Mastering RxJava: Turning BlockingQueue into Observables!
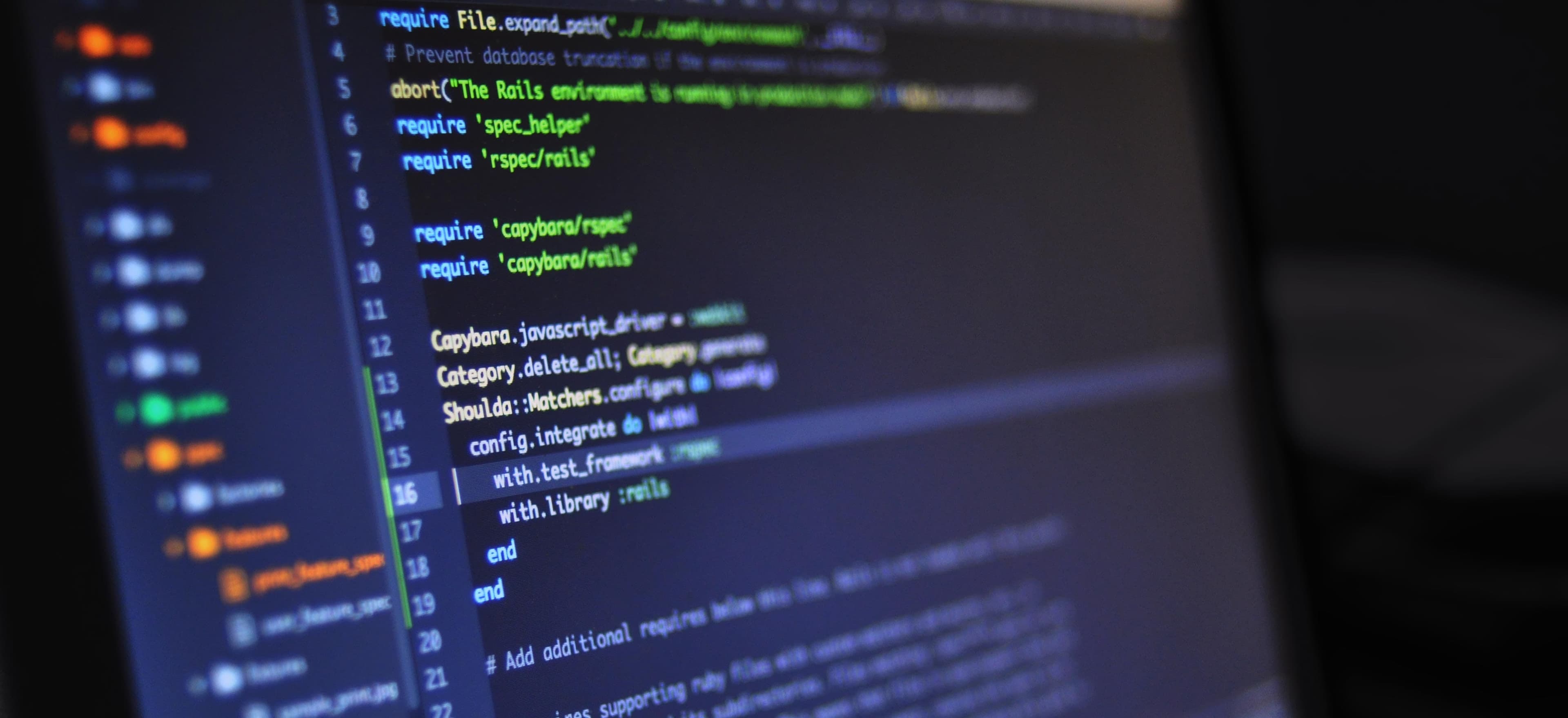
- Published on
Mastering RxJava: Turning BlockingQueue into Observables
In this blog post, we will explore the powerful world of RxJava and how it can be leveraged to turn a BlockingQueue into Observables. We will delve into the concept of Reactive Programming and showcase the seamless transformation of data streams using RxJava. By the end of this post, you will have a solid understanding of how to apply RxJava to convert BlockingQueue operations into reactive streams.
The Opening Bytes to RxJava
RxJava is a popular library for composing asynchronous and event-based programs using observable sequences. It provides a functional and reactive programming paradigm that enables developers to work with asynchronous data streams. The core building block of RxJava is the Observable, which represents a sequence of events that can be observed by interested parties.
The Power of Observables
Observables in RxJava allow for the creation of data streams that can be transformed, filtered, combined, and consumed efficiently. This programming model promotes the propagation of changes through the system and enables the creation of responsive and scalable applications.
Transforming BlockingQueue into Observables
Let's consider a scenario where we have a BlockingQueue containing data that we want to transform into Observables using RxJava. This can be achieved by creating custom Observables that emit items from the BlockingQueue and handle backpressure, concurrency, and error handling seamlessly.
Setting Up the Dependencies
To begin, ensure that you have the RxJava library added to your project's dependencies. If you're using Maven, you can include RxJava with the following snippet in your pom.xml
file:
<dependency>
<groupId>io.reactivex.rxjava2</groupId>
<artifactId>rxjava</artifactId>
<version>2.2.19</version> <!-- Replace with the latest version -->
</dependency>
For Gradle users, include the RxJava dependency in your build.gradle
:
implementation 'io.reactivex.rxjava2:rxjava:2.2.19' // Replace with the latest version
Creating an Observable from BlockingQueue
We can create an Observable from a BlockingQueue by using the create
method provided by RxJava. This method allows us to generate custom observable sequences. Let's take a look at how this can be accomplished in the following code snippet:
import io.reactivex.Observable;
import java.util.concurrent.BlockingQueue;
public class BlockingQueueToObservable {
public Observable<String> convertBlockingQueueToObservable(BlockingQueue<String> blockingQueue) {
return Observable.create(emitter -> {
while (!emitter.isDisposed()) {
try {
String item = blockingQueue.take();
emitter.onNext(item);
} catch (InterruptedException e) {
emitter.onError(e);
Thread.currentThread().interrupt();
}
}
});
}
}
In this code, we create a custom method convertBlockingQueueToObservable
that takes a BlockingQueue
as input and returns an Observable
emitting items from the queue. Within the create
method, we continuously take items from the BlockingQueue
and emit them using the onNext
method. Additionally, we handle potential errors by invoking onError
in case of interruptions.
Subscribing to the Observable
Once we have the Observable representing our BlockingQueue data, we can now subscribe to it to consume the emitted items. Here's an example of how we can subscribe to the created Observable and process the emitted items:
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.BlockingQueue;
public class Main {
public static void main(String[] args) {
BlockingQueue<String> blockingQueue = new ArrayBlockingQueue<>(10);
BlockingQueueToObservable converter = new BlockingQueueToObservable();
Observable<String> observable = converter.convertBlockingQueueToObservable(blockingQueue);
observable.subscribe(
item -> System.out.println("Received item: " + item),
Throwable::printStackTrace,
() -> System.out.println("Completed")
);
// Add items to the blocking queue
blockingQueue.add("First item");
blockingQueue.add("Second item");
// ...
}
}
In this example, we initialize a BlockingQueue
and convert it to an Observable
using our custom conversion method. We then subscribe to the Observable and specify the actions for processing the emitted items, encountering errors, and handling completion.
A Final Look
In this blog post, we have witnessed the seamless transformation of a BlockingQueue into Observables using RxJava. We explored the process of creating custom Observables and subscribing to them for consuming the emitted data. By understanding and applying these principles, you can effectively harness the power of RxJava to handle asynchronous data streams in a reactive and efficient manner.
To delve deeper into RxJava, consider exploring its documentation and trying out more advanced features such as operators for data manipulation, combining multiple streams, and handling concurrency. By mastering RxJava and its capabilities, you can elevate your programming skills and develop robust, responsive applications.
In conclusion, RxJava presents a paradigm shift in handling asynchronous operations. By grasping its concepts and implementation, developers can streamline their code, enhance reactivity, and build more responsive and efficient applications.
Start transforming your data streams today with RxJava and witness the power of reactive programming in action!
Be sure to check out the official RxJava GitHub repository for more in-depth information and resources. Happy coding!