Micro vs Nano Services: Navigating the Tiny Divide
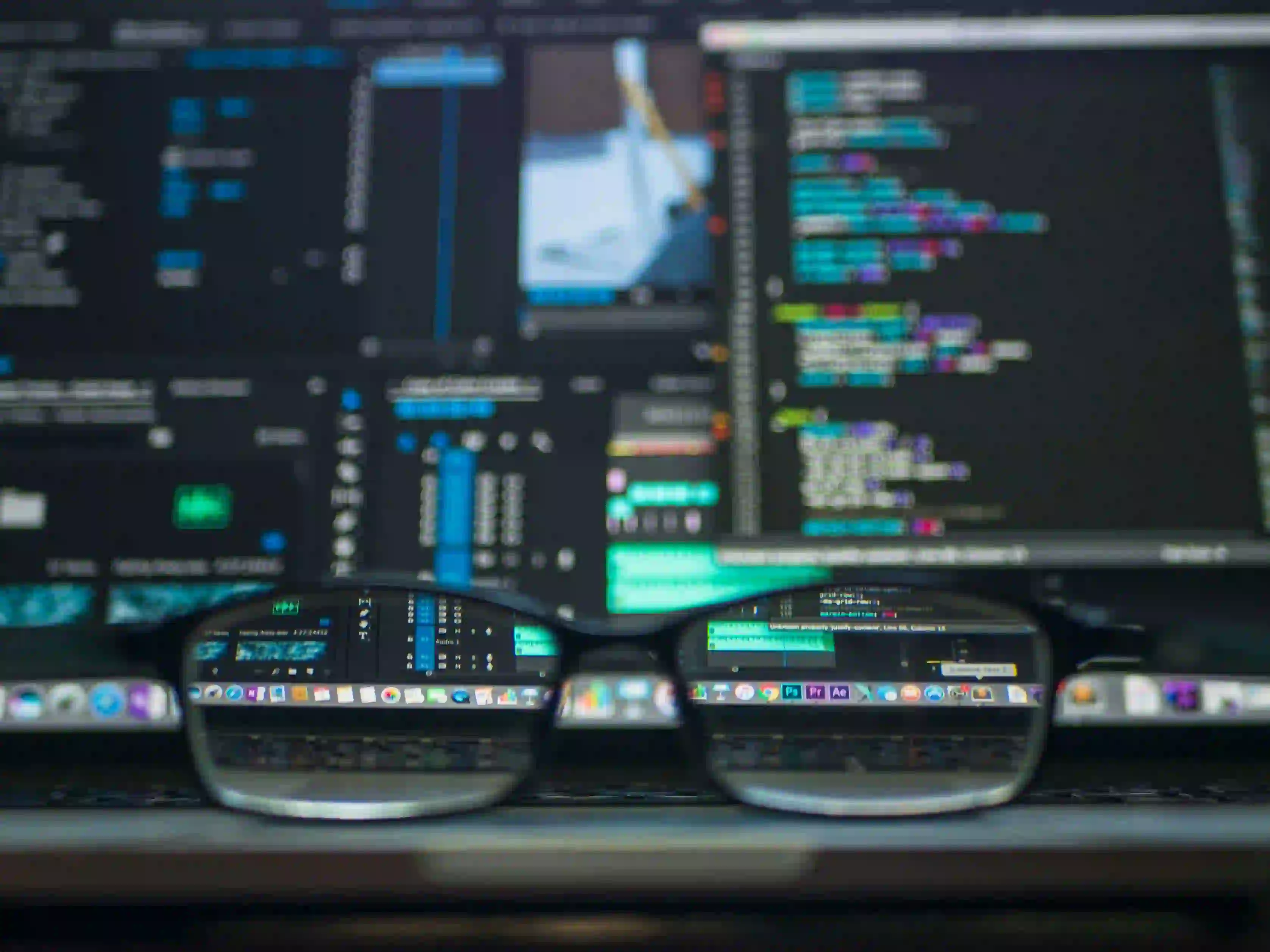
Micro vs Nano Services: Navigating the Tiny Divide
In today's fast-paced and demanding development environment, the concept of microservices has gained significant traction, as it enables agility, scalability, and an independent deployment model. However, with the emergence of nano services, developers are pondering the trade-offs and benefits of these extremely lightweight components. This article delves into the nuances of micro and nano services, their impact on the overall architecture, and the considerations to navigate the tiny yet crucial divide.
Understanding Microservices
Microservices are an architectural style that structures an application as a collection of loosely coupled services. Each service is self-contained, can be developed and deployed independently, and communicates through well-defined APIs. This results in a scalable, flexible, and easier-to-maintain system, where changes can be localized, and failures in one service do not cascade across the entire application.
Java, with its robust ecosystem and tools, has been a popular choice for building microservices. Frameworks such as Spring Boot provide a convenient way to create standalone, production-grade Spring-based applications.
Code Demonstration: Creating a Simple Microservice with Spring Boot
@SpringBootApplication
public class UserServiceApplication {
public static void main(String[] args) {
SpringApplication.run(UserServiceApplication.class, args);
}
}
@RestController
@RequestMapping("/users")
public class UserController {
@GetMapping("/{id}")
public User getUserById(@PathVariable Long id) {
// Retrieve user details from database and return
}
@PostMapping
public ResponseEntity<String> createUser(@RequestBody User user) {
// Create user in the database and return success message
}
@DeleteMapping("/{id}")
public ResponseEntity<String> deleteUser(@PathVariable Long id) {
// Delete user from database and return success message
}
}
In the above code snippet, we showcase a simple User Service created with Spring Boot. The UserController
demonstrates the endpoints for retrieving, creating, and deleting a user. The standalone nature of this service exemplifies the principles of microservices.
Introducing Nano Services
While microservices have been widely adopted, the concept of nano services takes service granularity to the extreme. Nano services advocate breaking down functionalities into their smallest possible components, often at the method or function level. This level of granularity enables fine-grained scalability and the potential for reusability of the smallest units of code.
The Java Perspective
In the Java landscape, the introduction of Quarkus has sparked interest in nano services. Quarkus, with its emphasis on low memory consumption, fast boot time, and efficient execution of microservices, has positioned itself as a formidable player in the nano services arena.
Code Demonstration: Creating a Nano Service with Quarkus
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.PathParam;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("/hello")
public class HelloWorldResource {
@GET
@Path("/{name}")
@Produces(MediaType.TEXT_PLAIN)
public String hello(@PathParam("name") String name) {
return "Hello, " + name;
}
}
In this example, we showcase a nano service created with Quarkus. The HelloWorldResource
contains a single method to greet a person by name. The compactness of this service exemplifies the granularity of nano services.
Navigating the Divide: Micro vs Nano Services
-
Granularity: Microservices strike a balance between being granular enough for independent management and coarse-grained enough for operational simplicity. Nano services, on the other hand, offer an unparalleled level of granularity, opening the door for extreme scalability and targeted optimization.
-
Complexity vs. Efficiency: Microservices introduce operational complexity, such as orchestrating multiple services, managing inter-service communication, and ensuring data consistency. Nano services, while offering efficiency due to their size and focused functionality, may introduce governance challenges and increased operational overhead due to the sheer volume of services.
-
Resource Utilization: Nano services, with their fine-grained nature, have the potential to optimize resource utilization by consuming only the exact resources required for a specific functionality. Microservices, despite being more resource-intensive, provide a balance between resource utilization and operational overhead and are well-suited for larger, more complex functionalities.
-
Deployment Overhead: While microservices exhibit deployment overhead at the service level, nano services further amplify this overhead because of the larger number of services, each requiring individual provisioning, orchestration, and networking.
The Closing Argument
Microservices and nano services each have their merits and trade-offs, and the choice between them depends on the specific business requirements, operational constraints, and the nature of the functionalities at hand. As Java developers navigate the tiny yet crucial divide between micro and nano services, an understanding of the nuances, impacts, and practical considerations will be essential to architecting resilient and efficient systems.
In summary, while microservices continue to dominate the architectural landscape, nano services offer an intriguing proposition for extreme granularity and efficiency. Ultimately, the decision between micro and nano services should be driven by a thorough evaluation of the specific use case, operational challenges, and the long-term maintainability and scalability of the system.
By carefully weighing these factors, developers can effectively leverage the advantages of both paradigms, ensuring that their architectural choices align with the overarching goals of the organization and the demands of modern, agile software development.
Related Links:
- Spring Boot
- Building Microservices with Spring Boot
- Quarkus Framework
- Quarkus - Supersonic Subatomic Java
- Java Microservices: A Happy Meal for Developers?
- Nano Services: The Future of Microservices