Mastering Android: Fix Common SwipeRefreshLayout Issues
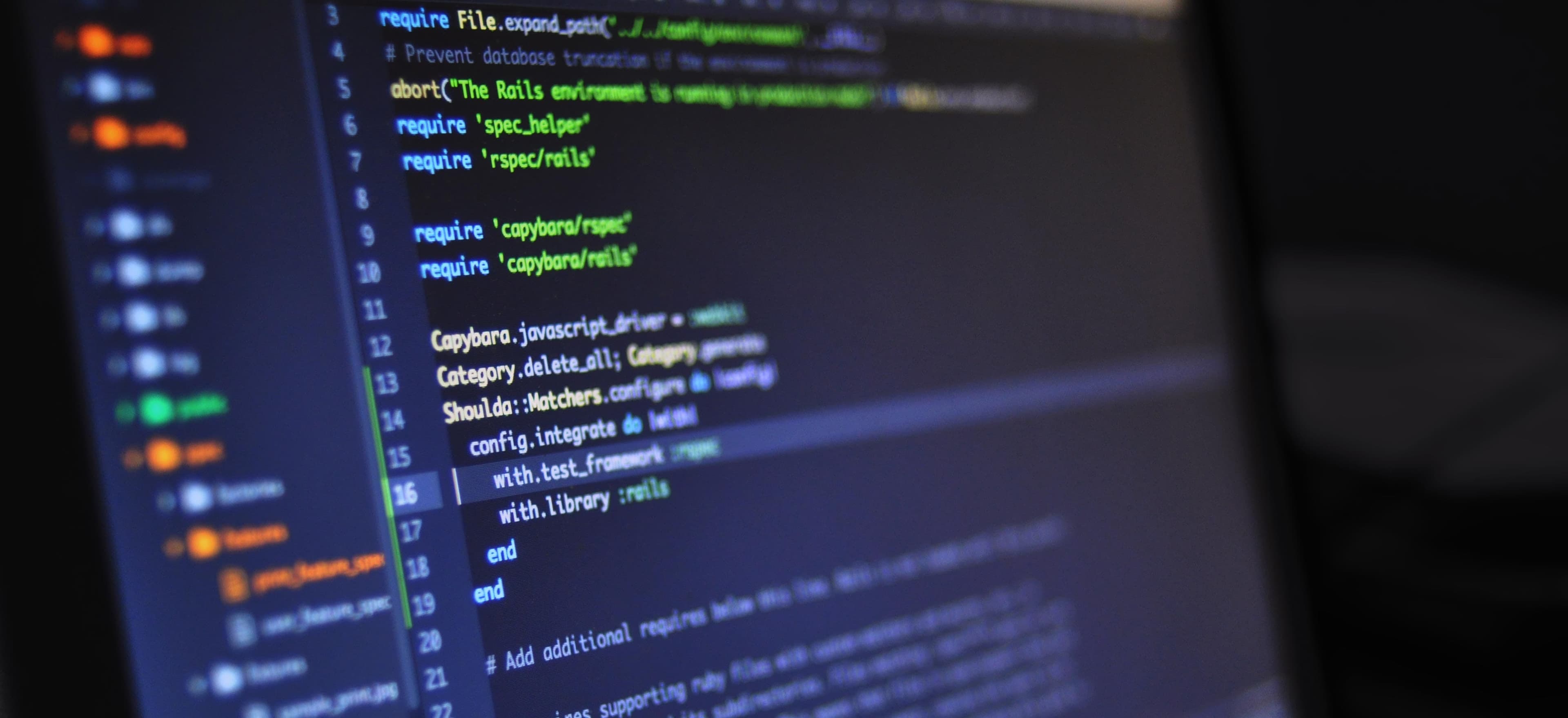
- Published on
Mastering Android: Fix Common SwipeRefreshLayout
Issues
When it comes to building modern and engaging Android applications, implementing pull-to-refresh functionality is a crucial aspect of enhancing user experience. SwipeRefreshLayout
is a popular component in Android that provides the swipe-to-refresh gesture, allowing users to refresh the contents of a view via a vertical swipe gesture. While SwipeRefreshLayout
is a powerful tool, it can come with its own set of issues that developers may encounter. In this guide, we'll explore some common problems with SwipeRefreshLayout
and provide effective solutions to address them.
Understanding SwipeRefreshLayout
Before delving into the common issues and their solutions, let's briefly understand the basics of SwipeRefreshLayout
. This built-in Android component provides a standard gesture for refreshing the contents of a view. It can wrap a single view or a view group, enabling the pull-to-refresh functionality for the enclosed content. The primary way SwipeRefreshLayout
is used is by adding a ListView
, RecyclerView
, or ScrollView
as its child, allowing users to trigger a refresh by swiping down on the view.
Initializing SwipeRefreshLayout
To implement SwipeRefreshLayout
, it's crucial to have a solid understanding of the initialization process. Here's a simple example of setting up SwipeRefreshLayout
in an Android Activity:
SwipeRefreshLayout swipeRefreshLayout = findViewById(R.id.swipe_refresh_layout);
swipeRefreshLayout.setOnRefreshListener(() -> {
// Perform actions when the user triggers a refresh
// This is where you would typically call an API or perform data loading
// Once the refresh is complete, make sure to call setRefreshing(false)
});
In this code snippet, we initialize the SwipeRefreshLayout
by finding its corresponding view in the layout file using findViewById
. We then set an OnRefreshListener
to listen for the swipe-to-refresh gesture. Inside the listener, you would typically perform data loading or API calls and stop the refreshing indicator when the task is completed.
Common SwipeRefreshLayout
Issues and Solutions
Issue 1: Multiple Refresh Triggers
One common issue that developers face with SwipeRefreshLayout
is multiple refresh triggers. This occurs when the refresh indicator is not properly reset after the refresh action is performed.
Solution:
To prevent multiple refresh triggers, ensure that you call setRefreshing(false)
once the refresh action is completed. This stops the refreshing indicator and prevents further refresh triggers until the user swipes again.
Issue 2: Nested Scroll Conflicts
Another prevalent issue arises when using SwipeRefreshLayout
with scrollable views such as RecyclerView
or NestedScrollView
. In some cases, nested scroll conflicts may cause the refresh functionality to behave unexpectedly or not work at all.
Solution:
To resolve nested scroll conflicts, you can set the nested scrolling enabled property to false
on the nested scrollable view. For example, in the case of a RecyclerView
, you can use the following code to disable nested scrolling:
recyclerView.setNestedScrollingEnabled(false);
By disabling nested scrolling, you can ensure that the swipe-to-refresh gesture functions seamlessly without any conflicts with the nested scrollable view.
Issue 3: Incorrect Colors or Styles
Developers often encounter issues related to the visual appearance of SwipeRefreshLayout
, such as incorrect colors or styles not being applied as expected.
Solution:
To address color or style-related issues, you should customize the SwipeRefreshLayout
by defining custom colors for the progress spinner or background. You can achieve this in the layout XML file by specifying the app:progressBackgroundColor
and app:progressColor
attributes to customize the appearance according to your app's theme.
<androidx.swiperefreshlayout.widget.SwipeRefreshLayout
android:id="@+id/swipe_refresh_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:progressBackgroundColor="@color/custom_background_color"
app:progressColor="@color/custom_progress_color">
<!-- Add your scrollable view here -->
</androidx.swiperefreshlayout.widget.SwipeRefreshLayout>
By explicitly defining the progress background color and progress color, you can ensure that SwipeRefreshLayout
adheres to your app's design guidelines and maintains a cohesive visual appearance.
To Wrap Things Up
In conclusion, SwipeRefreshLayout
is a powerful tool that enhances the user experience by enabling pull-to-refresh functionality in Android applications. By understanding common issues and implementing the provided solutions, developers can ensure a seamless and reliable user experience when integrating SwipeRefreshLayout
into their apps.
By mastering the nuances of SwipeRefreshLayout
and effectively addressing any issues that may arise, developers can create polished and user-friendly Android applications with seamless pull-to-refresh functionality.
It's essential to stay updated with the latest Android development best practices and continuously refine your skills in crafting exceptional user experiences through mastering essential Android components and addressing common issues that may arise.
With these solutions and best practices at your disposal, you can confidently leverage SwipeRefreshLayout
to its full potential, delivering a refined and responsive user experience in your Android applications.
Now that you're equipped with the knowledge to tackle common SwipeRefreshLayout
issues, it's time to elevate your Android development skills and ensure a seamless pull-to-refresh experience for your app users. Happy coding!
Remember, mastering Android development is an ongoing journey, and staying informed about new best practices and solutions is key to delivering exceptional applications.
By addressing common SwipeRefreshLayout
issues and providing effective solutions, this blog post aims to help developers enhance the user experience of their Android applications. By explaining the basics of SwipeRefreshLayout
and offering practical solutions to common problems, developers can gain a deeper understanding of this essential Android component. If you'd like to learn more about Android development, consider exploring the official Android Developer documentation.