Quarkus Quirks: Mastering Non-Standard CDI Features
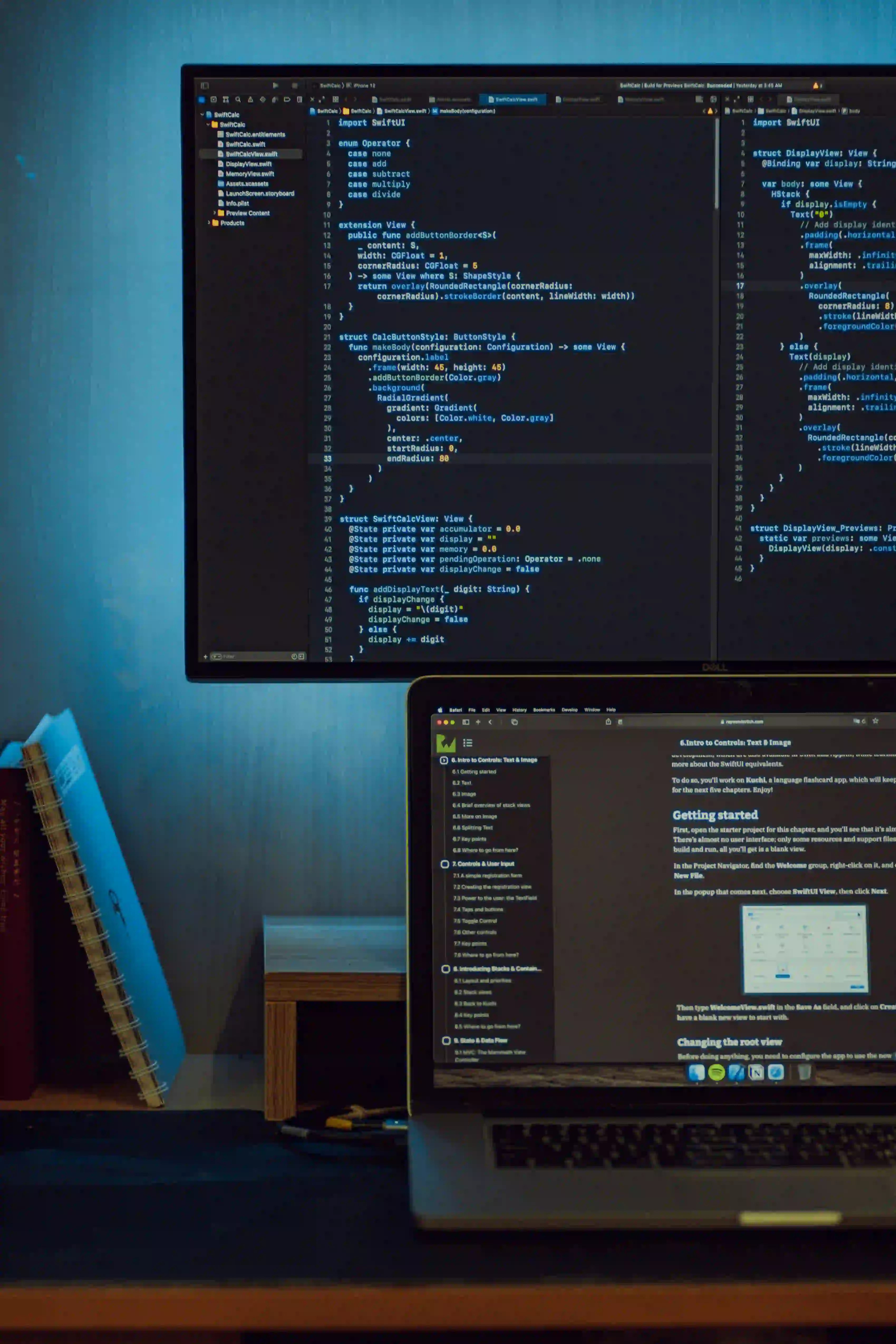
Quarkus Quirks: Mastering Non-Standard CDI Features
Quarkus is a modern, innovative framework tailor-made for Java virtual machine (JVM) developers who want to craft high-performance, low-memory footprint applications. With its vast array of features ranging from live coding, easy testing, and seamless deployment, Quarkus has become a go-to choice for Java developers. In this blog post, we will delve into non-standard Contexts and Dependency Injection (CDI) features in Quarkus and understand how to leverage them to build robust applications.
What Makes Quarkus CDI Special?
The Contexts and Dependency Injection (CDI) specification in Quarkus provides a powerful and flexible way to manage the lifecycle of objects and their dependencies. Quarkus embraces the CDI specification and takes it a step further by offering non-standard features that enhance the developer experience.
@RegisterExtension: Turbocharging CDI Extensions
In Quarkus, the @RegisterExtension
annotation serves as a powerful tool for turbocharging CDI extensions. It allows developers to easily integrate third-party CDI extensions and customize their behavior without the need for verbose configuration. Let's take a look at an example to understand the power of @RegisterExtension
.
@ApplicationScoped
public class MyCDIExtension implements Extension {
public void beforeBeanDiscovery(@Observes BeforeBeanDiscovery bbd, BeanManager bm) {
// Custom logic to manipulate the bean discovery process
}
}
By annotating the MyCDIExtension
class with @RegisterExtension
, Quarkus automatically registers it as a CDI extension, making the custom logic defined in the class effective without any additional configuration.
Integrating Non-Standard Scopes: @RequestScoped
Quarkus introduces non-standard scopes to augment the existing set of CDI scopes. One such scope is the @RequestScoped
, which is specifically tailored for handling HTTP requests in a stateful manner, resembling the behavior of traditional web applications. Let's see how we can integrate @RequestScoped
in a Quarkus application.
@RequestScoped
public class RequestScopedBean {
// Bean logic specific to the scope of an HTTP request
}
With @RequestScoped
, Quarkus enables developers to manage the lifecycle of beans at the granularity of an HTTP request, providing a cohesive and intuitive way to handle request-specific data.
Flexible Dependency Injection with @ConfigProperty
Quarkus simplifies the process of injecting configuration properties through the @ConfigProperty
annotation. This non-standard CDI feature allows for seamless integration of externalized configuration properties into the application. Here's an example of how to use @ConfigProperty
.
@ApplicationScoped
public class MyConfigurableBean {
@Inject
@ConfigProperty(name = "app.message")
String message;
public void displayMessage() {
System.out.println(message);
}
}
By leveraging @ConfigProperty
, developers can effortlessly inject configuration properties from various sources such as environment variables, system properties, and configuration files, making the application highly configurable and adaptable.
Eventing in Quarkus: Observed Events
Quarkus extends the standard CDI eventing model by introducing the concept of observed events. This allows beans to observe specific events and react accordingly, providing a decoupled and modular approach to event-driven programming. Let's explore how observed events work in Quarkus.
@ApplicationScoped
public class EventHandlerBean {
public void onOrderPlaced(@Observes OrderPlacedEvent event) {
// Logic to handle the order placed event
}
}
In this example, EventHandlerBean
observes the OrderPlacedEvent
and reacts to it without explicit event firing logic. This streamlined approach simplifies the event-driven programming model and promotes loose coupling between components.
Key Takeaways
Quarkus’ non-standard CDI features offer Java developers a plethora of tools to streamline the development of modern, high-performance applications. By embracing these advanced CDI capabilities, developers can leverage Quarkus’ full potential and push the boundaries of JVM-based application development.
In this post, we've explored various non-standard CDI features in Quarkus, ranging from @RegisterExtension for turbocharging CDI extensions and @RequestScoped for handling HTTP requests to @ConfigProperty for seamless configuration property injection and observed events for event-driven programming. By mastering these Quarkus quirks, developers can elevate their Java applications to new heights of performance and flexibility.
If you're keen on delving deeper into Quarkus CDI and its non-standard features, make sure to explore the official Quarkus documentation for comprehensive insights.
Unleash the true potential of CDI in Quarkus and revolutionize your Java development experience!