Master DB Unit Testing: Overcoming JSON Challenges
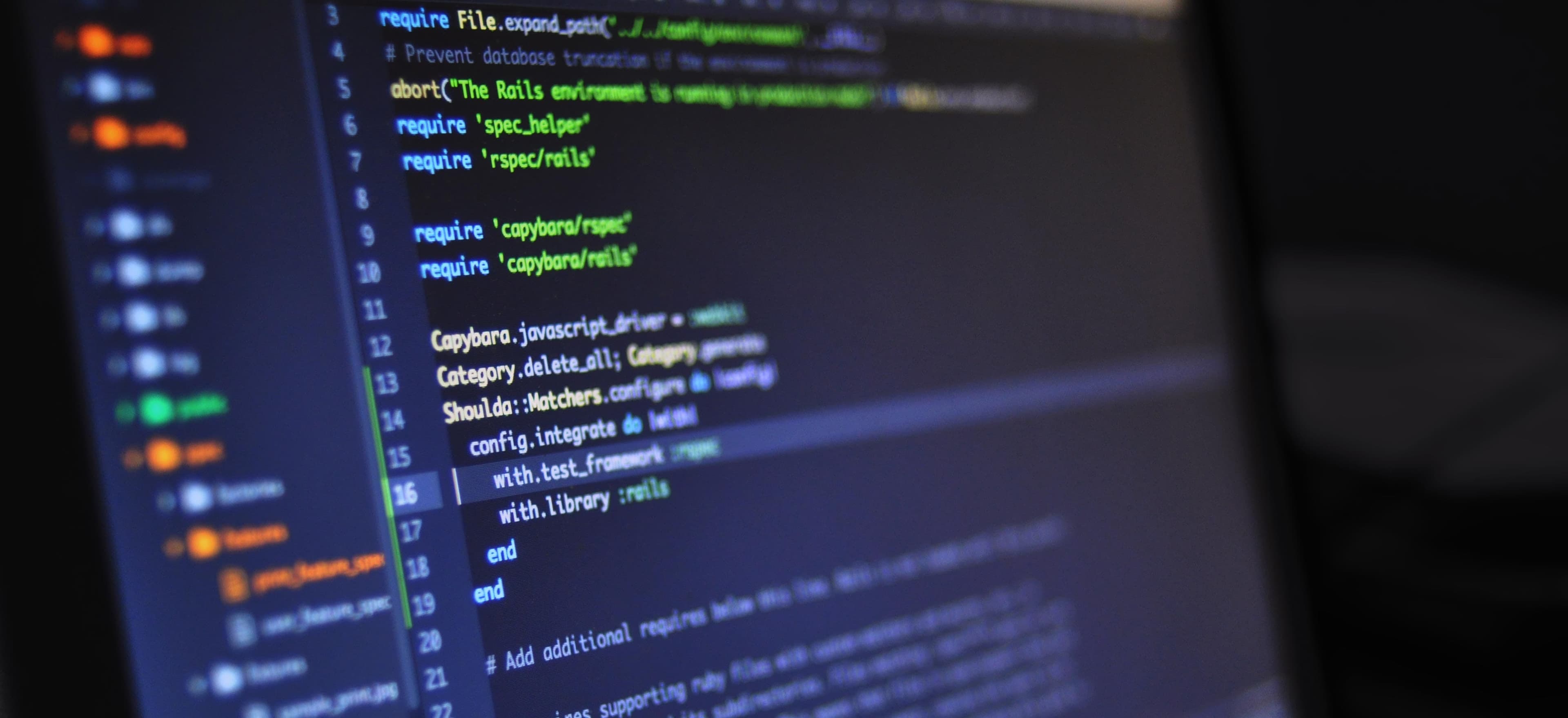
- Published on
Master DB Unit Testing: Overcoming JSON Challenges
In the realm of software development, database unit testing holds undeniable significance. It ensures the reliability and robustness of the database, thereby contributing to the overall quality of the software system. However, when it comes to adopting a comprehensive approach to database unit testing, the challenges associated with testing JSON data cannot be overlooked.
Understanding the JSON Challenge
JSON and Databases
JSON (JavaScript Object Notation) has become a popular format for representing and exchanging data. In modern web development, it is widely utilized for storing data in databases, especially in NoSQL databases like MongoDB.
Structure Complexity
JSON structures can vary significantly in complexity, from simple key-value pairs to nested arrays and objects. Testing the interactions of these structures with the database requires a meticulous approach to ensure accurate and reliable results.
Data Transformation
During database operations, data is often transformed, modified, or processed. Testing the integrity and consistency of JSON data throughout these operations poses a unique set of challenges.
Overcoming JSON Testing Challenges in Database Unit Testing
To address the complexities of testing JSON data in database unit testing, developers and testers can leverage various strategies and tools. Let’s explore some effective approaches to overcome the JSON challenge.
1. Utilize Mocking Frameworks
Mocking frameworks, such as Mockito for Java, can be employed to simulate JSON data interactions within the database. These frameworks enable the creation of mock objects that mimic the behavior of real JSON data, facilitating comprehensive unit testing.
// Example of using Mockito to mock JSON data interactions
@Test
public void testJsonDataInteraction() {
// Create a mock JSON object
JsonObject mockJsonObject = Mockito.mock(JsonObject.class);
// Define the behavior of the mock JSON object
when(mockJsonObject.getString("key")).thenReturn("value");
// Perform database operation with the mock JSON object
// Assert the expected results
}
In this example, Mockito is used to create a mock JSON object and define its behavior, allowing for thorough testing of database interactions.
2. Leverage JSON Schema Validation
JSON schema validation plays a crucial role in ensuring the structural conformity of JSON data within the database. By defining and validating schemas, developers can verify the correctness of JSON structures, thereby fortifying database unit testing.
// Example of JSON schema validation using a library like json-schema-validator
public boolean validateJsonWithSchema(JsonObject data, String schema) {
// Validate the JSON data against the specified schema
// Return true if the data conforms to the schema, false otherwise
}
By integrating JSON schema validation into the testing process, developers can ascertain the consistency and correctness of JSON data stored in the database.
3. Comprehensive Edge Case Testing
Given the diverse nature of JSON data, comprehensive edge case testing is indispensable. By systematically testing boundary scenarios, nested structures, and corner cases, developers can uncover potential vulnerabilities and anomalies in JSON data handling.
// Example of edge case testing for JSON data interactions
@Test
public void testJsonEdgeCases() {
// Test scenarios with nested JSON structures
// Test boundary cases for JSON array operations
// Evaluate the behavior of JSON data during complex database operations
}
Thoroughly testing edge cases provides valuable insights into the robustness and resilience of JSON data interactions within the database.
Key Takeaways
In the domain of database unit testing, effectively overcoming the challenges associated with JSON data is paramount. By embracing advanced testing strategies, leveraging tools like mocking frameworks and JSON schema validation, and conducting meticulous edge case testing, developers and testers can master the art of database unit testing in the JSON era. Embracing the complexities of JSON data and implementing robust testing methodologies ultimately leads to the establishment of a dependable and resilient database infrastructure.
In the fast-evolving landscape of software development, staying abreast of best practices and innovative techniques for database unit testing is pivotal. Embracing the complexities of JSON data and mastering the intricacies of testing it in the context of database operations strengthens the foundation of software systems, assuring their reliability and durability.
As the technological landscape continues to advance, the realm of database unit testing will undoubtedly witness further innovations and refinements, with a steadfast focus on conquering the challenges posed by contemporary data formats such as JSON.
To delve deeper into the realm of database unit testing and enhance your expertise, explore the in-depth insights provided in this comprehensive guide to unit testing in Java. Additionally, gaining a thorough understanding of JSON schema validation will further augment your proficiency in testing JSON data within the database.
Mastering the art of database unit testing in the era of JSON is no small feat. Embracing the intricacies and challenges posed by JSON data empowers developers and testers to fortify the integrity and reliability of database operations, manifesting in robust and resilient software systems.
Checkout our other articles