Unlocking Java Objects: A Dive into ObjectStreamClass
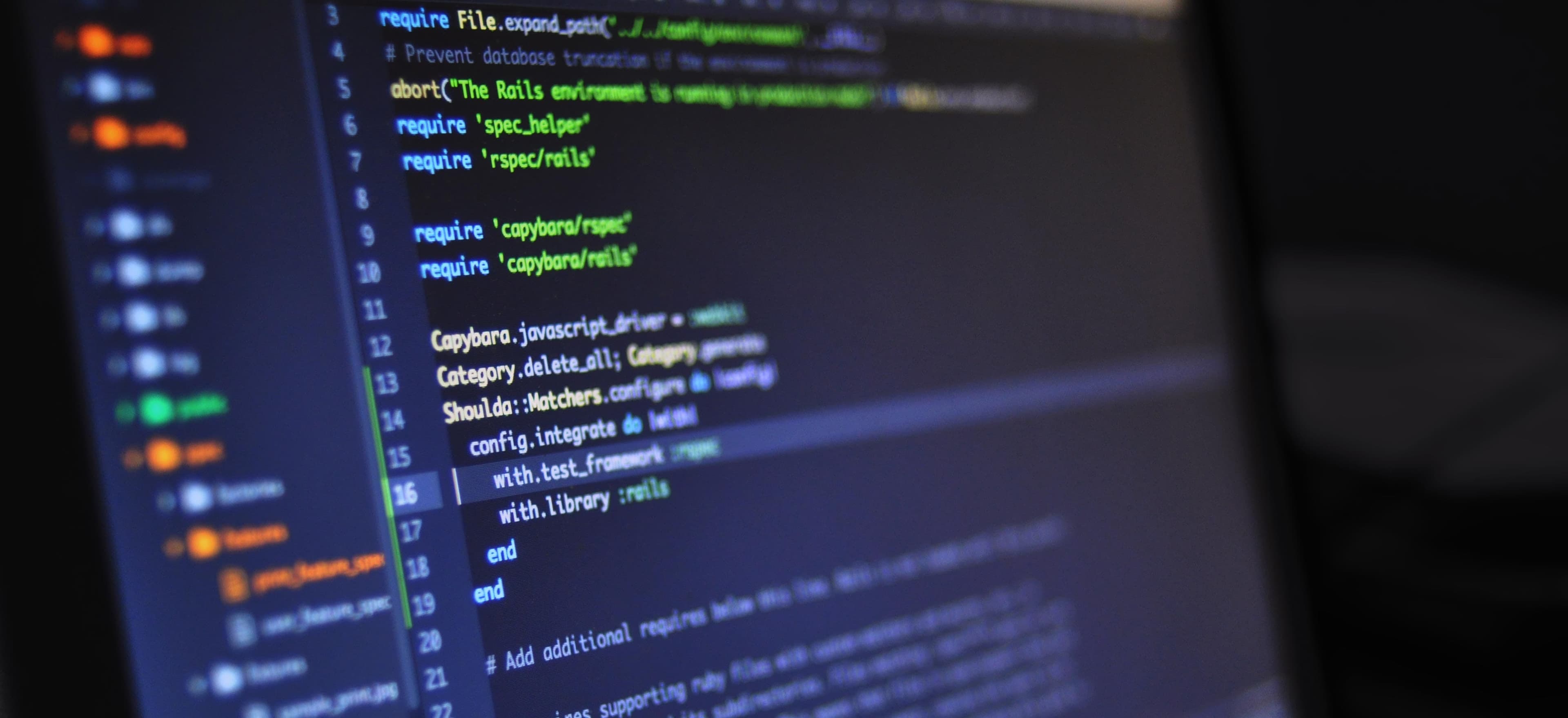
- Published on
Unlocking Java Objects: A Dive into ObjectStreamClass
When working with Java, the concept of object serialization is crucial for storing and transmitting objects. One fundamental aspect of object serialization is the ObjectStreamClass
. This class plays a pivotal role in the serialization and deserialization process in Java. In this blog post, we will explore the ObjectStreamClass
in detail, understand its significance, and learn how to utilize it effectively in Java applications.
What is ObjectStreamClass?
In Java, the ObjectStreamClass
class is responsible for providing important information about the serialization of a class. It encapsulates the various properties of a class, such as the class name, serialVersionUID, field names, and field types. These details are essential for accurately reconstructing objects during deserialization.
Understanding the Significance of ObjectStreamClass
The ObjectStreamClass
class holds significance due to its pivotal role in the serialization and deserialization process. It helps in identifying and verifying the compatibility of a serialized object during deserialization. The serialVersionUID
is particularly crucial, as it enables the JVM to determine if the serialized object's class definition matches the local class definition. This allows for smooth deserialization without encountering compatibility issues.
Exploring ObjectStreamClass in Action
Let's delve into a practical example to grasp the utility of ObjectStreamClass
. Consider a simple Java class Customer
that needs to be serialized and deserialized.
import java.io.*;
public class Customer implements Serializable {
private static final long serialVersionUID = 1L;
private String name;
private int age;
// Getters and setters
}
In the code snippet above, the Customer
class implements the Serializable
interface, and a serialVersionUID
is explicitly defined. Now, let's examine how ObjectStreamClass
can provide insights into the serialization properties of the Customer
class.
public class ObjectStreamClassDemo {
public static void main(String[] args) {
ObjectStreamClass osc = ObjectStreamClass.lookup(Customer.class);
System.out.println("Class name: " + osc.getName());
System.out.println("SerialVersionUID: " + osc.getSerialVersionUID());
ObjectStreamField[] fields = osc.getFields();
for (ObjectStreamField field : fields) {
System.out.println("Field: " + field.getName() + ", Type: " + field.getType());
}
}
}
In the above code, we utilize the lookup
method of ObjectStreamClass
to obtain information about the Customer
class. We then retrieve and display the class name, serialVersionUID
, and the fields present in the class. This demonstrates how ObjectStreamClass
empowers developers by providing critical class serialization details.
Leveraging ObjectStreamClass for Enhanced Serialization
One of the key advantages of ObjectStreamClass
is its ability to facilitate versioning of serialized objects. When a class undergoes changes, ensuring backward and forward compatibility becomes essential. The serialVersionUID
plays a crucial role in this aspect, and ObjectStreamClass
aids in managing versioning effectively.
Let's consider a scenario where the Customer
class undergoes a change by adding a new field email
.
public class Customer implements Serializable {
private static final long serialVersionUID = 1L;
private String name;
private int age;
private String email;
// Getters and setters
}
Now, we can leverage ObjectStreamClass
to handle versioning during deserialization.
public class ObjectStreamClassVersioningDemo {
public static void main(String[] args) {
try {
FileInputStream fileIn = new FileInputStream("customer.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
Customer customer = (Customer) in.readObject();
in.close();
fileIn.close();
System.out.println("Customer name: " + customer.getName());
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
In the above scenario, even after the modification in the Customer
class, ObjectStreamClass
assists in successful deserialization without compromising compatibility.
To Wrap Things Up
In conclusion, ObjectStreamClass
is a crucial aspect of Java object serialization. It provides essential information about the class structure, aiding in seamless serialization and deserialization. Understanding its significance and leveraging it effectively enables developers to manage versioning and compatibility of serialized objects efficiently.
As developers, harnessing the power of ObjectStreamClass
adds a new dimension to handling object serialization in Java, ensuring robust and adaptable systems.
By delving into the intricacies of ObjectStreamClass
, we have unearthed its vital role in maintaining the integrity and compatibility of serialized objects, propelling Java developers towards enhanced serialization practices.
Incorporating ObjectStreamClass
knowledge into your Java arsenal equips you with the prowess to navigate the complexities of object serialization with confidence and proficiency.
So, embrace the power of ObjectStreamClass
and chart a course towards robust, version-compatible serialization in your Java journey.
Are you ready to take your Java serialization prowess to the next level with ObjectStreamClass
?
Remember, the realm of Java serialization holds many nuances, and mastering the intricacies of ObjectStreamClass
is a significant stride towards serialization excellence.
With comprehensive comprehension of ObjectStreamClass
, the realm of Java serialization unlocks its full potential, empowering you to build resilient, version-compatible applications.
Unlock the potential of ObjectStreamClass
and soar to new heights in the art of Java object serialization!
Checkout our other articles