Unlocking Productivity: Solving Code Generation Complexity
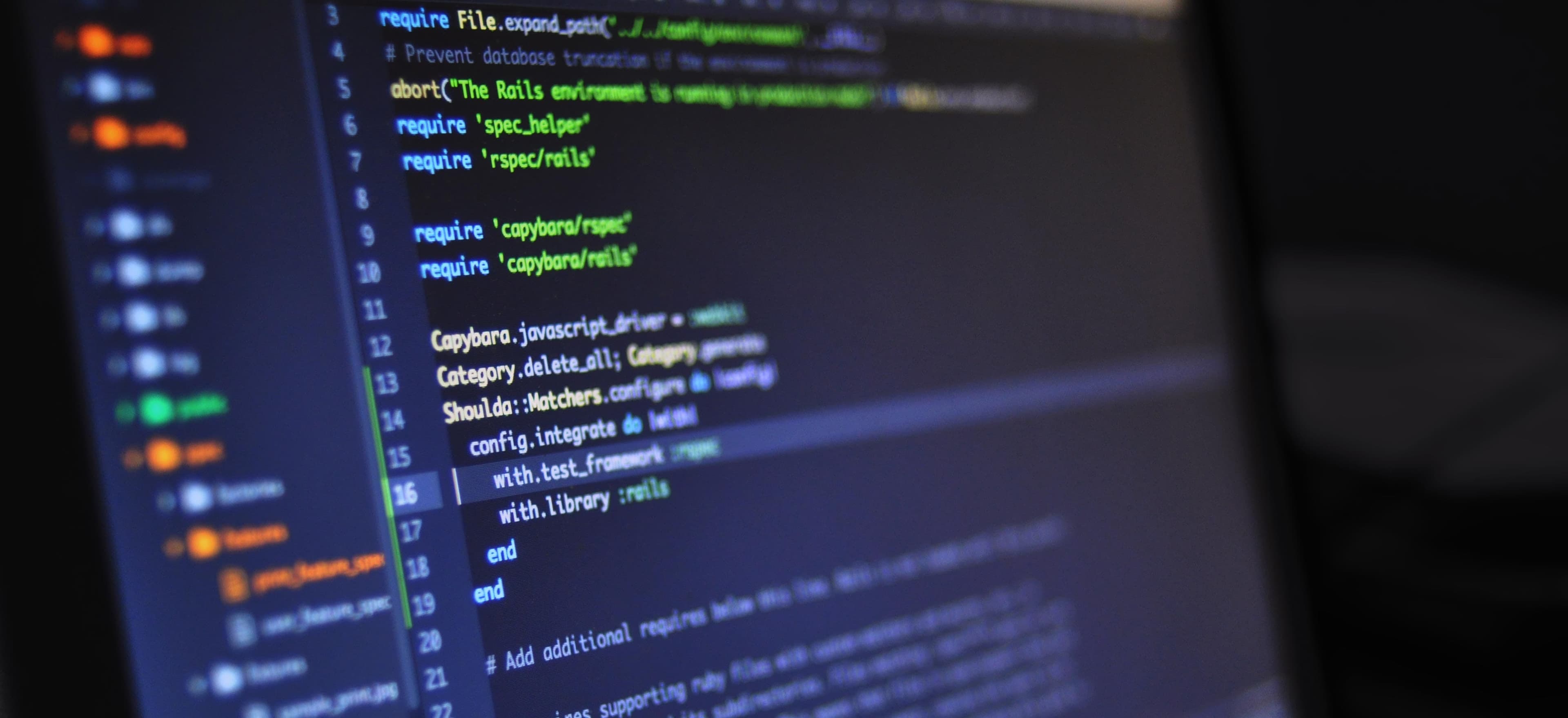
- Published on
Unlocking Productivity: Solving Code Generation Complexity
Essentials at a Glance
In the fast-paced world of software development, productivity is a precious commodity. However, developers often face daunting challenges with code generation complexity, especially in the Java ecosystem. Java code generation, when harnessed effectively, can significantly enhance productivity by automating repetitive tasks and reducing boilerplate code. In this article, we'll delve into the intricacies of code generation in Java, covering both static and dynamic approaches, exploring popular tools and libraries, discussing best practices, and highlighting common pitfalls and solutions.
The Basics of Code Generation in Java
Code generation in Java involves the automatic creation of source code, typically to simplify repetitive tasks and reduce manual effort. This can be achieved through static or dynamic approaches.
Static Code Generation
Static code generation occurs at compile time and is commonly facilitated by annotation processors. Let's consider a basic example where an annotation processor generates a class based on user-defined annotations. Below is a simplified demonstration:
// Define a custom annotation
@Retention(RetentionPolicy.SOURCE)
@Target(ElementType.TYPE)
public @interface GenerateHelper {
String value();
}
// Processor to generate a helper class
@SupportedAnnotationTypes("com.example.GenerateHelper")
@SupportedSourceVersion(SourceVersion.RELEASE_8)
public class HelperProcessor extends AbstractProcessor {
@Override
public boolean process(Set<? extends TypeElement> annotations, RoundEnvironment roundEnv) {
for (TypeElement annotation : annotations) {
// Generate a helper class based on annotation information
// ... (implementation details here)
}
return true;
}
}
In this example, the GenerateHelper
annotation triggers the HelperProcessor
to generate a helper class at compile time based on the provided information.
Dynamic Code Generation
Dynamic code generation, on the other hand, occurs at runtime and is commonly associated with techniques like reflection. An example of dynamic code generation involves creating proxies using Java's reflection API. Here's a simple demonstration:
// Interface to be proxied
public interface DataService {
void performOperation();
}
// Create a dynamic proxy
public class ProxyExample {
public static void main(String[] args) {
DataService realDataService = new RealDataService();
DataService proxyDataService = (DataService) Proxy.newProxyInstance(
ProxyExample.class.getClassLoader(),
new Class[]{DataService.class},
(proxy, method, arguments) -> {
// Perform additional operations before delegating to the realDataService
return method.invoke(realDataService, arguments);
});
// Use the proxy to perform the operation
proxyDataService.performOperation();
}
}
In this example, a dynamic proxy is dynamically created to intercept method invocations on the DataService
interface and perform additional operations before delegating to the actual implementation.
Tools and Libraries for Code Generation in Java
Several tools and libraries exist in the Java ecosystem to aid in code generation. Let's explore some of the popular ones, such as Lombok, MapStruct, and Byte Buddy.
Lombok
Project Lombok is a popular library that aims to reduce boilerplate code in Java. It achieves this by introducing annotations to generate commonly used code blocks. For instance, the @Getter
and @Setter
annotations can be used to automatically generate getter and setter methods for a class, respectively.
import lombok.Getter;
import lombok.Setter;
public class User {
@Getter @Setter
private String name;
// Other class members
}
In this example, Lombok's @Getter
and @Setter
annotations eliminate the need for manually declaring getter and setter methods for the name
field.
MapStruct
MapStruct is a code generator that simplifies the implementation of mappings between Java bean types. It generates mapping code at compile time based on defined mapper interfaces. Consider the following example:
@Mapper
public interface UserMapper {
UserDto toDto(User user);
User fromDto(UserDto userDto);
}
In this example, the UserMapper
interface specifies the mappings between User
and UserDto
objects. MapStruct generates the corresponding mapping implementation, reducing the need for boilerplate mapping code.
Byte Buddy
Byte Buddy is a dynamic code generation library that allows developers to create Java classes and modify existing classes at runtime. It offers a higher level of abstraction compared to traditional reflection-based approaches. Here's a simple example of using Byte Buddy to create a new dynamic class at runtime:
DynamicType.Unloaded<?> dynamicType = new ByteBuddy()
.subclass(Object.class)
.method(named("toString"))
.intercept(FixedValue.value("Hello, Byte Buddy!"))
.make();
Class<?> dynamicClass = dynamicType.load(getClass().getClassLoader())
.getLoaded();
Object instance = dynamicClass.newInstance();
System.out.println(instance.toString());
In this example, Byte Buddy is used to create a dynamic subclass of Object
with a custom implementation of the toString
method.
Best Practices for Code Generation
While code generation can offer significant benefits, there are several best practices to follow when incorporating it into a project.
Managing Generated Code in Your Project
It is crucial to properly manage generated code within a project. This includes separating generated code from hand-written code, organizing it into dedicated packages or directories, and ensuring it is correctly handled by version control systems. Additionally, leveraging build tools like Maven or Gradle to integrate code generation seamlessly into the build process is essential for maintaining a clean and manageable codebase.
Common Pitfalls and How to Avoid Them
Despite the advantages of code generation, certain pitfalls can hinder its effectiveness. These include overuse of code generation, neglecting performance implications, and difficulties in debugging generated code. To mitigate these issues, it's crucial to strike a balance between generated and hand-written code, thoroughly profile and test generated artifacts, and provide comprehensive documentation for generated code to aid in debugging.
Key Takeaways
In this article, we've explored the intricacies of Java code generation, encompassing both static and dynamic approaches, popular tools and libraries, best practices, and common pitfalls. When utilized effectively, code generation can amplify developer productivity by automating repetitive tasks and reducing boilerplate code. By following best practices and understanding the potential pitfalls, developers can harness the power of code generation to streamline their Java projects.
Call to Action
We encourage readers to explore the tools and techniques discussed in this article and integrate code generation into their Java projects where applicable. Share your experiences with code generation and discover how it can revolutionize your development workflow. Stay tuned for more insightful posts on Java development by subscribing to our blog or following us on social media.