Streamline Your Workflow: Mastering Continuous Integration
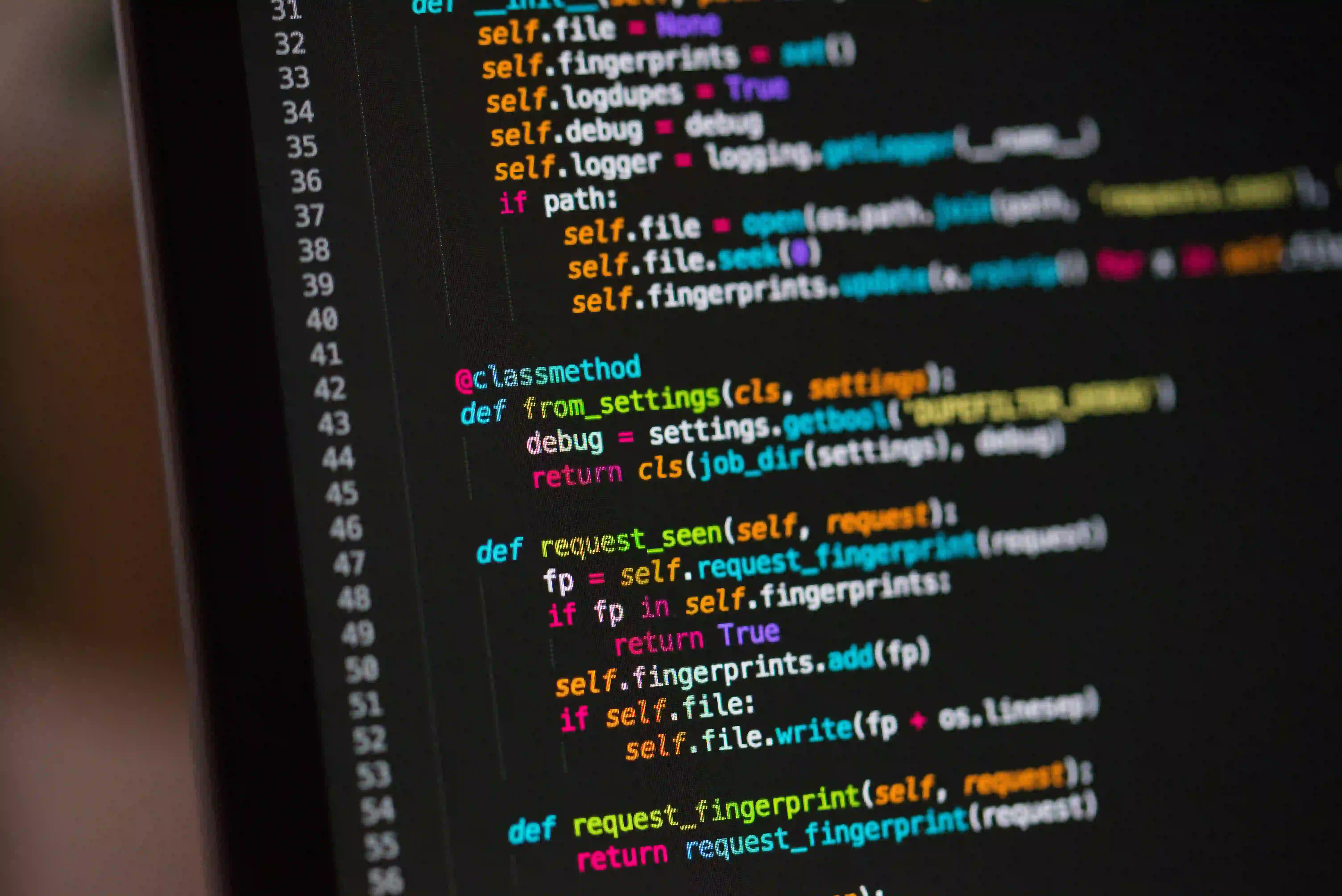
Streamline Your Workflow: Mastering Continuous Integration with Java
In the fast-paced world of software development, maintaining efficiency, code quality, and seamless collaboration are paramount. This is where Continuous Integration (CI) comes into play, a practice that has drastically transformed how developers approach coding, testing, and deployment. For Java developers, mastering CI not only elevates the workflow but also paves the way for robust, error-free applications. This comprehensive guide takes you through the essentials of Continuous Integration for Java, offering practical insights, code examples, and best practices to streamline your development process.
Understanding Continuous Integration
Continuous Integration is a development practice where developers frequently integrate their code changes into a shared repository, preferably multiple times a day. Each integration is automatically verified by building the project and running automated tests, ensuring that the new changes do not break or disrupt the application. This practice highlights errors quickly, improves software quality, and accelerates the delivery process.
Why Continuous Integration Matters?
- Rapid Feedback Loop: Identifies integration and regression errors quickly.
- Increased Transparency: Offers visibility into the project’s health to all team members.
- Enhanced Collaboration: Encourages team members to integrate work frequently.
Setting Up Continuous Integration for Java
Implementing CI in your Java project is a game-changer. Let’s walk through how to set up a basic CI pipeline using Jenkins, one of the most popular open-source automation servers.
Step 1: Install Jenkins
Begin by installing Jenkins on your machine. You can download it from the official Jenkins website. Ensure you meet the necessary system requirements. For detailed installation instructions, refer to their documentation.
Step 2: Create a New Jenkins Project
After installation and opening Jenkins in your browser:
- Click on “New Item”.
- Enter a name for your project.
- Select “Freestyle project”.
Step 3: Configure Source Code Management
Under the project configuration:
- Select “Git” in Source Code Management.
- Enter your repository URL.
- Add credentials if your repository is private.
Step 4: Add Build Triggers
Configure Jenkins to poll SCM (Source Code Management) to check for changes:
- Check “Poll SCM”.
- Schedule the poll using cron syntax, e.g.,
H/5 * * * *
to poll every five minutes.
Step 5: Configure Build Steps
Add the following build step to compile your Java project:
- Click “Add build step”.
- Choose “Invoke top-level Maven targets”.
- In “Goals”, type
clean install
.
Step 6: Test Reporting with JUnit
For Java projects, it's crucial to generate test reports. Jenkins can archive JUnit test reports and provide detailed test results:
- Click “Add post-build action”.
- Select “Publish JUnit test result report”.
- Specify the path to your test reports, typically
**/target/surefire-reports/*.xml
.
Step 7: Monitor and Iterate
Once the setup is complete, commit a change to your repository. Jenkins will automatically trigger a build based on the poll schedule. Monitor the build results, adjust configurations as necessary, and continue improving your code based on feedback.
Example: Automating Java Builds with Maven
Maven is a powerful tool for managing and automating Java projects. Integrating Maven with Jenkins streamlines the build process. Below is an example pom.xml
file snippet that illustrates how to configure Maven to compile and package a simple Java application:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>java-ci-example</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<!-- Add your project dependencies here -->
</dependencies>
<build>
<plugins>
<!-- Maven Compiler Plugin -->
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
<!-- Maven Surefire Plugin for running tests -->
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.2</version>
</plugin>
</plugins>
</build>
</project>
Why This Matters: The maven-compiler-plugin
ensures your Java code compiles correctly using Java 1.8, while the maven-surefire-plugin
is essential for executing unit tests and generating reports. This setup automates the build and test process, reducing manual intervention and the risk of human error.
Best Practices for Continuous Integration in Java
- Commit Regularly: Frequent commits reduce integration issues and ensure the CI pipeline remains efficient.
- Write Meaningful Tests: Quality over quantity. Ensure your tests cover critical functionalities and potential failure points.
- Keep the Build Fast: Optimize build times to ensure quick feedback. Slow builds hinder productivity.
- Maintain a Clean Environment: Use containers or virtual machines to create consistent, isolated build environments.
- Monitor and Optimize: Regularly review your CI process. Identify bottlenecks and continuously refine your workflow.
The Bottom Line
Continuous Integration is not merely a technical process; it's a philosophy that promotes better coding practices, teamwork, and software quality. For Java developers, embracing CI means embarking on a journey towards more efficient, reliable, and collaborative development. By following the guidelines, setting up a CI pipeline, and maneuvering through the intricacies of Maven integration in Jenkins, you're well on your way to mastering Continuous Integration, transforming your workflow into a streamlined, error-resilient process. Start now, and elevate your Java projects to new heights.
Incorporating Continuous Integration into your development lifecycle is a significant step towards modernizing your approach to software construction and delivery. As technology evolves, so do the tools and practices. Keeping up with these changes ensures your projects remain competitive, robust, and above all, successful in meeting the demands of users and businesses alike.