Mastering Apache Camel for Java Newbies: A Quick Guide
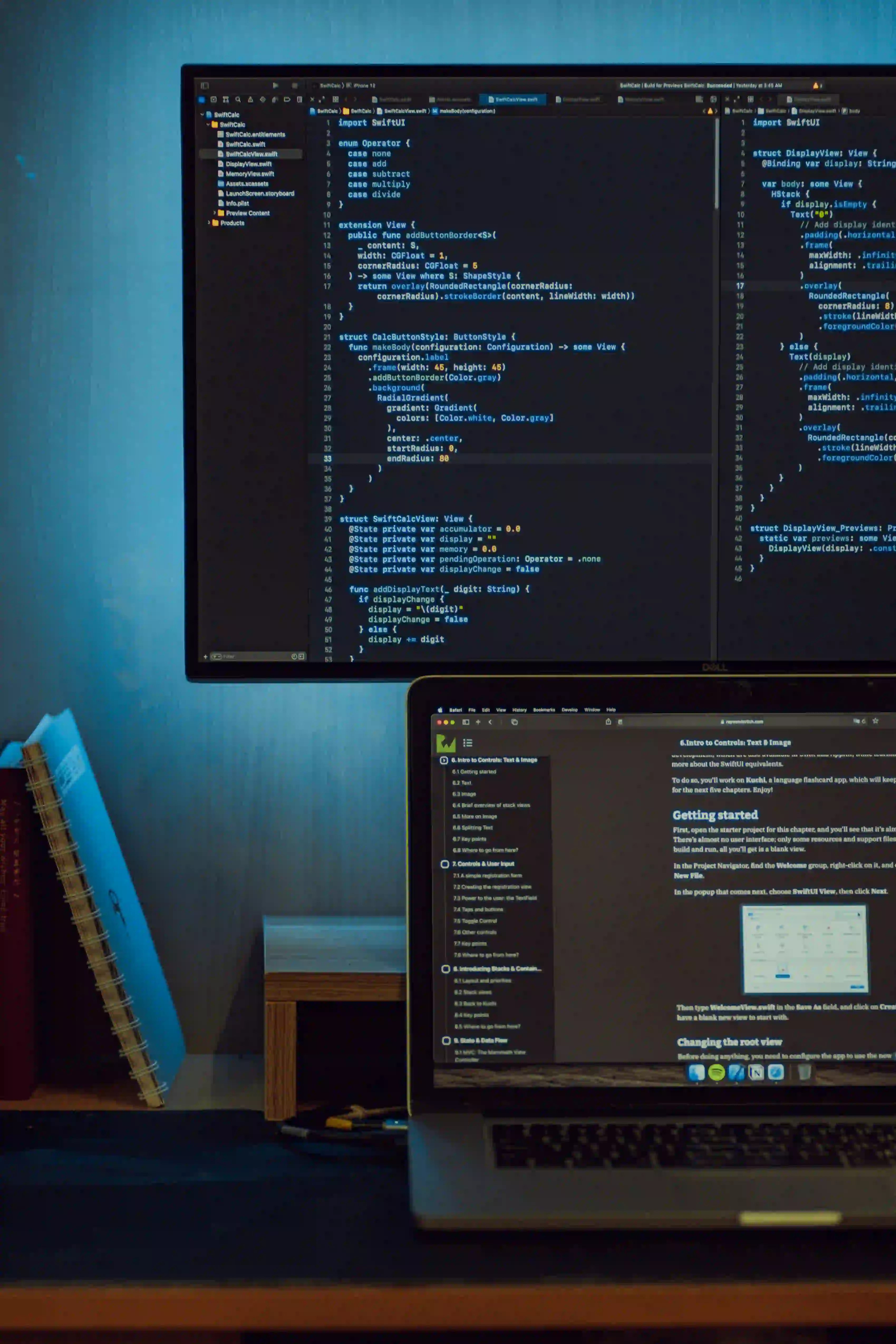
Mastering Apache Camel for Java Newbies: A Quick Guide
If you are a Java developer looking to enhance your skills in building integration systems, Apache Camel is a framework you should definitely consider. In this beginner's guide, we will walk you through the basics of Apache Camel, show you how to get started with it, and demonstrate some fundamental concepts through practical code examples.
What is Apache Camel?
Apache Camel is an open-source Java framework that focuses on making integration of different systems easy. It provides a rule-based routing and mediation engine that allows you to define routing and mediation rules in a variety of domain-specific languages. Camel facilitates the integration of different systems using a wide range of protocols and technologies.
Getting Started with Apache Camel
To get started with Apache Camel, you'll first need to set up your development environment. You can easily include Apache Camel in your Java project using Maven or Gradle by adding the appropriate dependency to your build file.
Here's a basic example of a Maven dependency for Apache Camel:
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-core</artifactId>
<version>x.x.x</version>
</dependency>
Replace x.x.x
with the latest version of Apache Camel.
Creating a Simple Camel Route
Once you have Apache Camel included in your project, you can start creating your first Camel route. A Camel route consists of components, endpoints, and processors.
Here's an example of a simple Camel route that routes a message from one endpoint to another:
import org.apache.camel.builder.RouteBuilder;
public class MyCamelRoute extends RouteBuilder {
@Override
public void configure() throws Exception {
from("direct:start")
.to("log:output");
}
}
In this example, we create a route that consumes messages from the direct:start
endpoint and logs the messages to the console using the log:output
endpoint. The from
method defines the input endpoint, and the to
method defines the output endpoint.
Why Use Apache Camel?
Apache Camel simplifies the integration of different systems by providing a wide range of components and a simple yet powerful DSL for defining routing and mediation rules. It allows you to focus on the business logic of your integration system rather than the low-level details of the integration process.
With Camel, you can easily connect to various systems such as databases, message brokers, RESTful services, and more, without having to write complex integration code from scratch. This enables you to build robust and maintainable integration solutions in a fraction of the time it would take using traditional integration approaches.
Handling Message Transformation
One of the key features of Apache Camel is its support for message transformation. Camel provides a variety of components and tools for transforming messages as they pass through the integration route.
Here's an example of how you can use Camel to transform a message from one format to another:
from("direct:input")
.setBody(simple("Hello, ${header.name}"))
.to("mock:output");
In this example, the setBody
method is used to transform the message by setting its body to a new value. You can also use processors and transformers to apply more complex transformations to the message payload.
Error Handling in Apache Camel
Error handling is an important aspect of any integration system. Apache Camel provides robust support for error handling and fault tolerance.
Here's an example of how you can define error handling in a Camel route:
onException(Exception.class)
.handled(true)
.to("log:error");
In this example, the onException
method is used to define a handler for a specific type of exception. The handled
method is used to indicate whether the exception should be marked as handled, and the to
method is used to define the endpoint where the error should be routed.
Using Enterprise Integration Patterns (EIP)
Apache Camel is built on top of the Enterprise Integration Patterns (EIP) book by Gregor Hohpe and Bobby Woolf. EIP provides a set of patterns to solve recurring problems in the integration of systems. Apache Camel implements these patterns, making it easy to apply them in your integration solutions.
Here's an example of how you can use the Content-Based Router pattern in Apache Camel:
from("direct:input")
.choice()
.when(header("type").isEqualTo("A"))
.to("activemq:A")
.when(header("type").isEqualTo("B"))
.to("activemq:B")
.otherwise()
.to("activemq:other");
In this example, the choice
method is used to apply the Content-Based Router pattern, which routes messages to different endpoints based on the value of a header.
Wrapping Up
Apache Camel is a powerful integration framework that simplifies the process of integrating different systems using a variety of protocols and technologies. By providing a rich set of components, a versatile DSL, and seamless integration with Enterprise Integration Patterns, Apache Camel enables Java developers to build robust and maintainable integration solutions with ease.
In this quick guide, we've covered the basics of Apache Camel and demonstrated how to create a simple Camel route, handle message transformation, manage error handling, and apply Enterprise Integration Patterns. We hope this has piqued your interest in exploring Apache Camel further and leveraging its capabilities to streamline your integration projects.
To dive deeper into the world of Apache Camel, you can explore the official Apache Camel documentation and the book Camel in Action. Happy coding with Camel!