Serializable vs Parcelable: Optimizing Android Intents
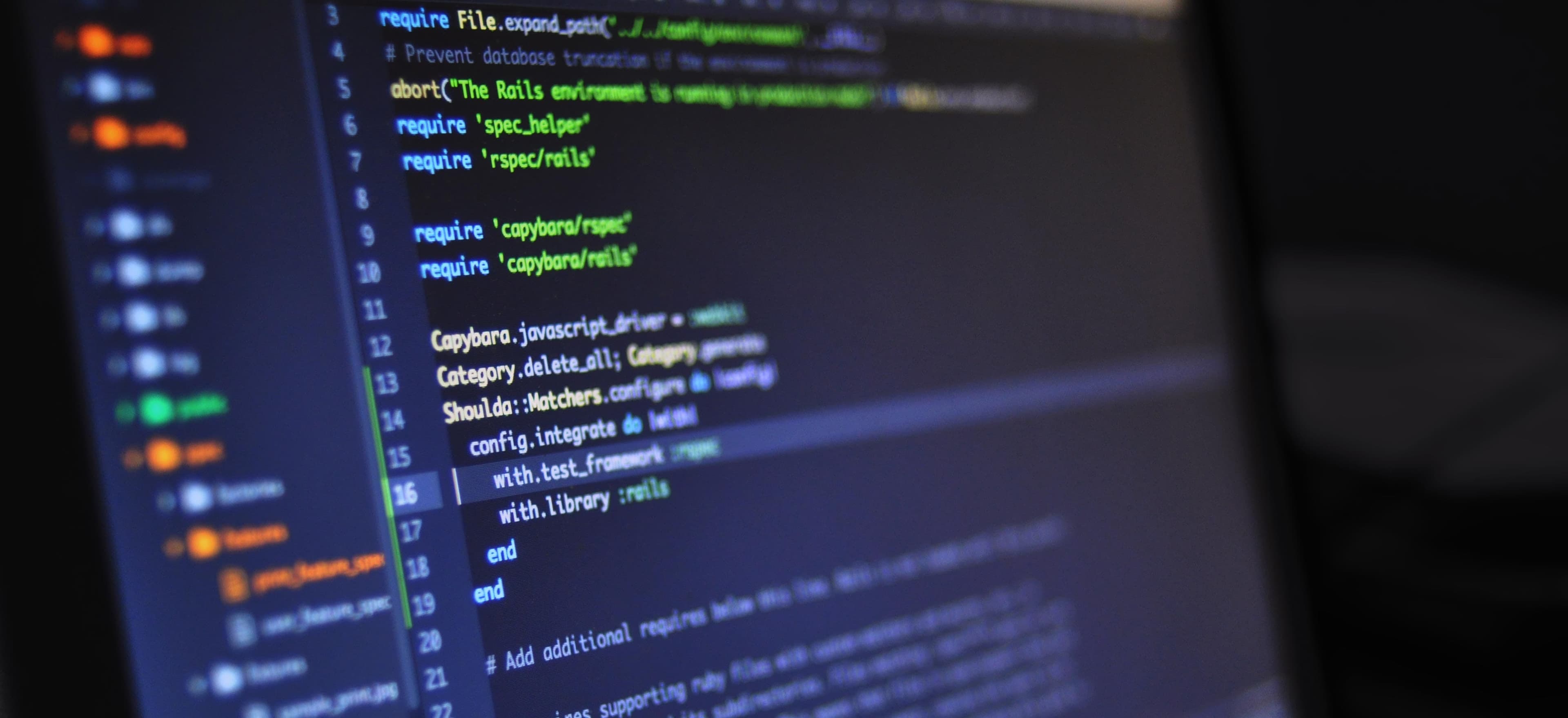
- Published on
Serializable vs Parcelable: Optimizing Android Intents
When it comes to passing data between Android components such as Activities, Fragments, or Services, using Intents is a common practice. Android provides two main mechanisms for passing objects within Intents: Serializable
and Parcelable
. In this article, we'll delve into the differences between these two approaches and discuss why Parcelable
is often preferred for better performance in Android applications.
Serializable
Serializable
is a marker interface that allows objects to be serialized. When an object is serialized, its state is converted into a byte stream, which can be stored in a file or sent over the network. In the context of Android, Serializable
can be used to pass objects between components via Intents.
Example using Serializable
public class Person implements Serializable {
private String name;
private int age;
// Getters and setters
}
When passing a Serializable
object through an Intent:
Intent intent = new Intent(this, NextActivity.class);
Person person = new Person("John", 25);
intent.putExtra("person", person);
startActivity(intent);
While using Serializable
is straightforward, it has some performance drawbacks, especially in the context of Android applications. The process of serializing and deserializing objects using Serializable
can be computationally expensive and can lead to increased memory usage.
Parcelable
Parcelable
is an Android-specific interface that allows objects to be serialized with the focus on performance. Unlike Serializable
, Parcelable
requires the developer to implement serialization and deserialization methods explicitly.
Example using Parcelable
public class Person implements Parcelable {
private String name;
private int age;
// Constructor, getters, and setters
// Parcelable implementation
@Override
public void writeToParcel(Parcel dest, int flags) {
dest.writeString(name);
dest.writeInt(age);
}
protected Person(Parcel in) {
name = in.readString();
age = in.readInt();
}
}
When passing a Parcelable
object through an Intent:
Intent intent = new Intent(this, NextActivity.class);
Person person = new Person("John", 25);
intent.putExtra("person", person);
startActivity(intent);
Implementing Parcelable
requires more effort compared to Serializable
, but it offers better performance and is optimized for Android's environment.
Performance Comparison
While Serializable
is easier to implement, Parcelable
outperforms Serializable
in terms of speed and resource usage, which is crucial in the mobile environment where performance is a key factor.
The serialization and deserialization process with Parcelable
is faster compared to Serializable
due to the explicit implementation of serialization methods. Additionally, Parcelable
is designed to work efficiently within the Android framework, resulting in reduced memory overhead and faster object retrieval.
Best Practices and Considerations
When to use Serializable
- For small, simple objects where performance is not a critical factor.
- When dealing with legacy code or third-party libraries that rely on
Serializable
.
When to use Parcelable
- For larger and more complex objects that require better performance.
- In scenarios where object serialization and deserialization performance is essential, such as passing data between activities or fragments frequently.
The Bottom Line
In conclusion, while both Serializable
and Parcelable
can be used to pass objects between Android components, Parcelable
is the recommended approach for better performance and efficiency. When designing Android applications, especially those targeting mobile devices, optimizing performance is crucial for providing a smooth user experience. By understanding the differences between Serializable
and Parcelable
and choosing the appropriate approach based on performance requirements, developers can ensure efficient data transmission within their Android applications.
In summary, when it comes to optimizing Android Intents, choosing Parcelable
over Serializable
can lead to improved performance and better resource utilization, contributing to a more responsive and efficient app.