Fixing SLF4J Logging Issues in Your Eclipse Plugins
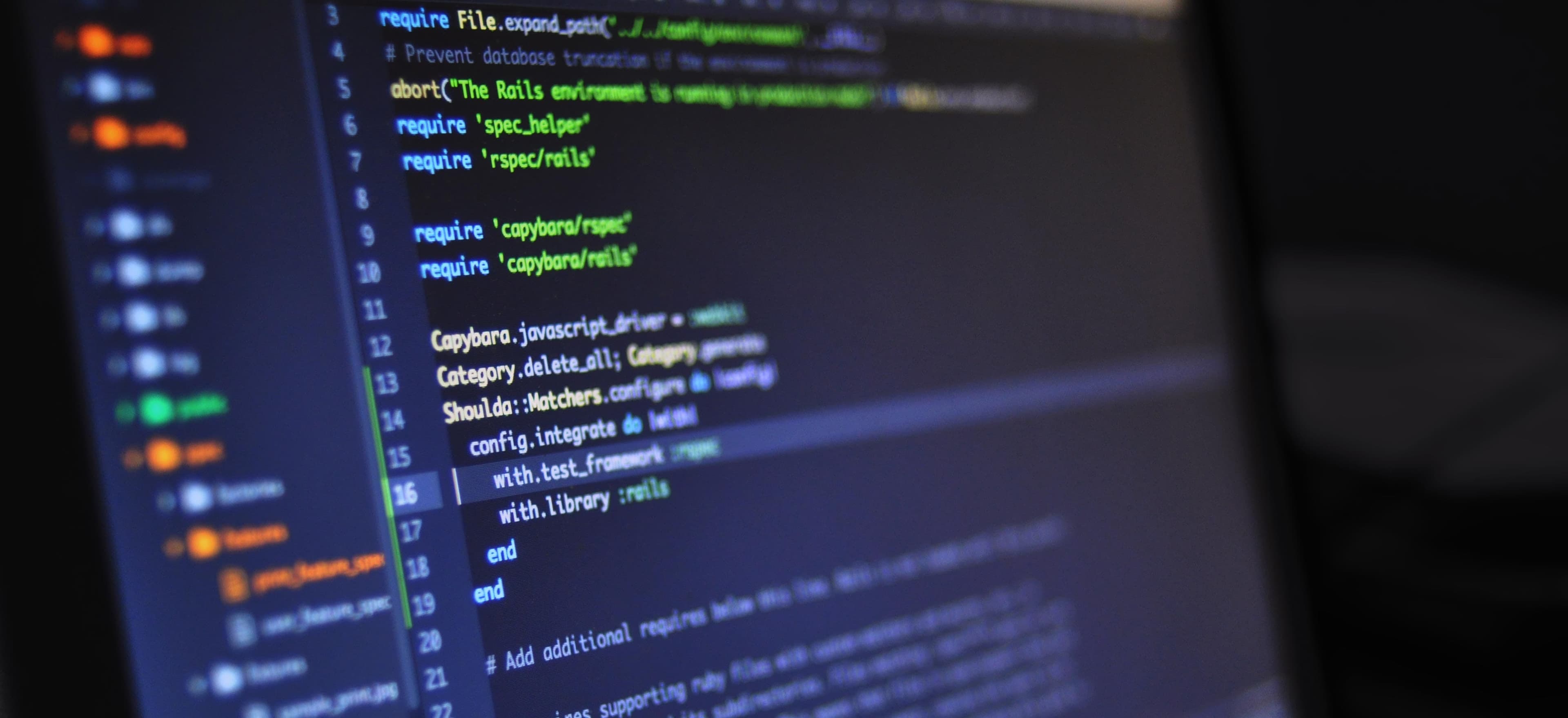
- Published on
Fixing SLF4J Logging Issues in Your Eclipse Plugins
When developing Eclipse plugins with Java, it's essential to implement proper logging to facilitate debugging and monitoring of your code. The Simple Logging Facade for Java (SLF4J) serves as a robust logging API, providing a simple facade for various logging frameworks.
In this guide, we'll explore how to address common SLF4J logging issues that developers encounter when working with Eclipse plugins and Java.
Understanding SLF4J
SLF4J is designed as a logging facade, providing a simple API that allows developers to plug in their desired logging framework at deployment time. By using SLF4J, developers can write log statements using the SLF4J API, and choose their logging implementation later.
Common SLF4J Issues in Eclipse Plugins
1. Missing SLF4J Binding
When working with Eclipse plugins and SLF4J, it's not uncommon to encounter a "Missing slf4j binding" error. This issue typically arises when the SLF4J API is present, but no binding is found at runtime.
2. Conflicting Logging Implementations
Eclipse plugins often rely on various libraries, each potentially using its own logging implementation. This can lead to conflicts and unexpected behavior when working with SLF4J in your Eclipse plugin.
Now, let's delve into how to address these issues effectively.
Fixing SLF4J Logging Issues
1. Resolving Missing SLF4J Binding
To address the "Missing slf4j binding" issue, you need to ensure that a compatible SLF4J binding is included in your project's dependencies. For example, you can include the slf4j-simple
binding by adding the following dependency to your Maven pom.xml
:
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-simple</artifactId>
<version>1.7.30</version>
</dependency>
In the above snippet, we're including the slf4j-simple
binding, which provides a simple implementation for SLF4J, making it useful for development and testing purposes.
2. Resolving Conflicting Logging Implementations
To address conflicting logging implementations in your Eclipse plugin, you need to ensure that only one logging implementation is used throughout your project. You can achieve this by excluding other logging dependencies and specifying the SLF4J binding you intend to use.
For instance, if you're using Maven, you can exclude other logging implementations and specify slf4j-simple
as the sole logging implementation:
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.30</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-simple</artifactId>
<version>1.7.30</version>
<exclusions>
<exclusion>
<groupId>org.slf4j</groupId>
<artifactId>*</artifactId>
</exclusion>
</exclusions>
</dependency>
By excluding other logging implementations, you ensure that only the specified SLF4J binding is used, mitigating potential conflicts.
My Closing Thoughts on the Matter
By following these steps, you can effectively address common SLF4J logging issues encountered when developing Eclipse plugins with Java. It's crucial to ensure that the appropriate SLF4J binding is included, and to resolve any conflicting logging implementations to streamline your logging framework within your Eclipse plugin development.
Remember, adopting best practices for logging not only aids in debugging and monitoring your Eclipse plugins but also contributes to maintaining a robust and efficient codebase.
For further reading and detailed documentation, you can refer to the official SLF4J documentation and the SLF4J user manual.
Happy coding!
Checkout our other articles