Master JSON in Android: A Guide to Creating & Parsing
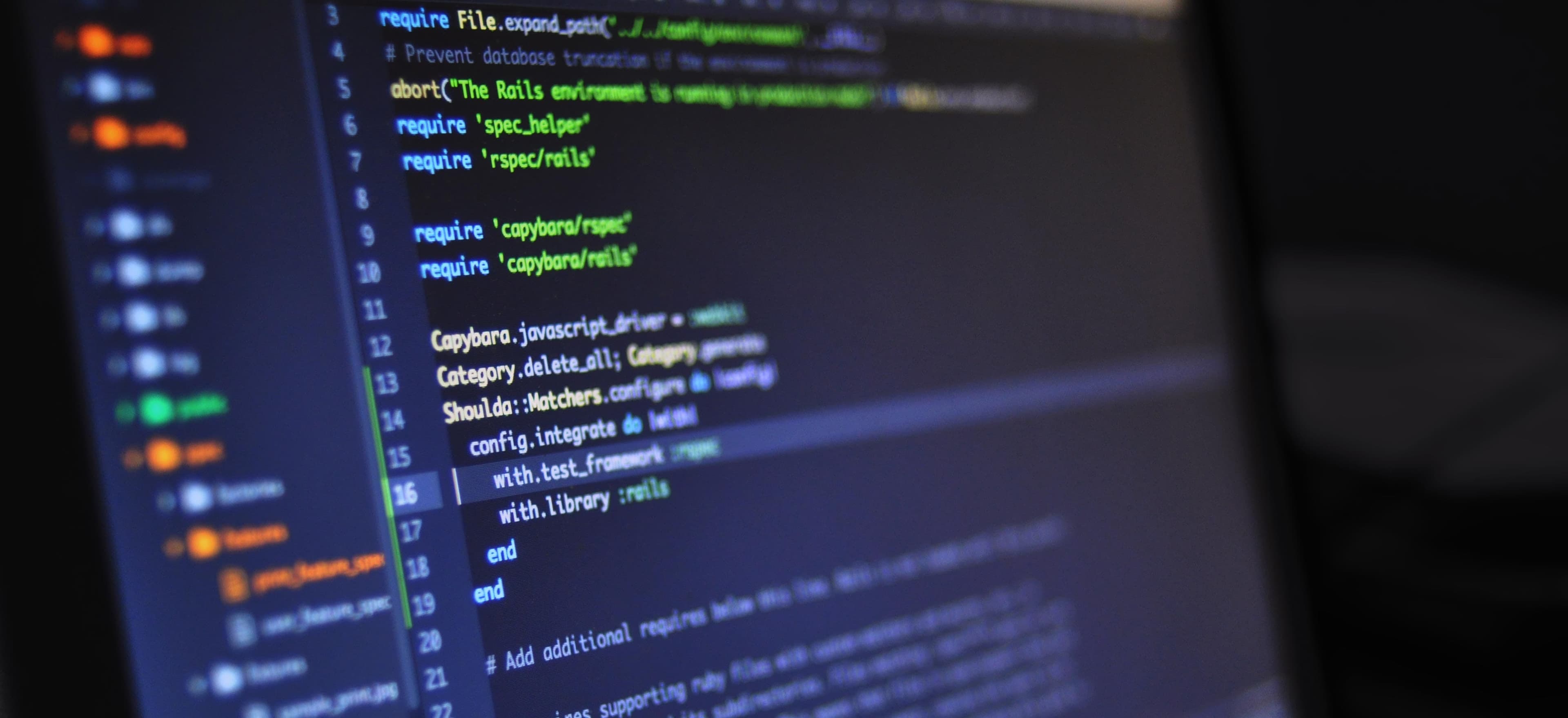
- Published on
Master JSON in Android: A Guide to Creating & Parsing
JSON (JavaScript Object Notation) has become a popular data interchange format in the programming world due to its lightweight and human-readable nature. In the Android environment, mastering JSON is essential for creating and parsing data from web services, APIs, and local storage. In this guide, we will explore how to work with JSON in Android, covering the creation of JSON data and the parsing of JSON responses.
What is JSON?
JSON is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. It is based on a subset of the JavaScript Programming Language, Standard ECMA-262 3rd Edition - December 1999. JSON is often used to transmit data between a server and a web application, serving as an alternative to XML.
Creating JSON Data in Android
In Android, creating JSON data is often necessary when preparing data to be sent to a server or when storing complex data structures locally. The JSONObject
class and the related classes in the org.json
package provide the necessary tools for creating JSON objects and arrays.
import org.json.JSONException;
import org.json.JSONObject;
// Create a JSON object
JSONObject personObject = new JSONObject();
try {
// Add key-value pairs to the JSON object
personObject.put("name", "John Doe");
personObject.put("age", 30);
personObject.put("isStudent", false);
} catch (JSONException e) {
e.printStackTrace();
}
// Convert the JSON object to a string
String jsonString = personObject.toString();
In the above code snippet, we create a JSON object representing a person with name, age, and student status. We then convert the JSON object to a string using the toString()
method.
Why Use JSON for Data Transmission?
JSON is widely used for data transmission due to its lightweight and easy-to-parse nature. Unlike XML, JSON has a more straightforward and less verbose syntax, making it efficient for transmitting data over the network. Additionally, JSON's compatibility with JavaScript makes it an excellent choice for web-based applications and APIs.
Parsing JSON Responses in Android
When working with web services and APIs in Android, parsing JSON responses is a common task. The org.json
package provides classes such as JSONObject
and JSONArray
to parse JSON data received from a server.
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
// Example JSON response from a server
String jsonResponse = "{ \"name\": \"John Doe\", \"age\": 30, \"isStudent\": false }";
try {
// Parse the JSON response into a JSON object
JSONObject personObject = new JSONObject(jsonResponse);
// Retrieve values from the JSON object
String name = personObject.getString("name");
int age = personObject.getInt("age");
boolean isStudent = personObject.getBoolean("isStudent");
// Log the parsed values
Log.d("JSON Parsing", "Name: " + name);
Log.d("JSON Parsing", "Age: " + age);
Log.d("JSON Parsing", "Is Student: " + isStudent);
} catch (JSONException e) {
e.printStackTrace();
}
In the above code snippet, we parse a JSON response representing a person and extract the name, age, and student status from the JSON object. We then log the parsed values for verification.
Why Parse JSON in Android?
Parsing JSON responses is crucial in Android development, especially when working with web APIs and services. By parsing JSON data, we can extract meaningful information from the server's response and use it within our Android application. This allows for dynamic and up-to-date content to be displayed to the user.
Best Practices for Working with JSON in Android
-
Use Deserialization Libraries: Consider using third-party libraries such as Gson or Jackson for parsing JSON in Android. These libraries provide convenient methods for serializing and deserializing JSON data into Java objects, reducing the manual parsing effort and error-prone code.
-
Handle JSON Exceptions: When working with JSON in Android, always handle JSON-related exceptions such as
JSONException
to prevent crashes and unexpected behavior in your application. Use try-catch blocks to capture and handle JSON parsing exceptions gracefully. -
Validate JSON Data: Before parsing or using JSON data in Android, it's essential to validate its structure and content to avoid potential runtime errors. Validating JSON data ensures that it meets the expected format and prevents unexpected behavior during parsing.
-
Consider Using Kotlin: If you're starting a new Android project or have the flexibility to introduce Kotlin, consider leveraging Kotlin's concise syntax and built-in support for JSON parsing. Kotlin's data classes and extension functions can simplify JSON handling and parsing.
The Last Word
Mastering JSON in Android is pivotal for data interchange, communication with web services, and effective data management. Understanding how to create and parse JSON data empowers Android developers to interact seamlessly with remote servers and APIs while efficiently handling and processing data within their applications. By adhering to best practices and leveraging the provided tools and libraries, Android developers can streamline their JSON-related tasks and enhance the overall user experience of their applications.
Now that you have a strong foundation in creating and parsing JSON in Android, it's time to explore real-world scenarios and more advanced techniques for handling JSON data within your Android applications.
Happy coding!
For additional reference, you may find the official Android Developer documentation on JSON helpful.