Simplifying If-Else Chaos in Lambda Functions
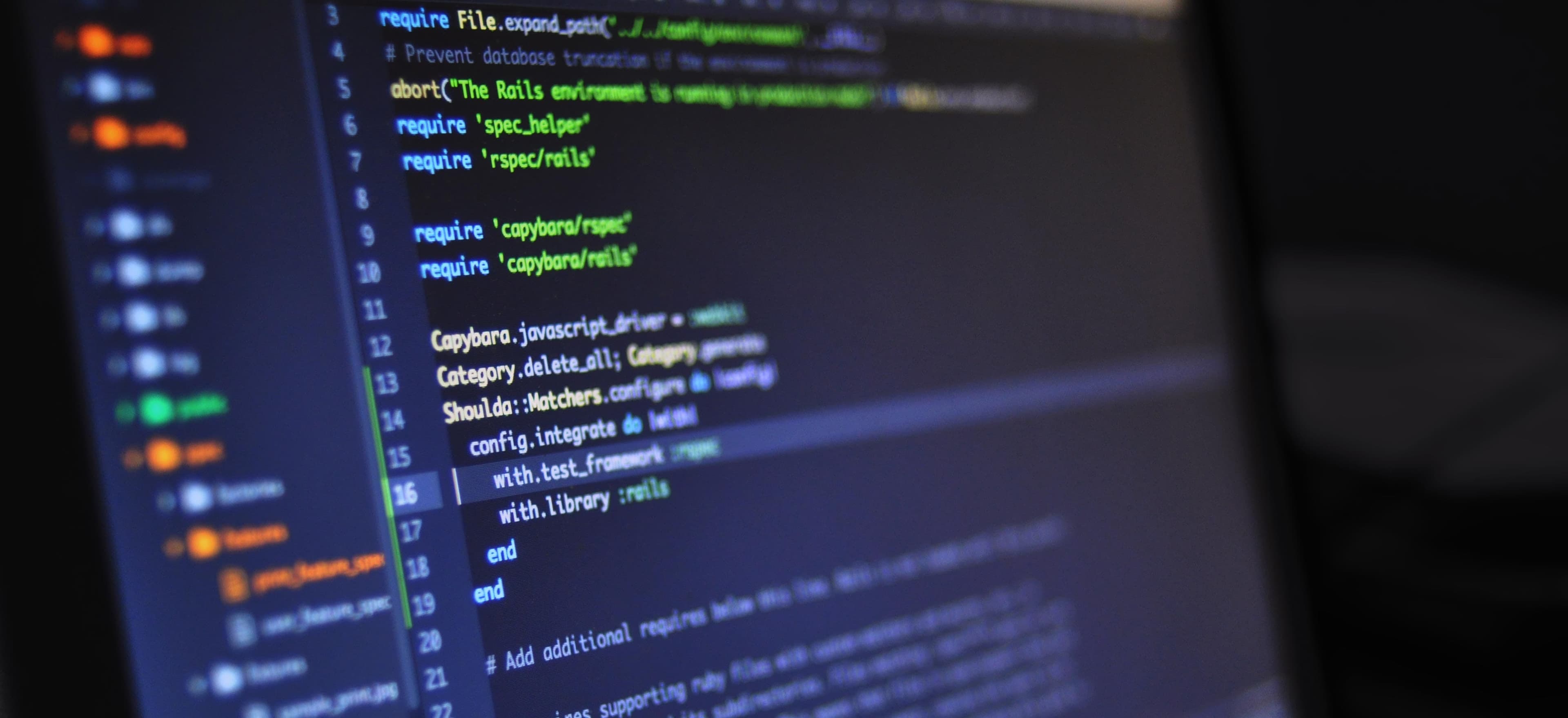
- Published on
Simplifying If-Else Chaos in Lambda Functions
When working with lambda functions in Java, it's common to come across complex if-else conditions that can make the code hard to read and maintain. In this blog post, we'll explore some strategies for simplifying if-else chaos in lambda functions, making your code more elegant and easier to work with.
The Problem
Consider a simple example where we have a lambda function that takes an integer input and performs different operations based on the input value:
Function<Integer, String> processNumber = (input) -> {
if (input == 0) {
return "Zero";
} else if (input == 1) {
return "One";
} else if (input == 2) {
return "Two";
} else {
return "Other";
}
};
While this code works, it can become cumbersome to manage as the number of conditions grows. It's not hard to imagine a scenario where the if-else ladder becomes long and unwieldy.
Strategy 1: Switch-Case Statements
One way to simplify multiple if-else conditions is to use switch-case statements. We can refactor the previous example using switch-case like this:
Function<Integer, String> processNumber = (input) -> {
switch (input) {
case 0:
return "Zero";
case 1:
return "One";
case 2:
return "Two";
default:
return "Other";
}
};
Using a switch-case statement can make the code more readable, especially when dealing with a larger number of conditions. It also allows for easier handling of duplicate cases.
Strategy 2: Function Mapping
Another approach to handle complex if-else conditions is by using a map to store the mappings between input values and the corresponding operations. We can then use this map to look up the operation for a given input. This approach can be particularly useful when the operations are not simple one-liners.
Let's see how we can refactor the previous example using function mapping:
Function<Integer, String> processNumber = (input) -> {
Map<Integer, String> numberMap = new HashMap<>();
numberMap.put(0, "Zero");
numberMap.put(1, "One");
numberMap.put(2, "Two");
return numberMap.getOrDefault(input, "Other");
};
By using a map, we eliminate the need for multiple if-else or switch-case blocks, leading to more concise and maintainable code. Additionally, the map can be dynamically populated, allowing for greater flexibility.
Strategy 3: Enum-Based Approach
If the conditions are more complex and involve multiple related operations, using an enum-based approach can be beneficial. We can define an enum to represent the input values and their corresponding operations, which helps in organizing and encapsulating related behavior.
Here's how we can refactor the example using an enum-based approach:
enum NumberType {
ZERO(0, "Zero"),
ONE(1, "One"),
TWO(2, "Two"),
OTHER(-1, "Other");
private final int value;
private final String label;
NumberType(int value, String label) {
this.value = value;
this.label = label;
}
public static NumberType fromValue(int value) {
for (NumberType type : values()) {
if (type.value == value) {
return type;
}
}
return OTHER;
}
public String getLabel() {
return label;
}
}
Function<Integer, String> processNumber = (input) -> {
NumberType type = NumberType.fromValue(input);
return type.getLabel();
};
By using an enum for the conditions, we can encapsulate related logic within the enum itself, making the code more organized and easier to manage. It also allows for additional methods and properties to be associated with each enum value, offering more flexibility.
In Conclusion, Here is What Matters
When dealing with complex if-else conditions in lambda functions, it's essential to seek strategies that make the code more readable, maintainable, and concise. Switching to switch-case statements, utilizing function mapping, and employing an enum-based approach are effective ways to simplify if-else chaos in lambda functions.
By choosing the right strategy based on the complexity and nature of the conditions, you can enhance the clarity and elegance of your code. Remember, the goal is not just to make the code work, but to make it work in the most efficient and maintainable manner possible.
So, the next time you find yourself drowning in if-else chaos within lambda functions, consider these strategies to bring order and simplicity to your code.
Start simplifying your lambda functions today!