Unlocking Speed: How JDK 16's Vector API Solves Slow Computations
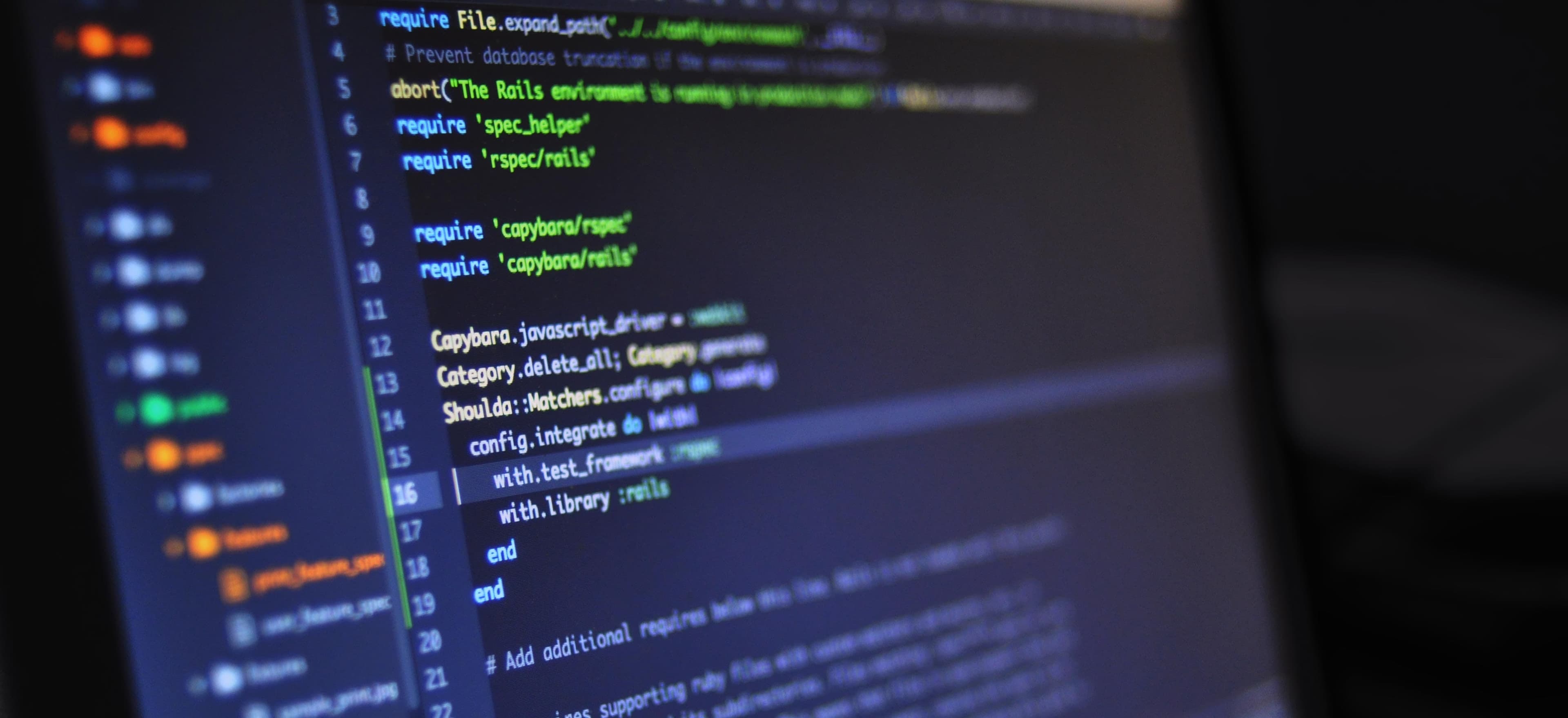
- Published on
Unlocking Speed: How JDK 16's Vector API Solves Slow Computations
In today's fast-paced world, performance is key. Whether we're working with large datasets, processing complex computations, or building high-speed applications, we need our code to run as fast as possible. Java, known for its portability and security, has sometimes been criticized for its performance in numerical computations. However, with the release of JDK 16, Java introduced the Vector API, a new, low-level API that aims to leverage modern CPU hardware to accelerate computations. In this article, we'll explore JDK 16's Vector API and how it can unlock speed by optimizing computations.
Understanding the Need for Speed
Before delving into the Vector API, it's important to understand why speed is crucial in computation-intensive applications. From scientific simulations and financial modeling to machine learning algorithms, performance directly impacts a system's efficiency and capability to handle large-scale tasks. The ability to perform operations on multiple data elements simultaneously, known as vectorization, can significantly accelerate computations, making it a sought-after feature in programming languages and frameworks.
Introducing JDK 16's Vector API
Traditionally, Java's approach to numerical computations involved using arrays or libraries like Apache Commons Math or ND4J. While these methods are functional, they might not fully exploit hardware capabilities for vector operations. The Vector API, introduced as an incubator module in JDK 16, provides a set of low-level APIs for vector computations, enabling developers to harness the power of modern CPU instructions for vector operations.
Let's take a look at how the Vector API can be used to improve the performance of computations.
import jdk.incubator.vector.FloatVector;
import jdk.incubator.vector.VectorSpecies;
// Define the vector size
VectorSpecies<Float> SPECIES = FloatVector.SPECIES_256;
// Perform vectorized addition
public void vectorizedAdd(float[] a, float[] b, float[] result) {
int i = 0;
for (; i < a.length - SPECIES.length(); i += SPECIES.length()) {
FloatVector va = FloatVector.fromArray(SPECIES, a, i);
FloatVector vb = FloatVector.fromArray(SPECIES, b, i);
va.add(vb).intoArray(result, i);
}
for (; i < a.length; i++) {
result[i] = a[i] + b[i];
}
}
In the above code snippet, we define a vector size and perform vectorized addition using the Vector API. This approach allows us to efficiently process multiple floating-point values in parallel, leveraging CPU instructions for vector operations.
Why Vector API Matters
The Vector API's significance lies in its ability to unlock the potential of modern CPU hardware. By allowing developers to write code that explicitly utilizes vector operations, the Vector API enables high-performance, low-latency computations, essential for a wide range of applications. Additionally, the Vector API reduces the reliance on external libraries and custom implementations, providing a standardized and optimized solution within the JDK.
Performance Gains and Limitations
When discussing performance improvements, it's important to consider the potential gains and limitations of the Vector API. By harnessing hardware-level parallelism, vectorization can lead to significant speedups in numerical computations, especially when dealing with large datasets. However, it's crucial to note that the effectiveness of vectorization depends on the nature of the computations and the underlying hardware. Additionally, the Vector API requires careful consideration of data alignment and vectorization-friendly algorithms to fully leverage its capabilities.
Compatibility and Adoption
As with any new feature, compatibility and adoption are key factors to consider. The Vector API is an incubator module in JDK 16, signaling that it's still under development and subject to change. While developers can start experimenting with the Vector API in JDK 16, it's essential to monitor its progress and updates as Java evolves. In terms of adoption, the Vector API provides a compelling option for developers seeking to optimize numerical computations in Java, especially in domains where performance is critical.
The Closing Argument
In conclusion, JDK 16's Vector API introduces a powerful tool for unlocking speed in computation-intensive applications. By providing low-level APIs for vector operations, the Vector API empowers developers to leverage modern CPU hardware and achieve significant performance gains. While the Vector API offers promising opportunities for optimizing numerical computations, it's important to consider its potential limitations and the evolving nature of incubator modules. As Java continues to evolve, the Vector API stands as a testament to the language's commitment to enhancing performance and staying relevant in the modern computing landscape.
Incorporating the Vector API into performance-critical applications can pave the way for accelerated computations and enhanced efficiency, ultimately contributing to the continuous evolution of Java as a high-performance language.
For further information on JDK 16's Vector API, consider referring to JEP 338: Vector API (Incubator) and the official JDK 16 documentation.