Handling Polymorphic Objects in JSON Serialization
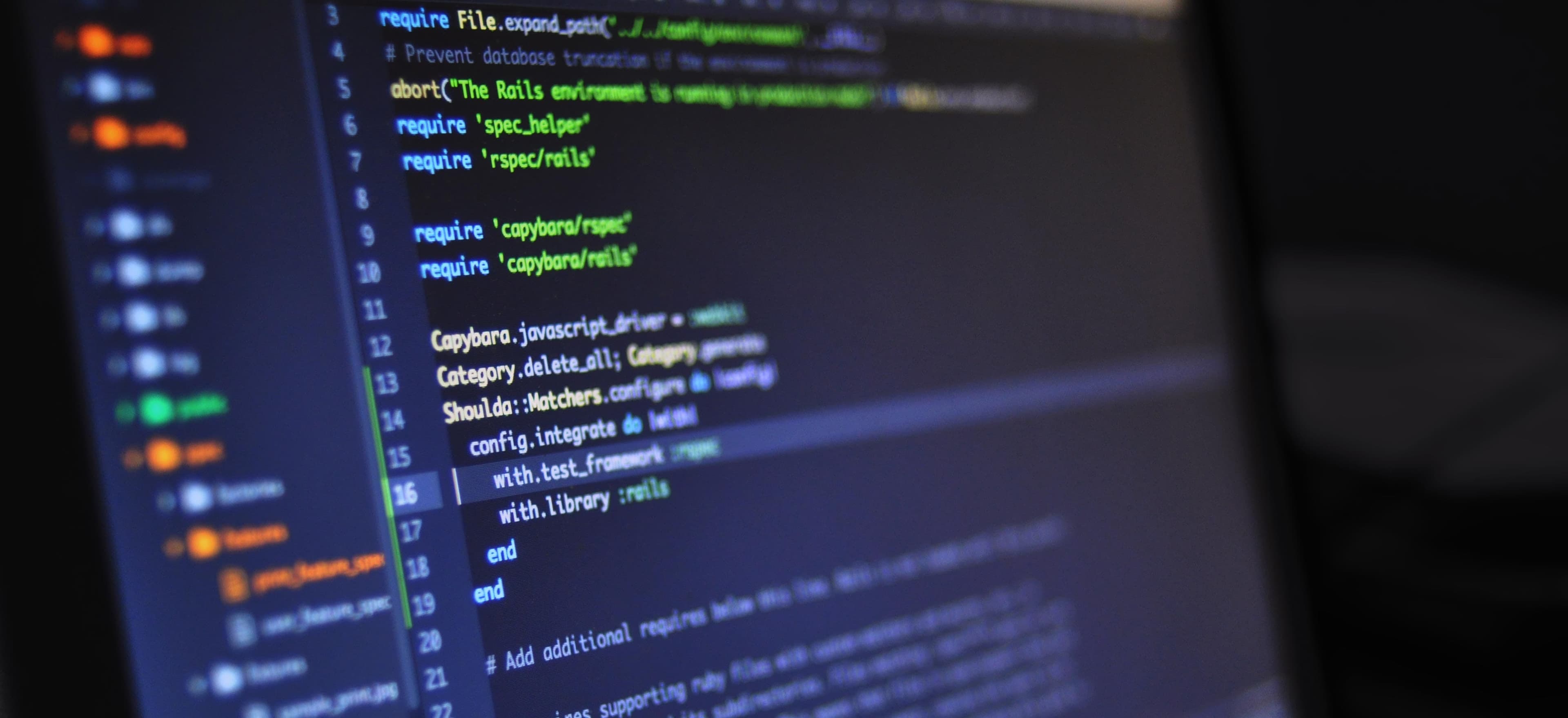
- Published on
Handling Polymorphic Objects in JSON Serialization
When working with Java and JSON, dealing with polymorphic objects can be tricky. In Java, polymorphism allows a reference variable to point to different types of objects at different times. However, when serializing these objects to JSON, we need to ensure that the type information is properly preserved and reconstructed during deserialization. In this blog post, we will explore how to handle polymorphic objects in JSON serialization using the Jackson library.
Understanding Polymorphic Objects
Before we dive into JSON serialization, let's revisit polymorphism in Java. Polymorphism allows a superclass reference variable to refer to a subclass object. For example, consider the following class hierarchy:
public class Shape {
// ...
}
public class Circle extends Shape {
// ...
}
public class Square extends Shape {
// ...
}
Here, both Circle
and Square
are subclasses of Shape
. With polymorphism, we can do the following:
Shape circle = new Circle();
Shape square = new Square();
JSON Serialization with Jackson
Jackson is a popular JSON processing library for Java. It provides easy ways to convert Java objects to JSON and vice versa. However, when dealing with polymorphic objects, extra care is needed to handle the type information correctly during serialization and deserialization. Let's see how we can achieve this using Jackson.
Adding Type Information for Serialization
When serializing polymorphic objects, we need to include type information in the JSON output. Jackson provides a few ways to achieve this. One common approach is to use the @JsonTypeInfo
annotation along with @JsonSubTypes
to indicate the type information for the subclasses.
Let's consider the modified Shape
hierarchy:
@JsonTypeInfo(use = JsonTypeInfo.Id.CLASS, include = JsonTypeInfo.As.PROPERTY, property = "@class")
@JsonSubTypes({
@JsonSubTypes.Type(value = Circle.class, name = "circle"),
@JsonSubTypes.Type(value = Square.class, name = "square")
})
public class Shape {
// ...
}
public class Circle extends Shape {
// ...
}
public class Square extends Shape {
// ...
}
In this example, we use @JsonTypeInfo
to include class information in the JSON output. The use
attribute specifies the mechanism to use, and the include
attribute determines where the type information should be included. The @JsonSubTypes
annotation is used to specify the available subtypes and their names.
Serializing Polymorphic Objects
Now that we've set up the type information, let's see how we can serialize polymorphic objects to JSON.
ObjectMapper mapper = new ObjectMapper();
String jsonString = mapper.writeValueAsString(shape);
In the above code, we use the ObjectMapper
class from Jackson to convert a Shape
object (which can be a Circle
or Square
due to polymorphism) to a JSON string. The resulting JSON will include the type information specified in the @JsonTypeInfo
annotation.
Deserializing Polymorphic Objects
When deserializing JSON with type information, Jackson can reconstruct the polymorphic objects correctly.
String json = "{\"@class\":\"circle\",\"radius\":5}";
Shape shape = mapper.readValue(json, Shape.class);
In this example, we deserialize the JSON string back to a Shape
object. Jackson recognizes the type information included in the JSON and reconstructs the appropriate subtype (Circle
in this case).
Key Takeaways
Handling polymorphic objects in JSON serialization with Java and Jackson requires the proper inclusion and utilization of type information. By using annotations such as @JsonTypeInfo
and @JsonSubTypes
, we can ensure that the type information is preserved during serialization and reconstructed during deserialization.
In this blog post, we've explored how to handle polymorphic objects in JSON serialization using the Jackson library. By understanding the intricacies of polymorphism and leveraging the features provided by Jackson, we can seamlessly work with polymorphic objects in our Java applications.
For more in-depth information on the topic, you can refer to the official Jackson documentation and the Java polymorphism guide.