Master REST: Solving the Puzzle of Concurrent Updates
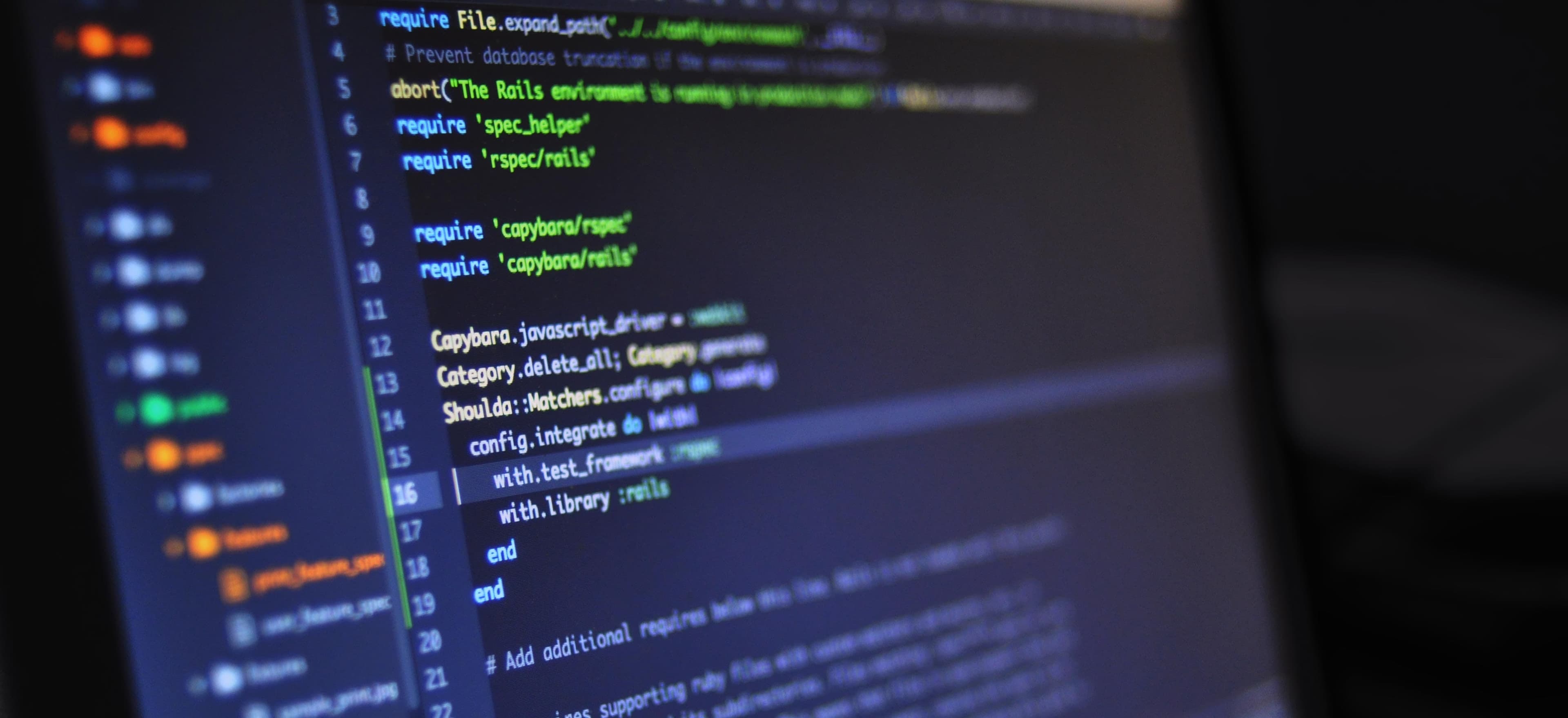
- Published on
Master REST: Solving the Puzzle of Concurrent Updates
In the world of web development, REST (Representational State Transfer) has become the go-to architectural style for designing networked applications. One of the key features of REST is its stateless nature, which allows for better scalability and reliability. However, this statelessness also presents a challenge when dealing with concurrent updates to a resource. In this article, we will explore the problem of concurrent updates in RESTful services and discuss strategies to solve it.
The Challenge of Concurrent Updates
Consider a scenario where multiple clients are trying to update the same resource simultaneously. Without proper handling, this could lead to inconsistencies in the resource state. For example, if two clients fetch the same resource, update it, and then attempt to save their changes, there is a risk of one update overwriting the other, leading to data loss or corruption.
ETags to the Rescue
ETags provide a solution to the problem of concurrent updates in RESTful services. An ETag is a unique identifier assigned to a particular state of a resource. When a client retrieves a resource, the server includes the ETag in the response. The client can then use this ETag when making subsequent requests to the server.
Let's look at an example of how ETags can be used to handle concurrent updates. In this example, we have a simple RESTful API for managing a collection of books. Each book resource has an id
, title
, and author
. When a client retrieves a book resource, the server includes the ETag in the response header.
@GetMapping("/books/{id}")
public ResponseEntity<Book> getBook(@PathVariable Long id) {
Book book = bookService.getBookById(id);
String eTag = calculateETag(book);
return ResponseEntity.ok()
.eTag(eTag)
.body(book);
}
In the above code snippet, the getBook
endpoint retrieves a book by its ID and includes the calculated ETag in the response. The calculateETag
method computes the ETag based on the book's attributes, ensuring that it reflects the current state of the resource.
Conditional Requests with ETags
Now, let's see how the client can use the ETag to make conditional requests. When the client wants to update the book, it includes the If-Match header with the ETag value in the request. This tells the server to perform the update only if the ETag on the server matches the one provided by the client.
@PutMapping("/books/{id}")
public ResponseEntity<?> updateBook(@PathVariable Long id, @RequestBody Book updatedBook,
@RequestHeader("If-Match") String eTag) {
Book existingBook = bookService.getBookById(id);
String currentETag = calculateETag(existingBook);
if (!eTag.equals(currentETag)) {
return ResponseEntity.status(HttpStatus.PRECONDITION_FAILED).build();
}
// Update the book
bookService.updateBook(id, updatedBook);
return ResponseEntity.noContent().build();
}
In the updateBook
endpoint, the server compares the ETag sent by the client with the current ETag of the book. If the ETags match, the update is performed; otherwise, a 412 Precondition Failed
response is returned, indicating that the resource has changed since the client last retrieved it.
Benefits of Using ETags
Using ETags for handling concurrent updates offers several benefits. First, it allows the server to detect concurrent modifications and prevent lost updates. Second, it reduces unnecessary data transfers, as the server can respond with a 304 Not Modified
status when the resource has not changed since the client last retrieved it. This helps improve performance and bandwidth utilization.
A Final Look
In this article, we have explored the problem of concurrent updates in RESTful services and learned how ETags can be used to address this challenge. By leveraging ETags and conditional requests, developers can ensure data consistency and mitigate the risks associated with concurrent updates. When building RESTful APIs, incorporating ETags into the design can lead to more robust and reliable systems.
Mastering the effective use of ETags is essential for any developer working with RESTful services. By understanding and implementing ETags, you can ensure that your applications handle concurrent updates gracefully, providing a seamless and reliable user experience.
Now that you have a deeper understanding of ETags and their role in handling concurrent updates, it's time to incorporate this knowledge into your RESTful API designs and enhance the robustness of your applications.
Incorporating best practices in RESTful API development, such as utilizing ETags for handling concurrent updates, can elevate the performance and reliability of your applications, ultimately leading to a better user experience.
So, go ahead, master the art of using ETags, and conquer the challenge of concurrent updates in your RESTful services. Your users will thank you for it.
Learn more about RESTful API best practices.
Remember, mastering REST is a journey, and continual learning and improvement are key to becoming a proficient RESTful API developer. Keep exploring, keep building, and keep mastering REST!
Happy coding!
Checkout our other articles