Slash Estimation Errors: 5 Strategies for Devs to Master
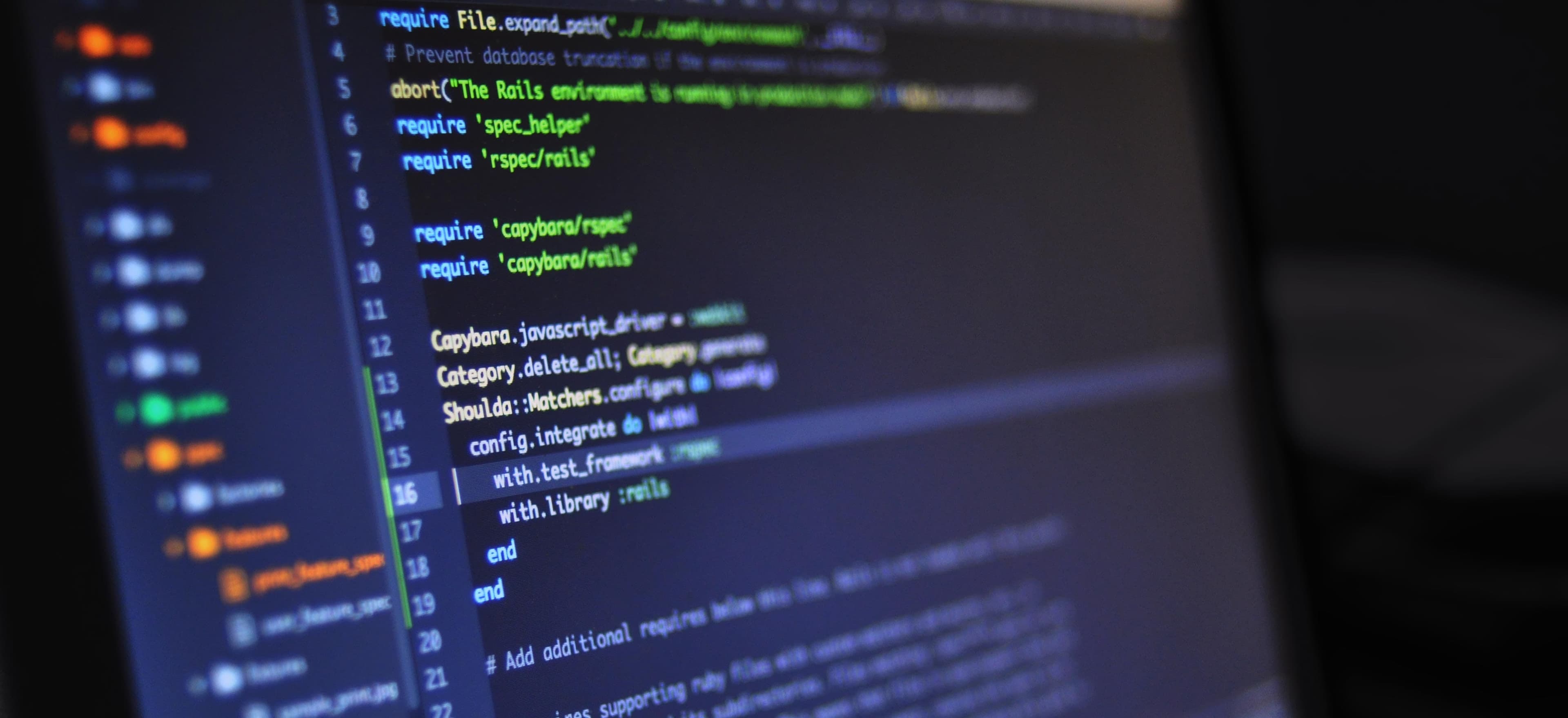
- Published on
Mastering Estimation in Java Development: 5 Strategies to Slash Errors
Estimation is an essential skill for Java developers, as it enables them to deliver projects on time and within budget. However, it's common for developers to struggle with estimation, leading to missed deadlines and cost overruns. In this post, we'll explore five strategies that Java developers can use to master estimation and slash errors.
1. Understand the Requirements Thoroughly
Before starting any Java development project, it's crucial to have a deep understanding of the requirements. Take the time to thoroughly analyze the project scope, including functional and non-functional requirements. By having a clear understanding of what needs to be built, developers can make more accurate estimations.
// Example of requirement analysis
public class RequirementAnalyzer {
public void analyzeRequirements(String projectScope) {
// Analyze the project scope here
}
}
2. Break Down the Tasks
Breaking down the project into smaller tasks can help in creating more accurate estimations. By breaking down the work, developers can identify potential challenges and dependencies, allowing for a more realistic estimation of the time and effort required for each task.
// Example of task breakdown
public class TaskBreakdown {
public void breakDownTasks(String projectScope) {
// Break down the tasks here
}
}
3. Leverage Historical Data
One effective strategy for estimation is to leverage historical data from previous Java projects. By analyzing similar past projects, developers can gain insights into how long specific tasks took to complete. This historical data can serve as a valuable reference point for estimating new projects.
// Example of leveraging historical data
public class HistoricalDataAnalyzer {
public void analyzeHistoricalData(String projectType) {
// Analyze historical data for similar projects here
}
}
4. Use Estimation Techniques
There are several estimation techniques that Java developers can utilize to improve accuracy. Techniques such as Three-Point Estimation or Planning Poker can help in considering best-case, worst-case, and most likely scenarios, leading to more refined estimations.
// Example of Three-Point Estimation
public class ThreePointEstimation {
public int estimateTaskTime(int bestCase, int worstCase, int mostLikely) {
return (bestCase + (4 * mostLikely) + worstCase) / 6;
}
}
5. Embrace Buffer Time
Unexpected issues and changes are inevitable in software development. By embracing buffer time in estimations, Java developers can account for these uncertainties. Adding a buffer for testing, code reviews, and potential setbacks can help in delivering more realistic estimates.
// Example of incorporating buffer time
public class BufferTimeEstimation {
public int estimateWithBuffer(int originalEstimate) {
int bufferPercentage = 20; // 20% buffer time
return originalEstimate + (originalEstimate * bufferPercentage / 100);
}
}
Wrapping Up
Mastering estimation is a continuous process, and by implementing these strategies, Java developers can minimize errors and deliver more accurate project timelines and budgets. Understanding requirements, breaking down tasks, leveraging historical data, using estimation techniques, and embracing buffer time are crucial steps towards becoming a proficient estimator. By honing their estimation skills, Java developers can enhance their project management abilities and contribute to successful project deliveries.
Remember, mastering estimation takes time and practice, but by employing these strategies, Java developers can significantly improve their estimation accuracy and ultimately become more efficient and reliable in their project deliveries.
Estimation is a critical aspect of software development, and mastering it can greatly contribute to the success of Java projects. Whether you are a seasoned developer or just starting, continuously honing your estimation skills can make a substantial difference in your professional growth and the success of your projects.
Start implementing these strategies today and witness a significant reduction in estimation errors while delivering projects more effectively and efficiently.
By continuously honing their estimation skills, Java developers can significantly contribute to successful project deliveries and enhance their project management abilities. Whether you are a seasoned developer or just starting, mastering estimation is essential for the success of Java projects. So, implement these strategies and witness a reduction in estimation errors while delivering projects more effectively and efficiently.
Mastering estimation is a critical skill for Java developers, and by utilizing these strategies, you can slash errors and deliver projects with confidence. Happy estimating!
Checkout our other articles