Mastering Apache Thrift: Avoid Beginner Pitfalls!
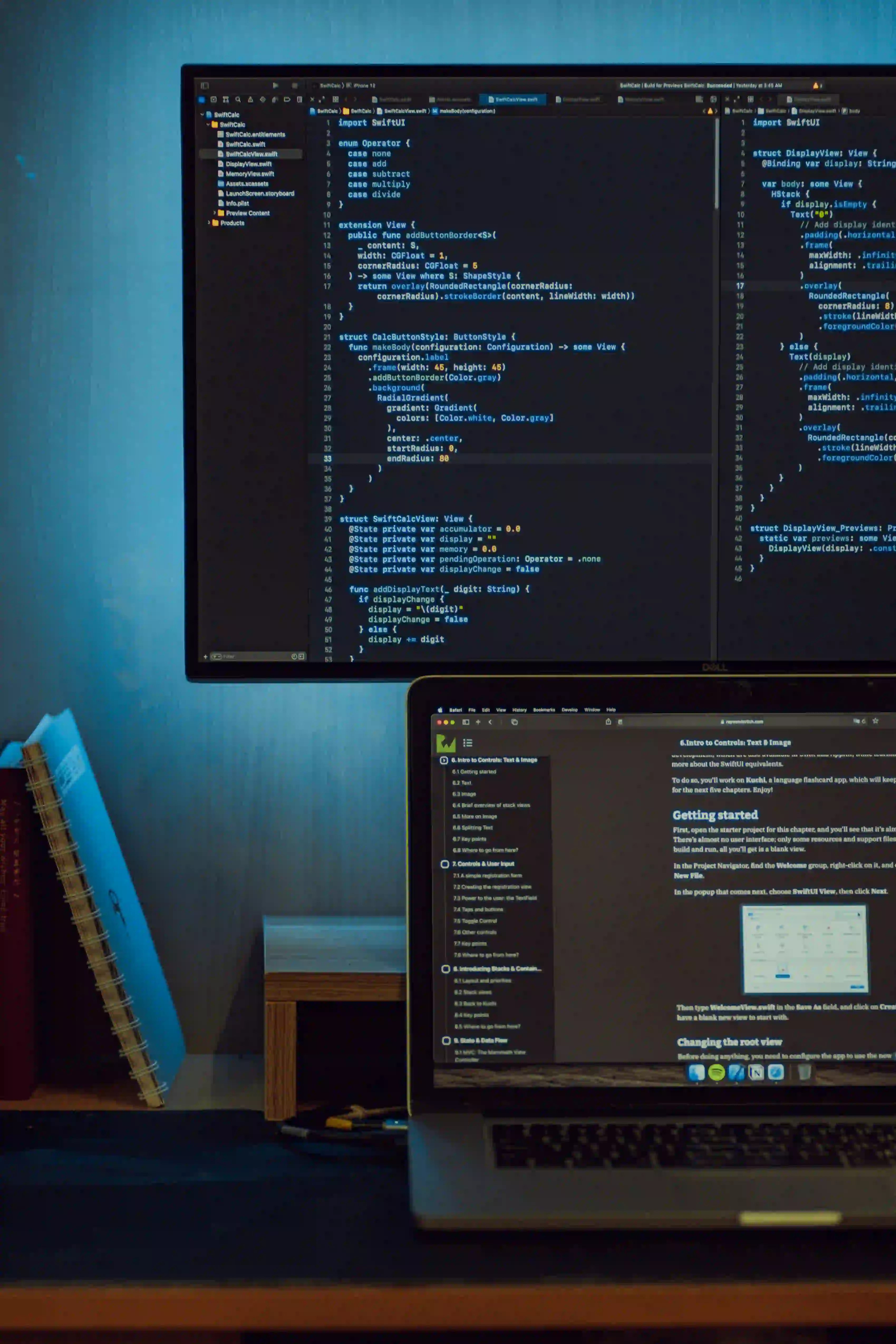
Mastering Apache Thrift: Avoid Beginner Pitfalls
When it comes to building robust and efficient distributed systems, Apache Thrift stands out as a reliable and high-performance framework. With its support for multiple programming languages, Thrift simplifies the process of cross-language development, making it an ideal choice for building microservices and large-scale distributed systems. However, for beginners diving into the world of Apache Thrift, there are common pitfalls that can hinder progress and efficiency. In this post, we'll explore these pitfalls and provide insights into how to avoid them, as well as best practices for mastering Apache Thrift.
Pitfall 1: Neglecting Protocol Definition
One of the fundamental aspects of Apache Thrift is the protocol definition. Neglecting to define a clear and comprehensive protocol can lead to interoperability issues and code inconsistencies across different languages. When defining a protocol, it's crucial to consider the data types, service definitions, and cross-language compatibility. Let's take a look at a simple protocol definition in Apache Thrift for a user service:
namespace java com.example.userservice
struct User {
1: required i32 id,
2: required string username,
3: optional string email
}
service UserService {
User getUserById(1: i32 id),
bool createUser(1: User user)
}
In this example, we define a User
struct with required fields and an optional field. We also define a UserService
with two methods. This clear protocol definition ensures that the data types and service definitions are consistent across different languages.
Pitfall 2: Overlooking Code Generation
Apache Thrift provides a powerful code generation feature that automatically generates client and server code in multiple programming languages based on the defined Thrift protocol. Failing to utilize code generation can result in tedious manual implementation of client-server communication, which not only introduces room for errors but also undermines the efficiency that Thrift offers. Here's an example of using Apache Thrift's code generation with the protocol definition shown earlier:
thrift --gen java userservice.thrift
Running this command generates the necessary Java code for the user service, including the client and server components. Embracing code generation streamlines the development process and ensures consistency across the client and server implementations.
Pitfall 3: Ignoring Versioning and Backward Compatibility
As distributed systems evolve, versioning and backward compatibility become critical factors. Ignoring versioning can lead to communication breakdowns between older and newer versions of services, causing compatibility issues. Apache Thrift offers built-in support for versioning through its protocol definition, enabling developers to introduce new fields, methods, or data types while maintaining backward compatibility. Consider the following example of adding a new field to the User
struct while ensuring backward compatibility:
struct User {
1: required i32 id,
2: required string username,
3: optional string email,
4: optional bool active
}
By making the new field optional, existing clients can still communicate with the updated service without any breaking changes. Embracing versioning and backward compatibility from the early stages of development ensures a smooth evolution of distributed systems built with Apache Thrift.
Pitfall 4: Neglecting Error Handling and Exception Propagation
Error handling is a crucial aspect of building reliable and resilient distributed systems. Neglecting proper error handling and exception propagation can result in unpredictable system behavior and make debugging a challenging task. Apache Thrift provides support for defining exceptions in the protocol, allowing for structured error handling across different languages. Consider the following example of defining a custom exception for user service in Apache Thrift:
exception UserNotFoundException {
1: string message
}
service UserService {
User getUserById(1: i32 id) throws (1: UserNotFoundException ex)
}
In this example, we define a UserNotFoundException
that can be thrown from the getUserById
method. By explicitly defining exceptions in the protocol, developers can ensure consistent error handling and propagation across different language implementations.
Best Practices for Mastering Apache Thrift
Now that we've explored common pitfalls in Apache Thrift, let's highlight some best practices for mastering this powerful framework:
- Thoroughly Define the Protocol: Take the time to carefully define the protocol, considering data types, service definitions, and cross-language compatibility.
- Utilize Code Generation: Embrace Apache Thrift's code generation feature to automate the generation of client and server code in multiple programming languages.
- Versioning and Backward Compatibility: Implement versioning strategies from the early stages of development to ensure smooth evolution of distributed systems.
- Robust Error Handling: Define and propagate exceptions in the protocol to ensure structured error handling across different language implementations.
By adhering to these best practices and avoiding common pitfalls, developers can harness the full potential of Apache Thrift in building scalable, efficient, and interoperable distributed systems.
In conclusion, Apache Thrift offers a powerful framework for building distributed systems, but beginners need to be mindful of common pitfalls such as neglecting protocol definition, overlooking code generation, ignoring versioning and backward compatibility, and neglecting error handling. By following best practices and embracing the core features of Apache Thrift, developers can navigate these pitfalls and master the art of building robust and efficient distributed systems.