MVC Explained: Solving the Misunderstanding Epidemic
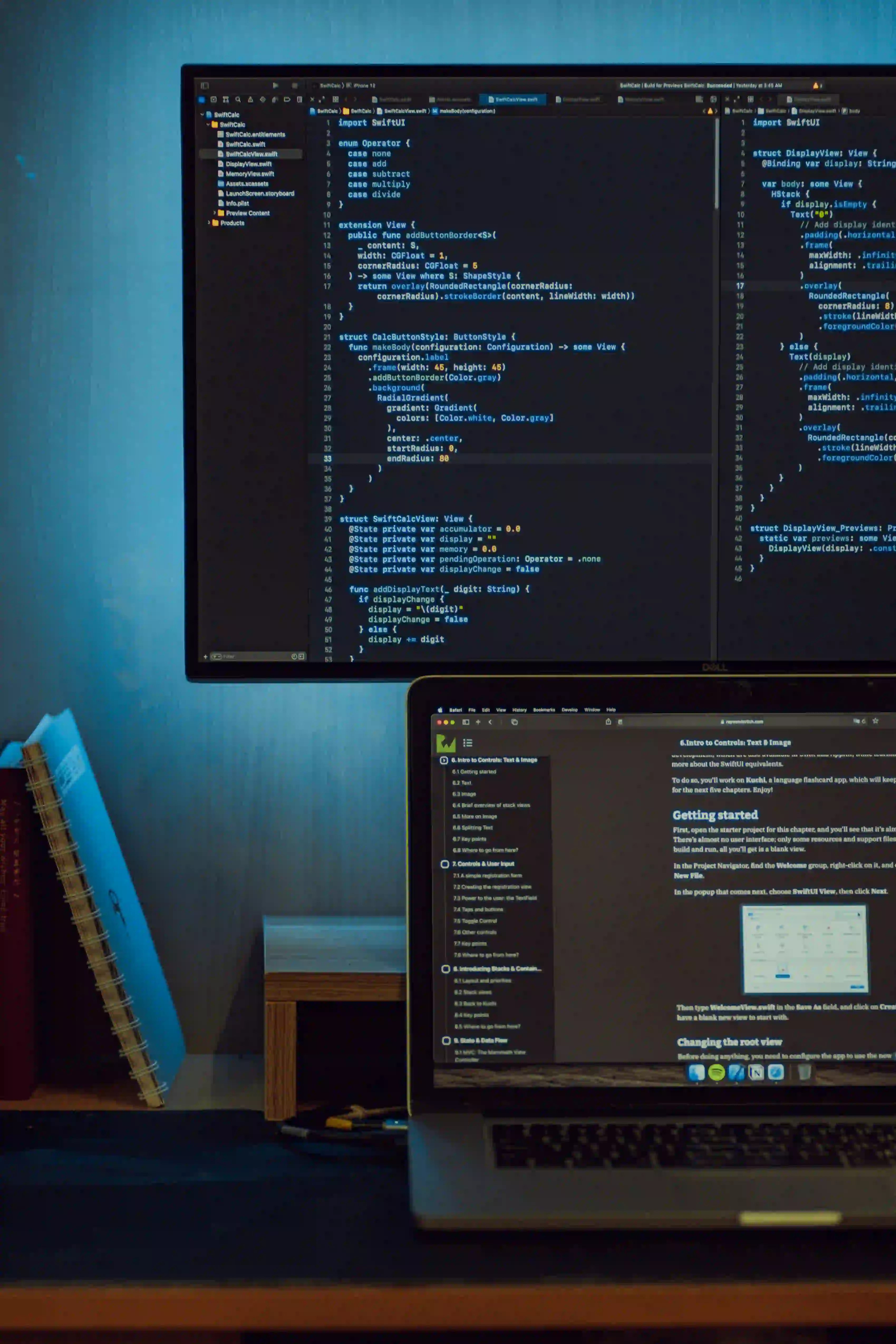
MVC Explained: Solving the Misunderstanding Epidemic
Stepping into the Topic
Model-View-Controller (MVC) is a widely used architectural pattern in software development. It provides a structured approach to organizing code and designing applications, promoting modularity, reusability, and maintainability. However, there are many misconceptions and misunderstandings surrounding MVC. This article aims to clear these misconceptions and provide a comprehensive understanding of MVC, with a focus on its implementation in Java projects. Understanding MVC can significantly enhance one's software development skills, enabling developers to create more robust and scalable applications.
What is MVC?
The Concept
At its core, MVC is a design pattern that separates the components of an application into three interconnected parts: the Model, the View, and the Controller.
The primary purpose of this separation is to achieve a clear division of concerns:
- The Model deals with the application's data and business logic.
- The View handles the presentation and user interface.
- The Controller acts as an intermediary, managing user input, updating the Model, and selecting the appropriate View to display the data.
For those interested in delving deeper into the origins of MVC, the seminal paper by Trygve Reenskaug, "Models: A Roadmap for the User," provides an excellent source of insights into the early concept and its evolution.
The Components
Model
The Model in MVC represents the application's data and its logic. It encapsulates the code responsible for interacting with the database, processing data, and enforcing business rules. In Java, a simple representation of the Model can be demonstrated with a class representing a user entity.
public class User {
private String name;
private String email;
// Constructor, getters, setters, and methods for data interaction
}
View
The View component is responsible for presenting the data to the user and handling user interface interactions. In a Java application, the View could be an HTML template or a scene in a JavaFX application, displaying user information and allowing interactions with the data.
Controller
The Controller acts as the bridge between the Model and the View. It receives user input, manipulates the Model as required, and determines which View to present to the user. In Java, a Controller class might handle user requests, fetch data from the Model, and control the navigation between different Views.
How They Work Together
In the MVC pattern, the flow of information typically follows this sequence:
- The user interacts with the View, triggering an action.
- The Controller receives the user's input and processes the request.
- The Controller updates the Model based on the user input.
- The Model notifies the View about changes in the data, prompting the View to update its display to reflect the new state.
This continuous interaction between the Model, View, and Controller forms the basis of the MVC design pattern, ensuring a separation of concerns and facilitating modularity and maintainability.
Common Misunderstandings
-
Misconception 1: Views must be graphical.
- Correction: While many applications implement graphical Views, this is not a strict requirement. Views can be any form of user interface, including command-line interfaces, APIs, or even non-graphical representations of data.
-
Misconception 2: Controllers are unnecessary in small applications.
- Correction: Even in small applications, separating concerns and maintaining a clear structure is beneficial. Controllers facilitate the interaction between the Model and the View, promoting scalability and maintainability.
-
Misconception 3: The Model must represent a database entity.
- Correction: While the Model often interacts with a database, it can also encapsulate business logic, data processing, or any other data-related functionality, not restricted to database operations.
Addressing these misconceptions is crucial for understanding the flexibility and utility of MVC in various application scenarios.
MVC in Java: A Practical Example
To demonstrate the implementation of MVC in a Java environment, let's consider a simple to-do list application.
Setting Up
Before diving into the implementation, ensure that Java Development Kit (JDK) and your preferred Integrated Development Environment (IDE) are installed and set up.
The Model
For the to-do list example, the Model might consist of a class representing a to-do item. It would handle tasks such as creating, updating, and deleting tasks.
public class TodoItem {
private String task;
private boolean isCompleted;
// Constructor, getters, setters, and methods for task operations
}
The View
In our JavaFX-based UI for the to-do list, the View would comprise the graphical user interface that presents the to-do items and allows users to interact with them.
// Code example for creating a JavaFX scene representing the to-do list
The Controller
The Controller for the to-do list application would manage user inputs, update the Model, and control the navigation between different Views. It would handle actions such as creating new tasks or marking tasks as completed.
// Code example for a to-do list Controller class
By employing MVC in this practical example, the application’s codebase becomes structured and organized, enhancing its maintainability and flexibility.
Best Practices When Using MVC
When implementing MVC in Java projects, consider the following best practices:
- Scalability: Design the application with scalability in mind, ensuring that as the application grows, the structure maintains its clarity and ease of maintenance.
- Maintaining Clean Separation of Concerns: Ensure that each component—Model, View, and Controller—remains focused on its specific area of concern without leaking into others, allowing for easier debugging and modification.
- Avoiding Tight Coupling: Minimize interdependencies between components to allow for easier testing and modification, promoting code reusability and maintainability.
Following these best practices can significantly improve the quality and longevity of the application when utilizing the MVC pattern.
Additional Resources
For further reading and learning about MVC and how to apply it in Java development, consider the following resources:
These resources cater to both beginners seeking to understand the fundamentals of MVC and more advanced learners looking to explore advanced application of this architectural pattern.
Final Thoughts
In conclusion, understanding the Model-View-Controller (MVC) pattern is fundamental for building well-organized and maintainable applications. By dispelling common misconceptions, discussing practical examples, and sharing best practices, this article aims to equip Java developers with the knowledge and insights necessary to confidently implement MVC in their projects. Embracing MVC not only enhances one's software development skills but also results in more robust and scalable applications.
Call to Action
We encourage readers to share their thoughts, experiences, or questions about MVC in the comments section. Additionally, feel free to share this article on social media or with peers who might benefit from a deeper understanding of MVC and its application in Java projects.
By understanding and applying MVC in Java development, developers can embark on a journey of creating well-structured, maintainable, and scalable software.
By adhering to the technical guidelines and providing an informative and engaging perspective on MVC in Java, we aim to demystify this architectural pattern, correct common misunderstandings, and offer practical insights for its application, ensuring real value for the reader.