Simplifying Java Apps: Master Google Cloud Structured Logging
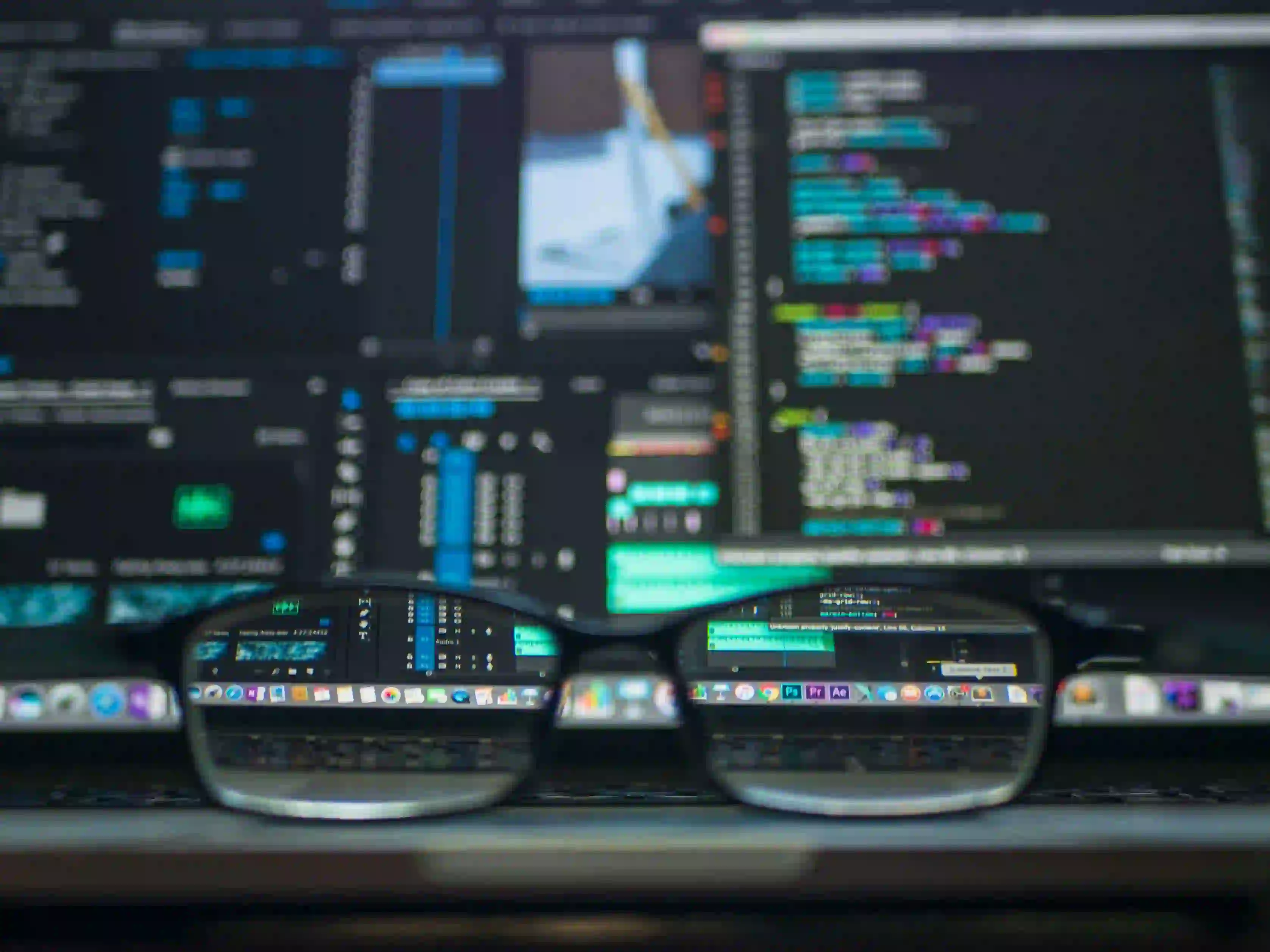
Simplifying Java Apps with Google Cloud Structured Logging
In the world of Java programming, logging is an indispensable tool for understanding the behavior of applications. It provides insight into the runtime flow of an application, helps in debugging, and more. However, managing and analyzing traditional log files can become cumbersome and inefficient as the application scales. This is where structured logging comes into play.
Structured logging enables developers to log data in a structured format, making it easier to search, analyze, and derive insights from the logs. In this article, we will explore how to simplify Java applications using structured logging with Google Cloud's logging service. We will leverage the powerful features of Google Cloud to enhance our logging capabilities and gain deeper insights into our Java applications.
What is Structured Logging?
Traditional logging typically involves writing unstructured text messages to log files. While this approach is straightforward, it has limitations when it comes to analysis and searching. Structured logging, on the other hand, involves logging data in a structured format, such as JSON or key-value pairs. This structured approach allows for better organization, querying, and analysis of log data.
Why Use Structured Logging with Google Cloud?
Google Cloud provides a robust logging service that allows developers to centralize, search, and analyze log data from various sources, including Java applications. By using Google Cloud's structured logging, developers can take advantage of advanced filtering, monitoring, and analysis capabilities offered by the platform. This can lead to better insights, easier troubleshooting, and improved overall application reliability.
Now, let's dive into how we can integrate structured logging into our Java applications and leverage Google Cloud's logging service.
Setting Up Google Cloud Logging
Before we can start logging our Java application data to Google Cloud, we need to set up a project on Google Cloud Platform and enable the Cloud Logging API. Once the API is enabled, we can use the Google Cloud Java Client Libraries to interact with the logging service programmatically.
// Create a Cloud Logging client
Logging logging = LoggingOptions.getDefaultInstance().getService();
// Create a log entry
LogEntry logEntry = LogEntry.newBuilder(
// Define log resource (e.g., the name of the application)
MonitoredResource.newBuilder("global").addLabel("project_id", "your-project-id").build(),
// Define log payload (e.g., structured data in JSON format)
StructuredPayload.newBuilder(ImmutableMap.of("message", "Structured log message")).build())
.setLogName("my-log")
.build();
// Write the log entry
logging.write(Collections.singleton(logEntry));
In the above code snippet, we create a Cloud Logging client using the Java Client Libraries. We then define a log entry with a log resource (e.g., the name of the application) and a log payload containing structured data in JSON format. Finally, we write the log entry to the Cloud Logging service.
Enhancing Java Logging with Structured Data
Now that we have set up Google Cloud Logging, let's see how we can enhance our Java application's logging by incorporating structured data. By logging structured data, we can provide additional context and information that can be invaluable during analysis and troubleshooting.
public class OrderService {
private static final Logger logger = Logger.getLogger(OrderService.class.getName());
public void processOrder(Order order) {
// ... process the order
// Log structured data
Map<String, Object> logData = new HashMap<>();
logData.put("orderId", order.getId());
logData.put("orderStatus", order.getStatus());
logData.put("orderAmount", order.getAmount());
logger.log(Level.INFO, "Order processed", logData);
}
}
In the above example, we have a simplified OrderService
class that processes orders. Instead of logging a plain text message, we log structured data that includes the order ID, status, and amount. This structured approach provides a richer context for each log message, making it easier to understand and analyze the log data.
Leveraging Advanced Logging Features
Google Cloud Logging offers a range of advanced features that can enhance our logging experience. For example, we can use log severity levels to indicate the importance of log messages, create custom logs based on specific criteria, and set up log sinks to export log data to external systems for further analysis.
// Log with severity levels
logger.log(Level.WARNING, "Potential issue with order", logData);
logger.log(Level.SEVERE, "Failed to process order", logData);
// Create custom logs
logger.log(Level.INFO, "Order processed", logData, new CustomLogRecord("custom-log-name"));
// Set up log sinks
// (See https://cloud.google.com/logging/docs/reference/tools/gcloud-logging for gcloud command examples)
By leveraging these advanced features, we can greatly improve the manageability and usability of our log data, making it easier to identify and respond to critical events in our Java applications.
Monitoring and Analyzing Log Data
Once our Java application is integrated with Google Cloud Logging and structured data is being logged, we can use Google Cloud's powerful monitoring and analysis tools to gain insights from the log data. Cloud Logging provides a user-friendly interface for searching and filtering log entries, creating metrics and alerts based on log data, and exporting logs for further analysis.
By utilizing these capabilities, we can gain visibility into the behavior of our Java applications, identify patterns, diagnose issues, and make informed decisions to improve application performance and reliability.
Final Thoughts
In this article, we have explored how to simplify Java applications by leveraging structured logging with Google Cloud's logging service. By adopting structured logging, we can enhance the organization, analysis, and usability of log data, leading to improved debugging, monitoring, and troubleshooting capabilities.
Integrating Java applications with Google Cloud Logging opens up a world of possibilities for managing and gaining insights from log data. By harnessing the power of structured logging and Google Cloud's advanced features, developers can take their logging capabilities to the next level and ensure the reliability and performance of their Java applications.
So, next time you're working on a Java application, consider incorporating structured logging with Google Cloud to streamline your logging process and unlock the full potential of your log data.