Unblock Your App: Mastering callSerially and EDT
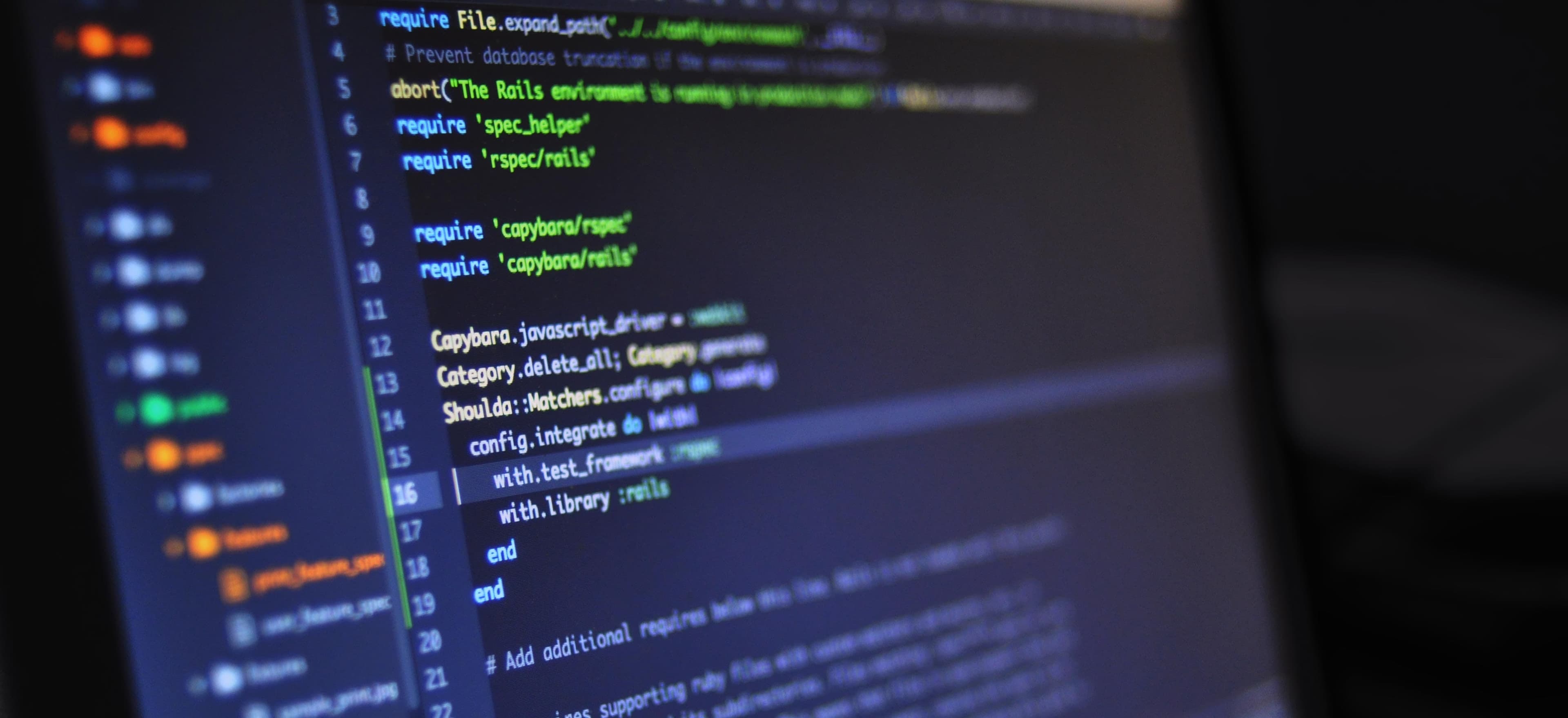
- Published on
Mastering callSerially and EDT in Java
In Java, working with user interfaces often involves dealing with events and ensuring that they are handled properly to maintain a responsive and smooth user experience. One of the key aspects of managing events in Java is understanding the Event Dispatch Thread (EDT) and utilizing the callSerially
method to ensure proper sequencing of tasks. In this post, we will dive into the EDT, explore the callSerially
method, and understand how mastering these concepts can unblock your Java application development.
Understanding the Event Dispatch Thread (EDT)
In Java, the Event Dispatch Thread (EDT) is a crucial aspect of the Swing framework, responsible for handling user interface events. It ensures that all user interface updates and event handling occur in a single, dedicated thread to prevent potential race conditions and maintain the responsiveness of the application.
Why is the EDT important?
The EDT is important because it ensures that all user interface updates and event handling occur in a single thread, avoiding synchronization issues and providing a consistent user experience. In addition, by keeping the UI updates and event handling in a dedicated thread, the application remains responsive, preventing the UI from freezing or becoming unresponsive during intensive tasks.
Example of EDT usage
SwingUtilities.invokeLater(() -> {
// UI update code goes here
});
In the above example, SwingUtilities.invokeLater
is used to schedule the specified code to be executed on the Event Dispatch Thread. This ensures that any UI updates are performed in the context of the EDT, maintaining the integrity of the user interface.
Utilizing callSerially for Sequential Execution
The callSerially
method is a powerful tool for ensuring that tasks are executed sequentially on the EDT. This is particularly useful when dealing with asynchronous tasks and updates to the user interface that must occur in a specific order.
Why use callSerially?
Using callSerially
is crucial for maintaining the order of tasks that need to be executed on the EDT. When multiple tasks need to be performed in sequence, callSerially
ensures that they are executed one after the other, preventing potential race conditions and maintaining the consistency of the user interface.
Example of callSerially usage
someComponent.callSerially(() -> {
// Task 1
});
someComponent.callSerially(() -> {
// Task 2
});
In the above example, callSerially
is used to schedule two tasks to be executed sequentially on the EDT. This guarantees that Task 2 will only be executed after Task 1 has completed, ensuring the desired order of execution.
Unblocking Your Application with callSerially and EDT
Mastering the use of callSerially
and understanding the EDT can significantly unblock your Java application development. By leveraging these concepts effectively, you can ensure that your user interface remains responsive, tasks are executed in the correct order, and potential synchronization issues are mitigated.
Avoiding UI freezing
By utilizing callSerially
for sequential execution of tasks on the EDT, you can prevent the UI from freezing or becoming unresponsive during intensive operations. This is crucial for providing a smooth and responsive user experience, especially in applications with complex user interfaces.
Maintaining task order
When dealing with tasks that must be executed in a specific order, such as updating UI components based on asynchronous data retrieval, callSerially
ensures that the tasks are executed sequentially as intended. This helps in maintaining the consistency and integrity of the user interface.
Preventing synchronization issues
Using callSerially
helps in preventing potential synchronization issues that can arise when multiple tasks attempt to update the user interface concurrently. By enforcing sequential execution on the EDT, you can avoid race conditions and ensure the stability of your application.
My Closing Thoughts on the Matter
In Java application development, mastering the concepts of callSerially and understanding the Event Dispatch Thread (EDT) are essential for maintaining a responsive and consistent user interface. By leveraging callSerially
for sequential task execution and ensuring that UI updates occur on the EDT, you can unblock your application and provide a seamless user experience. Understanding these concepts and applying them effectively can elevate the quality of your Java applications, making them more robust and user-friendly.
Now that you have a solid understanding of callSerially and EDT, it's time to put this knowledge into practice and elevate the performance and responsiveness of your Java applications.
Remember, mastering these concepts can make a substantial difference in the overall experience of your Java applications. Cheers to unblocking your app with callSerially and EDT!