Mastering Spark: Solving Local Run Design Pattern Dilemmas
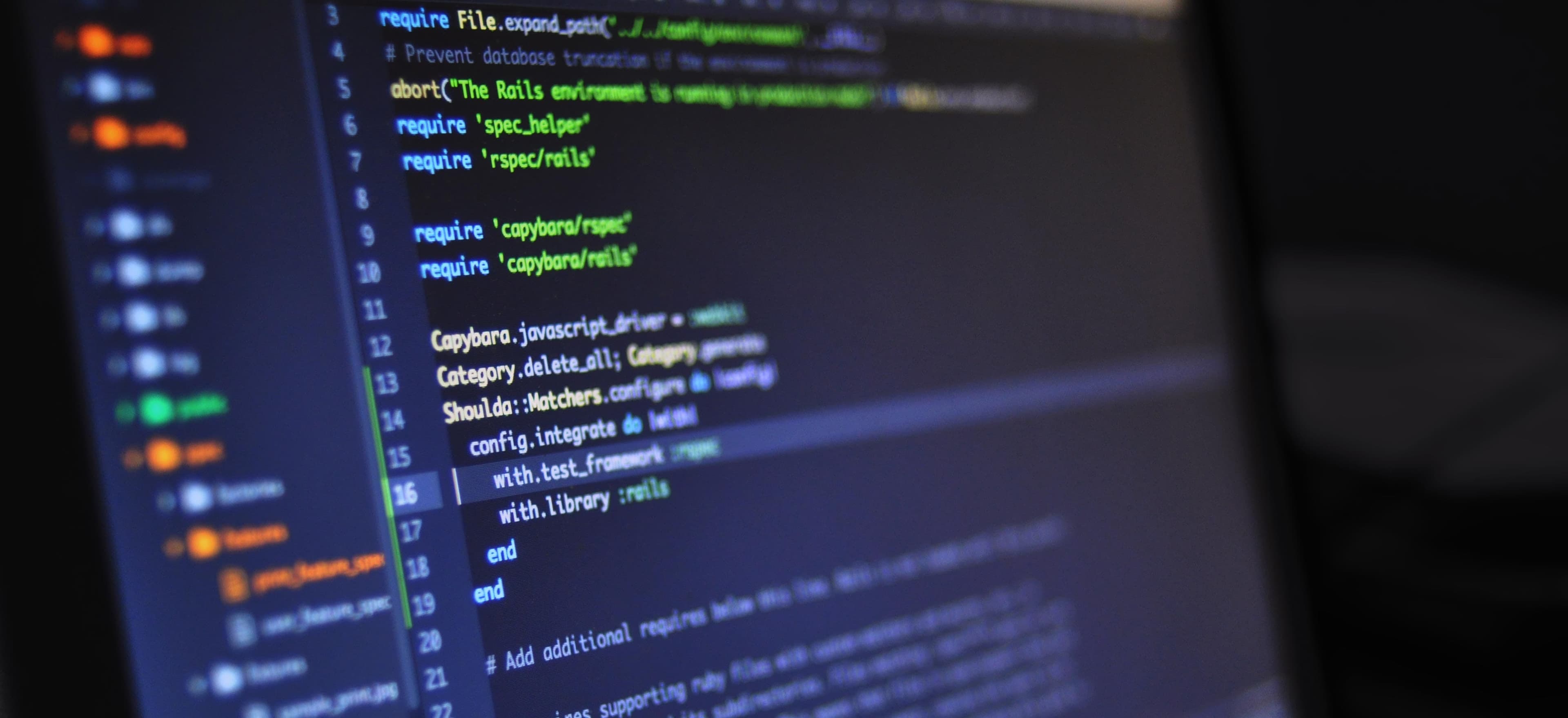
- Published on
Mastering Spark: Solving Local Run Design Pattern Dilemmas
When building applications with Apache Spark, developers often face challenges relating to local run design patterns, which are crucial to ensuring the smooth functioning of larger-scale distributed systems. In this article, we'll explore common design pattern dilemmas when running Spark locally and discuss effective solutions to overcome them.
Understanding Local Run Design Patterns
In a local run scenario, Spark runs on a single machine without leveraging the full potential of distributed computing. However, it's essential to mimic the distributed environment to uncover potential issues early in the development cycle.
Common Dilemmas
-
Data Source Paths: The path to data sources might differ between local and distributed environments, leading to discrepancies in file references and data loading.
-
Resource Management: Local runs require efficient resource management to simulate distributed execution, ensuring optimal performance and stability.
-
Configuration Consistency: Maintaining consistent configuration settings between local and distributed modes is essential for reliability and predictability.
Solution: Leveraging Design Patterns
1. Handling Data Source Paths
import org.apache.spark.sql.SparkSession;
public class LocalRunDesignPatterns {
public static void main(String[] args) {
SparkSession spark = SparkSession.builder()
.appName("LocalRunDesignPatterns")
.config("spark.master", "local[*]") // Simulate distributed environment
.getOrCreate();
String dataPath = "data/sample.json";
// Use absolute or relative path as per local execution
String absolutePath = spark.read().json(dataPath).toString();
}
}
In the code snippet above, we create a Spark session and specify the master URL as "local[*]," mimicking a distributed environment. Then, we handle the data source path by using either an absolute or relative path, ensuring compatibility with both local and distributed executions.
2. Efficient Resource Management
public class LocalRunDesignPatterns {
public static void main(String[] args) {
SparkSession spark = SparkSession.builder()
.appName("LocalRunDesignPatterns")
.config("spark.driver.memory", "4g") // Set driver memory for local run
.getOrCreate();
// Define resource allocation and configurations as needed
}
}
Here, we configure the driver memory explicitly for the local run, ensuring efficient resource allocation and management. This approach enables us to simulate resource constraints similar to those in distributed environments, facilitating early issue detection and resolution.
3. Consistent Configuration Settings
public class LocalRunDesignPatterns {
public static void main(String[] args) {
SparkSession spark = SparkSession.builder()
.appName("LocalRunDesignPatterns")
.config("spark.sql.shuffle.partitions", 5) // Set consistent shuffle partitions
.getOrCreate();
// Maintain consistent configuration settings for local and distributed runs
}
}
By explicitly setting consistent configuration parameters such as shuffle partitions, we ensure that the behavior of our application remains consistent across local and distributed run scenarios. This promotes reliability and predictability, enabling seamless transition between development and production environments.
Key Takeaways
Mastering local run design patterns is essential for constructing robust and scalable Spark applications. By understanding common dilemmas and adopting effective solutions through appropriate design patterns, developers can streamline the development process, minimize issues, and ensure seamless scalability to distributed environments. Embracing these design patterns not only enhances the reliability and performance of Spark applications but also paves the way for efficient debugging and troubleshooting, ultimately leading to a more robust and maintainable codebase.
In summary, mastering the intricacies of local run design patterns in Spark is a critical component in the journey towards becoming a proficient Spark developer. With the right understanding and implementation of design patterns, developers can navigate the complexities of local runs with ease, laying a solid foundation for successful scaling and deployment in distributed environments.
To delve deeper into the world of Spark and explore advanced concepts and best practices, check out the official Apache Spark documentation and Databricks' Spark resources. Happy Sparking!