Overcoming Common Pitfalls with Hibernate Validator in Java SE
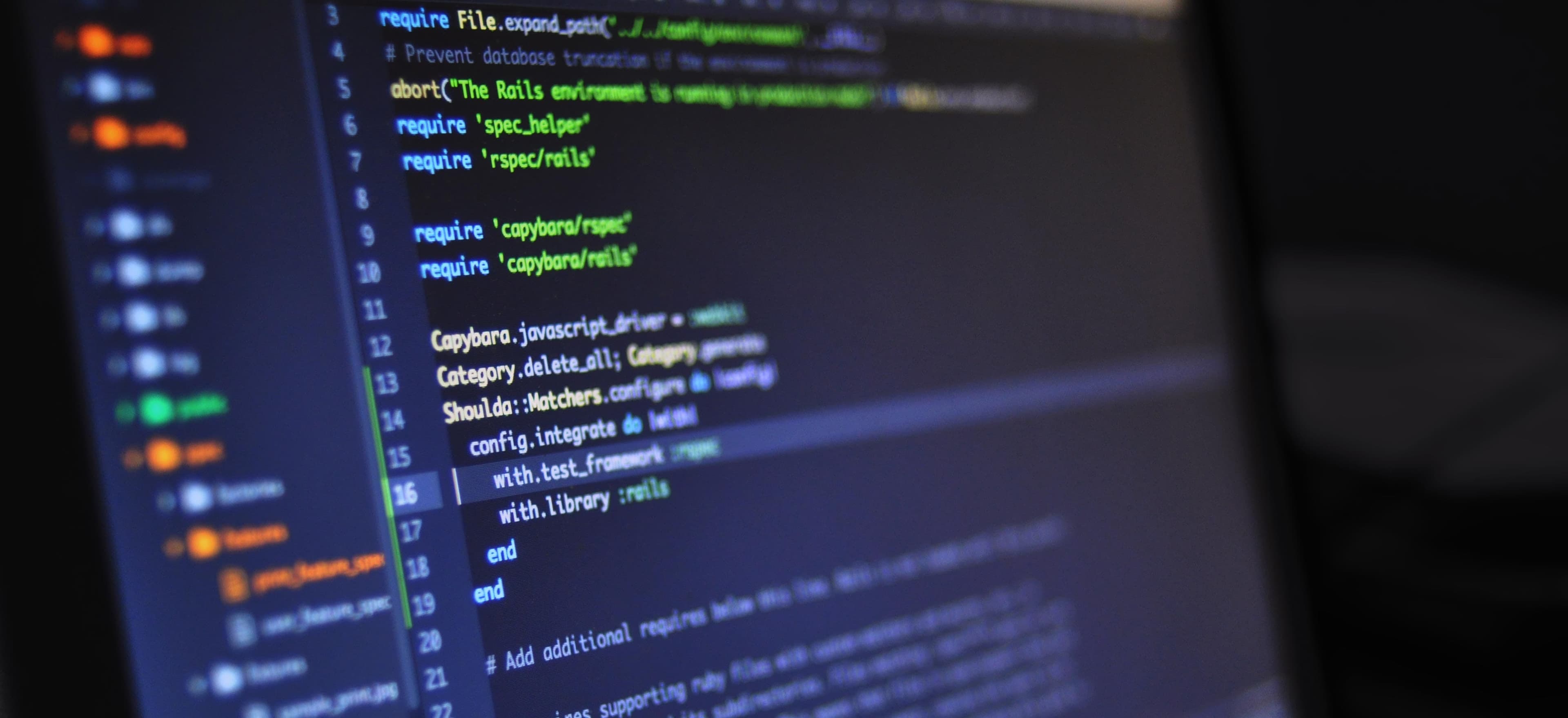
- Published on
Overcoming Common Pitfalls with Hibernate Validator in Java SE
When it comes to building robust and reliable Java applications, input validation is a critical aspect that cannot be overlooked. Hibernate Validator, a popular implementation of the Java Bean Validation (JSR 380) specification, provides a powerful framework for declaring and enforcing validation constraints on domain models. While it offers numerous benefits, developers often encounter common pitfalls when working with Hibernate Validator in Java SE applications. In this article, we will explore these pitfalls and provide solutions to overcome them.
Pitfall 1: Incorrect Configuration
One of the common pitfalls when working with Hibernate Validator is incorrect configuration. It's essential to ensure that the validation is properly configured in the application. The configuration typically involves setting up the ValidatorFactory and Validator instances, and it's crucial to initialize them correctly.
Solution:
// Correct way to configure ValidatorFactory and Validator instances
ValidatorFactory validatorFactory = Validation.buildDefaultValidatorFactory();
Validator validator = validatorFactory.getValidator();
Here, we use Validation.buildDefaultValidatorFactory()
to obtain a properly configured ValidatorFactory, and then acquire the Validator instance from it. This ensures that the validation is set up correctly and ready to be used in the application.
Pitfall 2: Handling Constraint Violation
Another common pitfall is dealing with constraint violations during validation. When validating an object, it's important to handle the constraint violations appropriately, such as logging the errors or taking specific actions based on the validation results.
Solution:
// Handling constraint violations during validation
Set<ConstraintViolation<YourClass>> violations = validator.validate(yourObject);
if (!violations.isEmpty()) {
for (ConstraintViolation<YourClass> violation : violations) {
System.out.println(violation.getPropertyPath() + ": " + violation.getMessage());
}
}
In this solution, we validate the object using the validate
method, and if there are any constraint violations, we iterate through them to handle each violation as required. This ensures that the application responds appropriately to validation errors.
Pitfall 3: Custom Constraint Validation
Developers often need to define custom constraints for specific validation requirements. Implementing custom constraint validators and integrating them with Hibernate Validator can be challenging for those new to the framework.
Solution:
// Example of a custom constraint annotation
@Target({ ElementType.FIELD, ElementType.METHOD, ElementType.PARAMETER })
@Retention(RetentionPolicy.RUNTIME)
@Constraint(validatedBy = CustomValidator.class)
public @interface CustomConstraint {
String message() default "Custom constraint validation failed";
Class<?>[] groups() default {};
Class<? extends Payload>[] payload() default {};
}
// Example of a custom constraint validator
public class CustomValidator implements ConstraintValidator<CustomConstraint, String> {
@Override
public boolean isValid(String value, ConstraintValidatorContext context) {
// Custom validation logic
return /* validation result */;
}
}
In this example, we define a custom constraint annotation @CustomConstraint
and its corresponding validator CustomValidator
to encapsulate custom validation logic. Integrating custom constraints with Hibernate Validator allows developers to address specific validation requirements effectively.
Pitfall 4: Resource Management
Improper resource management, such as failing to close ValidatorFactory or Validator instances, can lead to resource leaks and affect the application's performance and stability.
Solution:
// Closing ValidatorFactory and Validator instances
validatorFactory.close();
It's crucial to properly close the ValidatorFactory
when it's no longer needed to release any resources associated with it. Failing to do so can result in resource leaks, which can impact the application's performance over time.
Pitfall 5: Internationalization of Error Messages
Developers often overlook the internationalization of error messages, which is crucial for building applications that support multiple locales and languages. Hard-coding error messages in a single language can lead to a poor user experience for non-English speakers.
Solution:
// Internationalizing error messages using ResourceBundle
Validator validator = Validation.byDefaultProvider()
.configure()
.messageInterpolator(new ResourceBundleMessageInterpolator(new PlatformResourceBundleLocator("ValidationMessages")))
.buildValidatorFactory()
.getValidator();
By using ResourceBundleMessageInterpolator
and providing the appropriate validation message bundles for different languages, developers can ensure that error messages are displayed in the user's preferred language, enhancing the application's usability.
My Closing Thoughts on the Matter
In conclusion, while Hibernate Validator offers powerful validation capabilities for Java SE applications, developers often face common pitfalls when working with it. By understanding and addressing these pitfalls, such as incorrect configuration, handling constraint violations, defining custom constraints, managing resources, and internationalizing error messages, developers can leverage Hibernate Validator effectively to build robust and reliable applications.
By following the solutions provided in this article, developers can overcome these pitfalls and harness the full potential of Hibernate Validator to ensure comprehensive input validation in their Java SE applications.
With its robust features and the ability to address the common pitfalls discussed, Hibernate Validator remains an essential tool for Java developers striving to achieve comprehensive and reliable input validation in their applications.
For further reading, the official Hibernate Validator documentation provides in-depth information on various aspects of Hibernate Validator and its usage.
Remember, understanding and addressing these common pitfalls ensures that Hibernate Validator is utilized effectively to build Java applications with robust input validation, thereby enhancing the overall quality and reliability of the software.
Checkout our other articles