Sequelize-ORM Woes: Tackling Association Pitfalls in Node.js
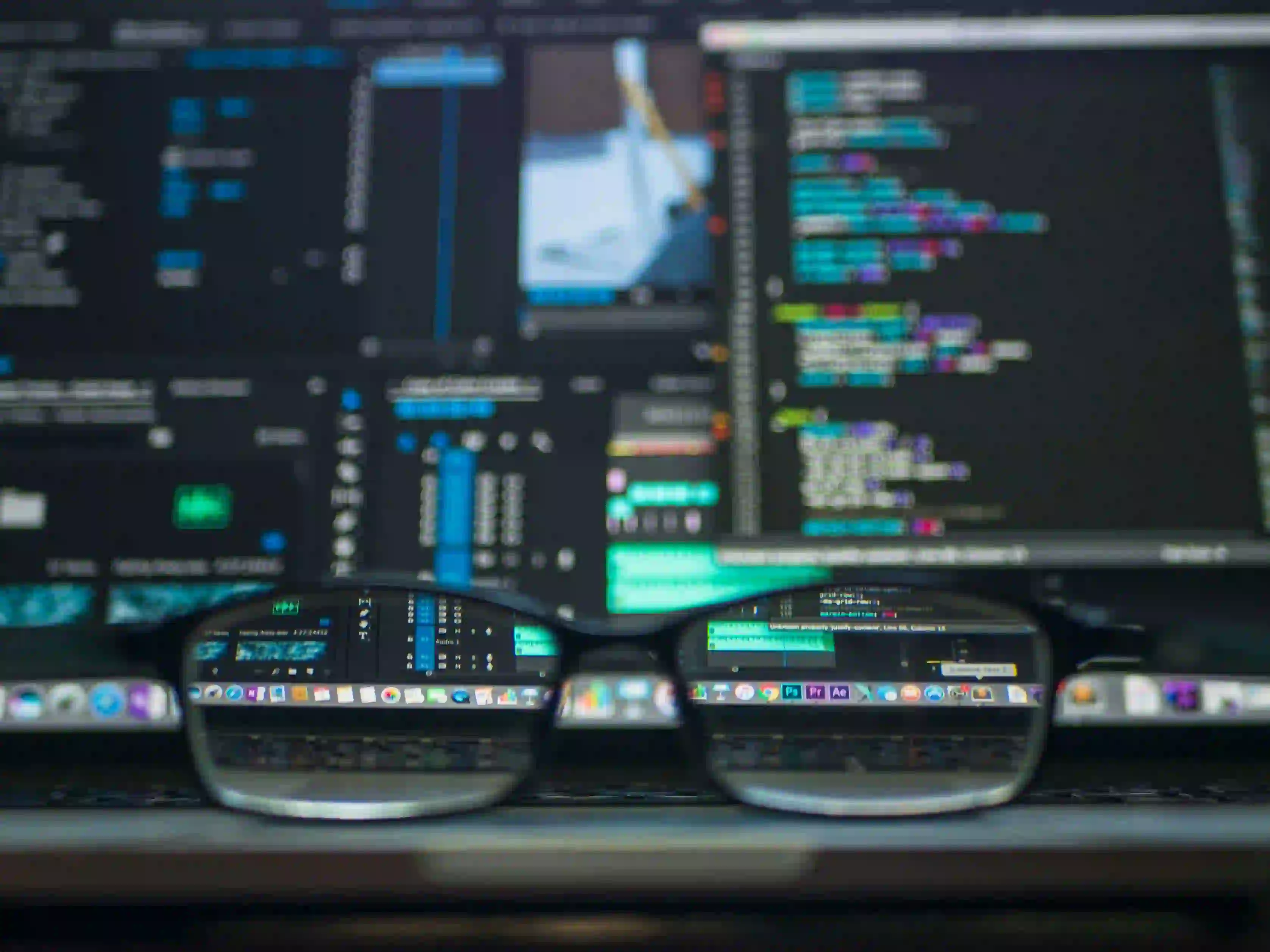
Tackling Association Pitfalls in Node.js with Sequelize-ORM
Node.js has become one of the most popular platforms for building backend applications, and Sequelize-ORM is often the go-to choice for working with databases. However, when it comes to handling associations in Sequelize, developers often encounter pitfalls that can lead to frustration and confusion.
In this blog post, we will delve into some common association-related challenges in Sequelize-ORM and discuss effective strategies for overcoming them. From understanding the different association types to tackling common pitfalls, this guide aims to provide a comprehensive resource for developers navigating the intricacies of Sequelize associations in their Node.js applications.
Understanding Sequelize Associations
Sequelize-ORM provides a powerful set of tools for defining and managing associations between database tables. Associations allow you to establish relationships between models, enabling you to retrieve related data and perform complex queries with ease.
Association Types
Sequelize supports several types of associations, including:
-
One-to-One: An association where a record in one table is associated with exactly one record in another table.
-
One-to-Many: An association where a record in one table is associated with multiple records in another table.
-
Many-to-Many: An association where records in one table are associated with multiple records in another table, and vice versa.
Each type of association has its own use cases and implications, and understanding them is crucial for building efficient and effective database schemas.
Common Pitfalls and How to Tackle Them
Pitfall 1: Improper Association Definitions
One common pitfall when working with Sequelize associations is improperly defining the associations between models. This can lead to errors when querying related data or performing operations that depend on the associations.
Let's take a look at an example of defining a one-to-many association between User
and Post
models:
// User model
const User = sequelize.define('user', {
// attributes
});
// Post model
const Post = sequelize.define('post', {
// attributes
});
User.hasMany(Post); // Define one-to-many association
In this example, the User.hasMany(Post)
statement establishes a one-to-many association, allowing us to retrieve all posts associated with a user. It's crucial to ensure that the association definitions accurately reflect the intended relationships between models.
Pitfall 2: Inconsistent Data Retrieval
Another common pitfall occurs when inconsistently retrieving associated data, leading to unexpected behavior or incomplete results. When querying related data, it's essential to use the appropriate Sequelize methods to ensure that the associated records are fetched correctly.
Consider the following example, where we retrieve a user and their associated posts:
User.findByPk(1, { include: Post })
.then(user => {
console.log(user.posts); // Access associated posts using proper attribute
});
In this example, the { include: Post }
option ensures that the associated Post
records are properly included in the query results. Failing to use the include
option can result in incomplete data retrieval, a common pitfall that can cause confusion during development.
Pitfall 3: Eager Loading Overload
Eager loading, a feature in Sequelize that allows you to retrieve associated data in a single query, can lead to performance issues when not used judiciously. Loading too much data eagerly can result in unnecessary overhead and slow down your application.
It's important to carefully consider which associations need to be eagerly loaded and which can be lazily loaded based on specific use cases. Utilizing Sequelize's eager loading capabilities effectively is essential for optimizing performance and ensuring efficient data retrieval.
Best Practices for Sequelize Associations
Now that we've explored common pitfalls, let's discuss best practices for working with associations in Sequelize-ORM.
Use Descriptive Association Names
When defining associations between models, it's beneficial to use descriptive and intuitive names that accurately reflect the nature of the relationship. Clear and consistent association names make your code more readable and understandable, contributing to better maintainability and collaboration within your development team.
Leverage Migration Scripts for Association Changes
When making changes to associations, such as adding or removing associations between models, it's crucial to leverage Sequelize migration scripts. Migration scripts allow you to version-control and apply incremental changes to your database schema, ensuring that association modifications are handled consistently and reproducibly.
Implement Data Validation and Integrity Checks
Maintaining data integrity is paramount when working with associations in Sequelize. Implementing data validation checks, such as ensuring foreign key constraints and enforcing referential integrity, helps prevent data inconsistencies and ensures the reliability of your database relationships.
Lessons Learned
Navigating association pitfalls in Sequelize-ORM can be challenging, but with a solid understanding of association types, common pitfalls, and best practices, developers can effectively tackle these challenges in their Node.js applications. By mastering Sequelize associations, you can build robust and performant database schemas that power your applications with efficiency and reliability.
In conclusion, Sequelize-ORM offers a powerful toolkit for managing associations, and by staying mindful of best practices and potential pitfalls, developers can harness its capabilities to build robust and performant applications.
Let's continue the discussion: How do you approach Sequelize association challenges in your Node.js projects? Share your insights and experiences in the comments below!