Revamping the Joel Test: Key Updates for Modern Dev Teams
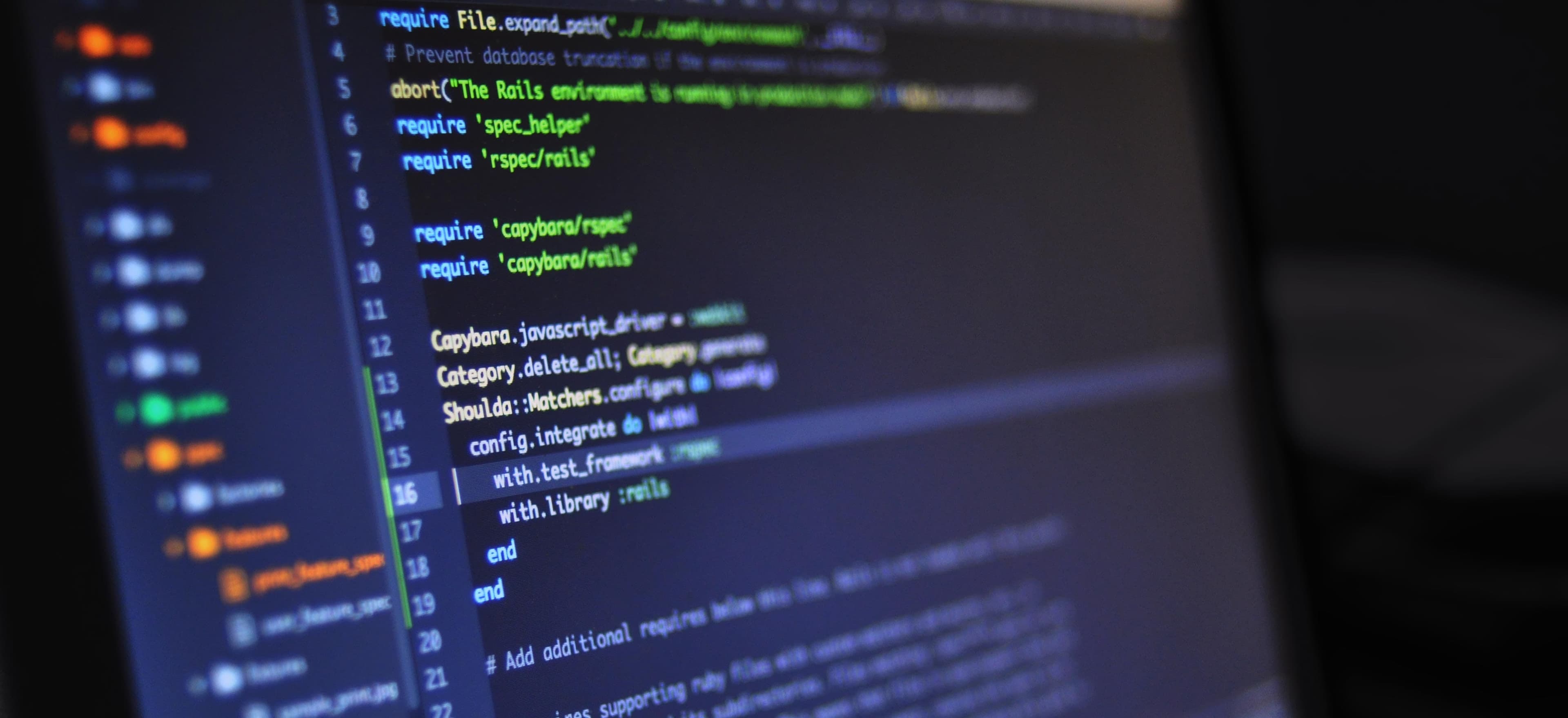
- Published on
Revamping the Joel Test: Key Updates for Modern Dev Teams
The Joel Test is a popular checklist created by Joel Spolsky to assess the quality of a software development team. While the original test was introduced over two decades ago, the landscape of software development has evolved significantly since then. This blog post will explore key updates and additions to the Joel Test to better reflect the needs and challenges of modern development teams.
The Original Joel Test
Before diving into the updates, let's take a quick look at the original Joel Test, which consists of 12 yes-or-no questions aimed at assessing the quality of a software team. The questions cover various aspects of development, including version control, bug tracking, testing, and more.
Here are a few of the original Joel Test questions:
- Do you use source control?
- Can you make a build in one step?
- Do you make daily builds?
- Do you have a bug database?
- Do you fix bugs before writing new code?
While these questions were highly relevant at the time they were formulated, the rapid evolution of technology and best practices in software development necessitates an updated assessment framework.
Updates to the Joel Test for Modern Dev Teams
1. Embracing Continuous Integration/Continuous Delivery (CI/CD)
In today's agile and DevOps-oriented environment, embracing CI/CD practices is crucial. Teams should not only be able to make a build in one step but also have automated pipelines for continuous integration and delivery.
// Example of a simple Jenkinsfile for CI/CD
pipeline {
agent any
stages {
stage('Build') {
steps {
// Your build steps here
}
}
stage('Test') {
steps {
// Automated testing steps
}
}
stage('Deploy') {
steps {
// Deployment steps
}
}
}
}
Why it matters: CI/CD not only automates the software delivery process but also ensures that potential issues are identified and resolved early in the development cycle.
2. Code Review and Pull Request Processes
Incorporating a robust code review process and using pull requests for collaboration and review is fundamental in modern software development.
// Example of a pull request review in GitHub
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Why it matters: Code reviews and pull requests facilitate knowledge sharing, identify potential issues, and improve overall code quality.
3. Infrastructure as Code (IaC) Adoption
With the rise of cloud computing and infrastructure automation, the ability to define and manage infrastructure through code has become essential. Tools like Terraform and AWS CloudFormation are commonly used for IaC.
// Example of Terraform code for provisioning infrastructure
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
}
Why it matters: IaC enables reproducible and consistent infrastructure deployments, reduces manual errors, and promotes agility in managing infrastructure.
4. Containerization and Orchestration Competence
Incorporating containerization with Docker and orchestration with Kubernetes has become a standard practice for modern software applications.
// Example of defining a Dockerfile for a Java application
FROM openjdk:8-jdk-alpine
COPY target/app.jar app.jar
CMD ["java","-jar","app.jar"]
Why it matters: Containerization and orchestration simplify application deployment, enhance scalability, and improve resource utilization.
5. Focus on Security Practices
With the increasing frequency of cybersecurity threats, integrating security practices into the development process is critical. This includes conducting security reviews, performing static/dynamic code analysis, and adhering to secure coding standards.
// Example of a basic input validation for security
public void processInput(String input) {
if (input.matches("[a-zA-Z0-9 ]+")) {
// Process the input
} else {
// Handle invalid input
}
}
Why it matters: Prioritizing security from the early stages of development can prevent vulnerabilities and protect against potential security breaches.
6. Adoption of Agile Methodologies
In today's fast-paced development environment, embracing agile methodologies such as Scrum or Kanban is crucial for improved collaboration, adaptability to change, and timely delivery of high-quality software.
// Example of a user story in a Scrum board
public class UserStory {
String title;
String description;
List<Task> tasks;
// Additional attributes and methods
}
Why it matters: Agile methodologies promote iterative and customer-centric development, enabling teams to respond to changing requirements more effectively.
Final Considerations
As software development practices and technologies continue to evolve, it's essential to periodically reevaluate the assessment criteria for development teams. The updates and additions to the Joel Test outlined in this post reflect the changing landscape of modern software development, emphasizing essential practices and tools that contribute to the success of development teams.
By incorporating these updates into the evaluation process, organizations can better gauge the effectiveness and efficiency of their development efforts in today's dynamic and demanding environment.
Remember, while the original Joel Test served as a valuable benchmark for its time, staying relevant in the ever-changing world of software development requires adaptation and continuous improvement.
For further in-depth understanding of modern Java development practices, check out Java Best Practices, a comprehensive guide to writing clean, efficient, and maintainable Java code.
Checkout our other articles