Overcoming Interface Default Method Issues in Spock & Kotlin
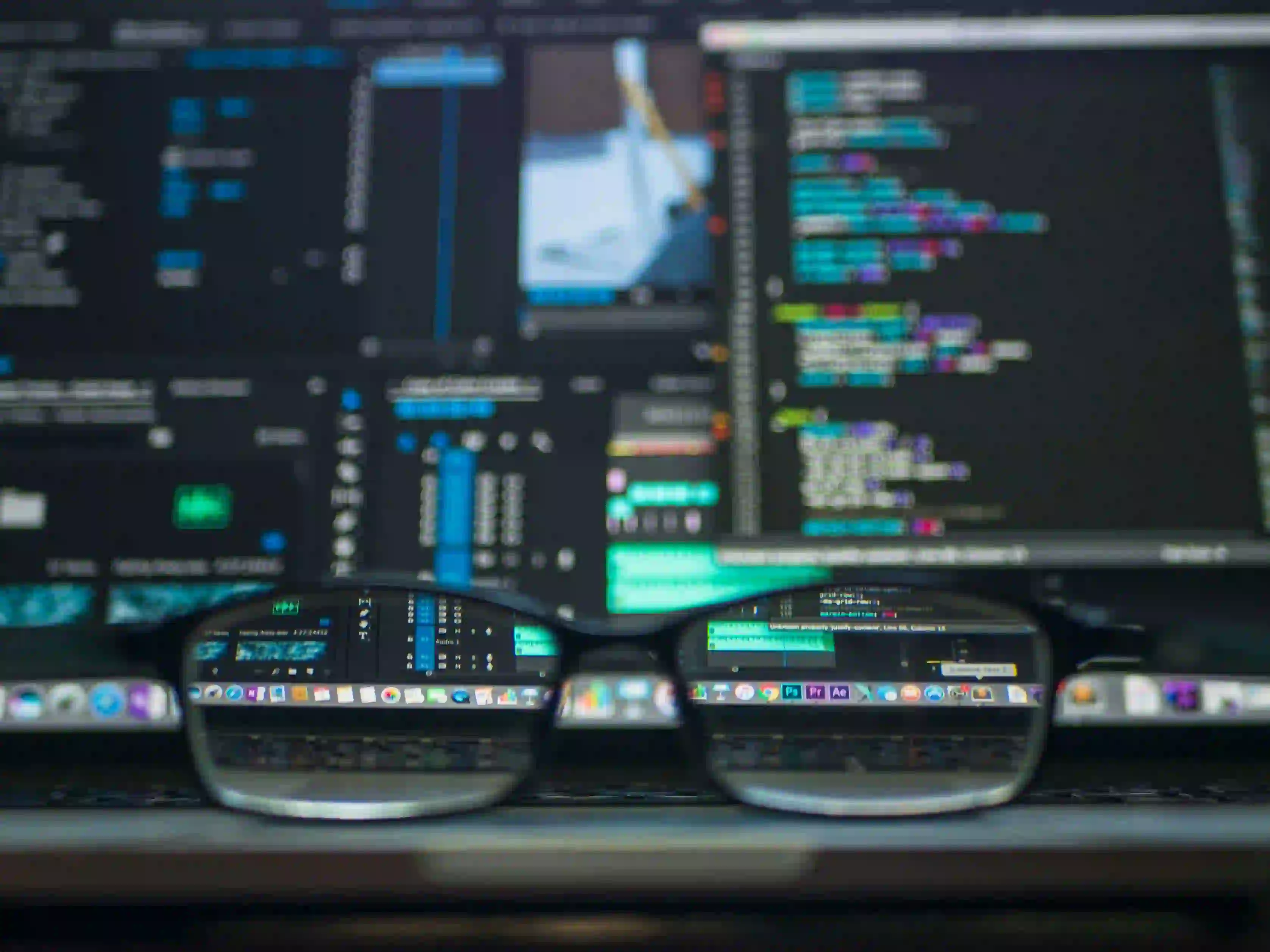
Overcoming Interface Default Method Issues in Spock & Kotlin
Interfaces in Java have come a long way since their introduction. With the introduction of default methods in Java 8, interfaces gained the ability to provide concrete implementations for their methods. This feature has brought a lot of flexibility to the language, allowing interfaces to evolve without breaking the classes that implement them. However, as with any feature, default methods come with their own set of challenges, particularly when working with testing frameworks such as Spock in the context of Kotlin.
In this article, we will explore the issues that can arise when using default methods in interfaces, particularly in the context of Spock tests, and how to overcome them when working with Kotlin.
The Problem
Consider the following scenario: You have an interface with a default method that you want to test using the Spock testing framework. The implementation of this interface is in Kotlin. When you write a Spock test for the class that implements this interface, you encounter unexpected behavior due to default methods in the interface.
The Code
Let's start by defining the problematic interface and its implementation in Kotlin:
interface Greeter {
fun greet(): String {
return "Hello, World!"
}
}
class SimpleGreeter : Greeter
In this code, Greeter
is an interface with a default method greet
, and SimpleGreeter
is a class that implements this interface.
The Test
Now, let's attempt to write a simple Spock test for the SimpleGreeter
class:
class SimpleGreeterSpec
extends spock.lang.Specification {
def "Test greet method"() {
given:
def greeter = new SimpleGreeter()
expect:
greeter.greet() == "Hello, World!"
}
}
Surprisingly, this test fails, even though the greet
method simply returns "Hello, World!". This unexpected behavior is due to Spock's interaction with default methods in Kotlin interfaces.
The Solution
Using Java Interface
One way to overcome this issue is to define the interface in Java, which is less affected by the default method issues:
public interface Greeter {
default String greet() {
return "Hello, World!";
}
}
By using a Java interface with default methods rather than a Kotlin interface, we can often avoid the unexpected behavior caused by Spock's handling of default methods.
Key Takeaways
Default methods in interfaces provide a lot of flexibility in Java, but they can also introduce unexpected behavior, especially in the context of testing frameworks like Spock. When working with Kotlin implementations of interfaces, it's important to be mindful of these potential issues and consider using Java interfaces with default methods as a workaround.
By understanding the challenges associated with default methods in interfaces and considering alternative approaches, such as using Java interfaces in Kotlin, developers can ensure the reliability and correctness of their code, particularly when working with testing frameworks like Spock.
In conclusion, while default methods offer powerful capabilities in Java interfaces, their usage in conjunction with testing frameworks requires careful consideration and potential workarounds to ensure smooth interactions between interfaces and their implementations in Kotlin.