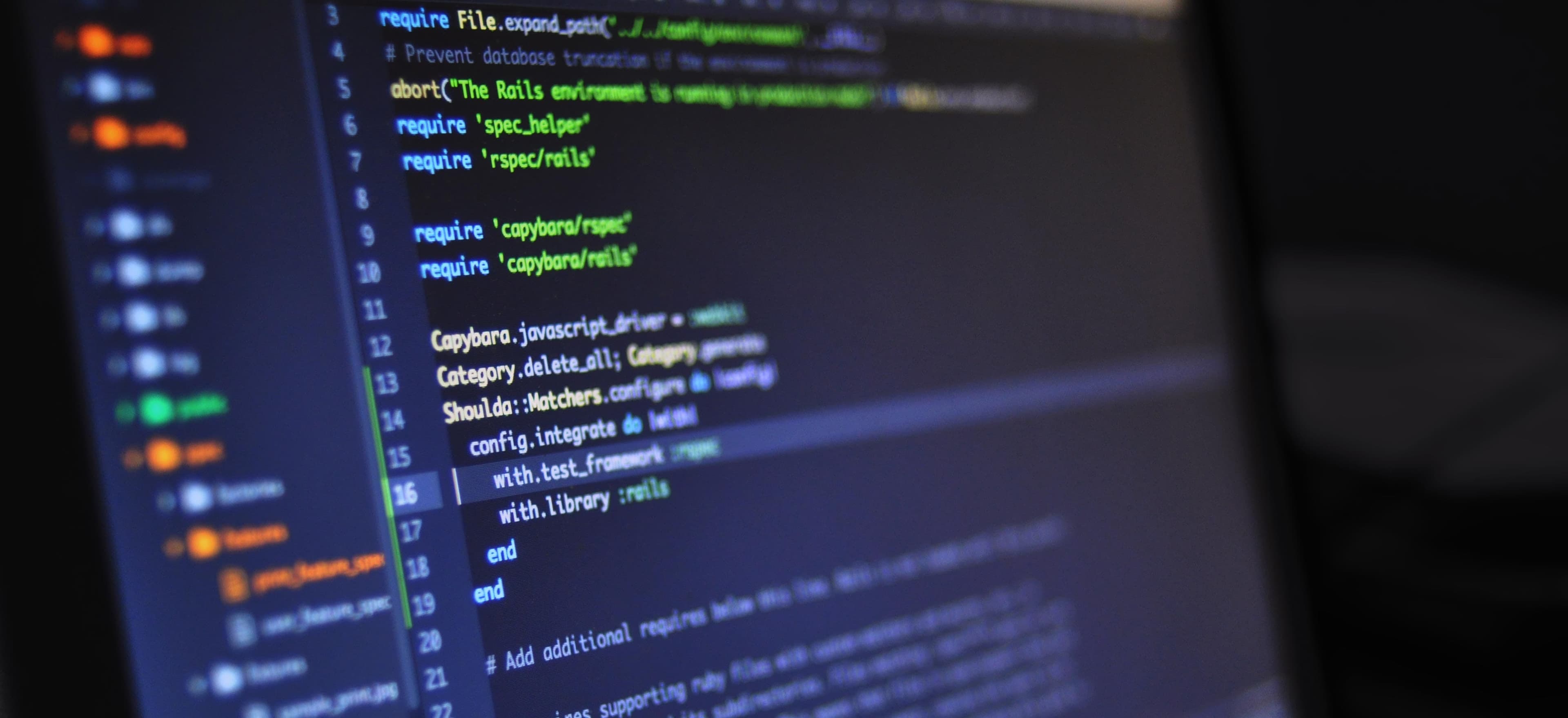
- Published on
Securing Grails: Mastering Callback Closures in Spring Security
When it comes to securing your Grails application, Spring Security is an indispensable tool. However, there's more to Spring Security than just configuring access rules. One powerful feature that often goes underutilized is the use of callback closures. In this post, we'll delve into mastering callback closures in Spring Security to elevate the security of your Grails application to the next level.
What are Callback Closures?
Callback closures in Spring Security are essentially pieces of code that are executed at specific points during the authentication and authorization process. They allow you to hook into various stages of the security workflow and perform custom logic. This level of customization can be incredibly powerful when it comes to implementing complex security requirements.
The Power of Callback Closures
Callback closures empower you to exert fine-grained control over the behavior of Spring Security in your Grails application. By leveraging callback closures, you can inject custom behavior at crucial points in the authentication and authorization process. This could involve performing additional checks, logging, sending notifications, or even customizing the behavior based on dynamic conditions.
Understanding The Callback Closures
Let's take a deep dive into the practical aspects of callback closures in Spring Security.
Pre-Authentication Callbacks
The beforeAuth
closure allows you to perform custom logic before the authentication process takes place. This can be particularly handy for scenarios where you need to check for additional conditions or inject custom behavior before the user is authenticated.
import grails.plugin.springsecurity.SecurityConfig
class SecurityConfig extends SecurityConfig {
// ...
@Override
void init() {
super.init()
preAuthenticationChecks = { token ->
// Perform custom pre-authentication checks here
// e.g., additional validation, logging, etc.
}
}
}
The preAuthenticationChecks
closure intercepts the authentication process before it occurs and enables you to inject your custom logic.
Post-Authentication Callbacks
On the flip side, the afterAuth
closure enables you to execute custom logic after the authentication process has successfully completed.
import grails.plugin.springsecurity.SecurityConfig
class SecurityConfig extends SecurityConfig {
// ...
@Override
void init() {
super.init()
postAuthenticationChecks = { userDetails ->
// Perform custom post-authentication tasks here
// e.g., logging successful login, updating user details, etc.
}
}
}
The postAuthenticationChecks
closure provides an excellent opportunity to carry out tasks such as logging successful login attempts or updating user details.
Pre-Authorization Callbacks
When it comes to authorizing access to specific resources, the beforeAccess
closure is where you can intervene before the authorization decision is made.
import grails.plugin.springsecurity.SecurityConfig
class SecurityConfig extends SecurityConfig {
// ...
@Override
void init() {
super.init()
preInvocationChecks = { accessDecisionManager, authentication, configAttributes ->
// Perform custom pre-authorization checks here
// e.g., additional access control, dynamic role assignment, etc.
}
}
}
The preInvocationChecks
closure allows you to implement custom pre-authorization logic, such as dynamic role assignment or additional access control checks.
Post-Authorization Callbacks
Similarly, the afterAccess
closure provides a means to execute custom logic after the authorization decision has been made.
import grails.plugin.springsecurity.SecurityConfig
class SecurityConfig extends SecurityConfig {
// ...
@Override
void init() {
super.init()
postInvocationChecks = { accessDecisionManager, authentication ->
// Perform custom post-authorization tasks here
// e.g., logging access, auditing, post-authorization notification, etc.
}
}
}
The postInvocationChecks
closure is a great way to carry out tasks post-authorization, such as logging access, auditing, or sending post-authorization notifications.
Best Practices and Use Cases
Now that you have a grasp of the power and flexibility of callback closures in Spring Security, it's vital to consider best practices and use cases for leveraging them effectively.
Best Practices
- Keep the callback closures focused: Each closure should handle a specific aspect of the security workflow, promoting clarity and maintainability.
- Use callback closures for non-standard security requirements: Callback closures are ideal for implementing custom security logic that deviates from typical use cases.
Use Cases
- Custom Authentication Checks: Utilize pre-authentication callback closures to perform additional validation or logging before the user is authenticated.
- Post-Authentication Tasks: Employ post-authentication callback closures for activities like updating user details or triggering notifications after successful login.
- Dynamic Authorization Decisions: Leverage pre-authorization callback closures for dynamic role assignment and additional access control logic.
- Post-Authorization Actions: Use post-authorization callback closures for tasks such as logging access, auditing, or triggering notifications after authorization decisions.
Bringing It All Together
Incorporating callback closures into your Spring Security configuration in a Grails application can significantly enhance the security posture. By mastering these callback closures, you gain the ability to insert custom logic at precisely the right moments in the security workflow, catering to diverse and complex security requirements.
Optimizing the use of callback closures not only elevates the security functionality but also fosters a deeper understanding of the Spring Security framework. Thus, dive into callback closures, embrace their flexibility, and fortify the security of your Grails application with precision and finesse.
For further understanding and exploration of Spring Security in Grails, you can delve into the official Spring Security documentation. Additionally, the Grails official documentation provides comprehensive insights into building secure and robust Grails applications.
Remember, in the realm of Grails application security, mastering callback closures in Spring Security — a small step for code, a giant leap for safeguarding your application!
Happy coding, and may your Grails applications be fortified with formidable security!