Mastering Geb Spock Tests in Maven: A Simple Guide
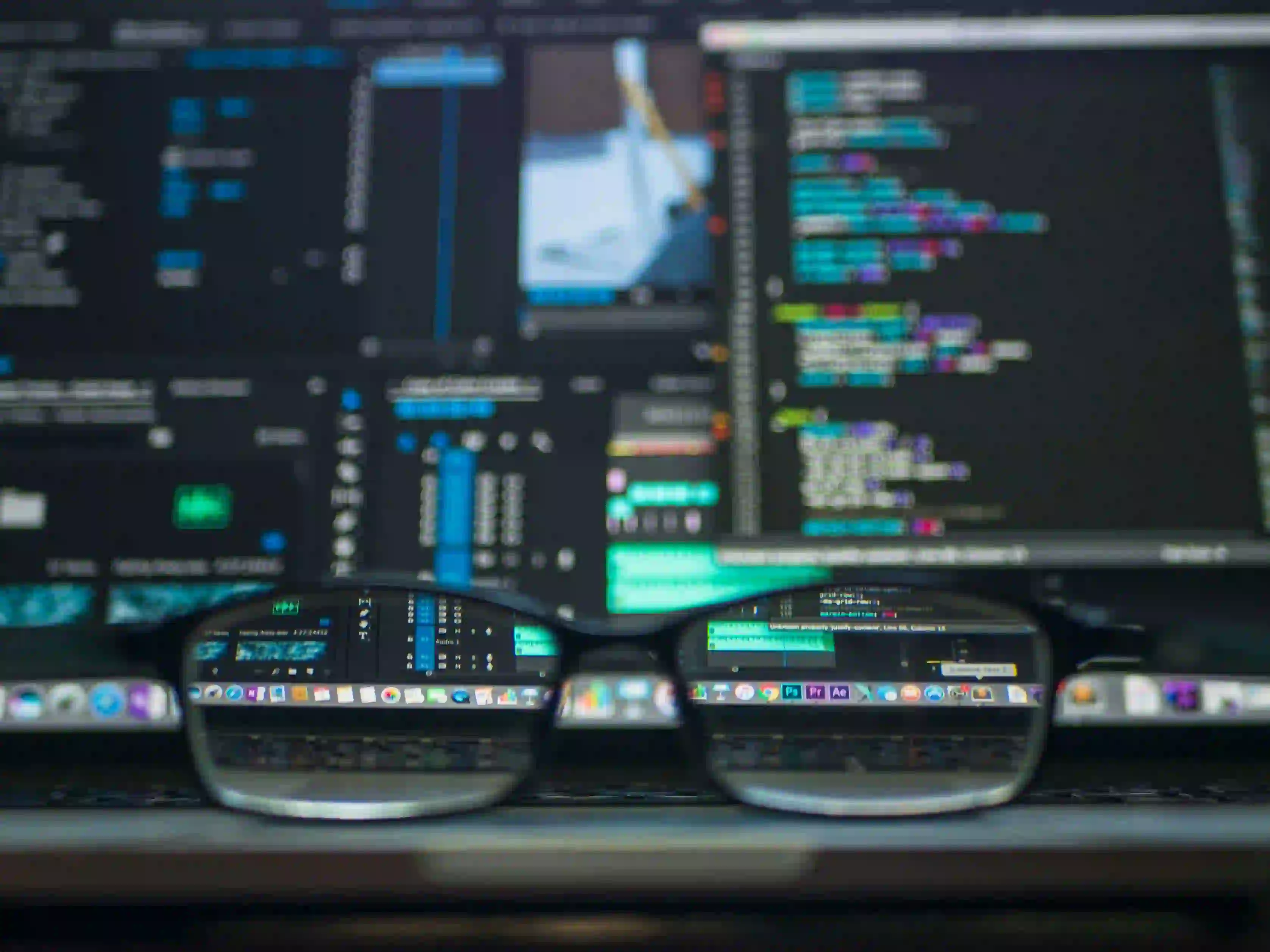
Mastering Geb Spock Tests in Maven: A Simple Guide
When it comes to automated testing in Java, Geb and Spock are a powerful combination that provides a clean and expressive way to write functional tests. In this blog post, we will explore how to set up and master Geb Spock tests in a Maven project. By the end of this guide, you will have a solid understanding of how to write and run Geb Spock tests within your Maven projects.
Setting Up the Maven Project
First, let's set up a new Maven project. You can use your favorite IDE or run the following command in your terminal to create a new Maven project:
mvn archetype:generate -DgroupId=com.example -DartifactId=geb-spock-demo -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
This will create a basic Maven project structure for you. Next, let's add the necessary dependencies for Geb and Spock to our project's pom.xml
:
<dependencies>
<!-- Other dependencies -->
<dependency>
<groupId>org.gebish</groupId>
<artifactId>geb-core</artifactId>
<version>4.1.0</version>
</dependency>
<dependency>
<groupId>org.codehaus.geb</groupId>
<artifactId>geb-spock</artifactId>
<version>4.1.0</version>
</dependency>
<dependency>
<groupId>org.spockframework</groupId>
<artifactId>spock-core</artifactId>
<version>2.0-M3-groovy-3.0</version>
<scope>test</scope>
</dependency>
</dependencies>
After adding these dependencies, Maven will fetch them from the respective repositories and make them available for our project. With the project set up, let's move on to creating our first Geb Spock test.
Writing a Geb Spock Test
Assuming you have a web application running locally on http://localhost:8080
, let's write a simple Geb Spock test to interact with the application.
Create a new test class, such as GebSpockTest
, in the src/test/groovy
directory of your Maven project. Here's an example of a Geb Spock test that navigates to a web page, enters a search term, and verifies the search results:
import geb.spock.GebSpec
import spock.lang.*
class GebSpockTest extends GebSpec {
def "searching for a term on the website"() {
when:
to HomePage
searchField.value("Geb Spock")
searchButton.click()
then:
at SearchResultsPage
searchResults.size() > 0
}
}
In this test, we extend GebSpec
to use Geb's functionalities and utilize Spock's BDD-style syntax for test cases. We navigate to the HomePage
, enter the search term "Geb Spock" into the search field, click the search button, and then verify that the search results page is displayed with at least one search result.
Running Geb Spock Tests
To run Geb Spock tests in a Maven project, we need to configure the maven-surefire-plugin to execute our Spock tests. Add the following plugin configuration to the build
section of your pom.xml
:
<build>
<plugins>
<!-- Other plugins -->
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M5</version>
<configuration>
<systemPropertyVariables>
<geb.build.reportsDir>build/test-reports/geb</geb.build.reportsDir>
</systemPropertyVariables>
<includes>
<include>**/*Test.*</include>
</includes>
</configuration>
</plugin>
</plugins>
</build>
This configuration tells Maven to include all test classes ending with "Test" in their file names. Now, when you run mvn test
, Maven will execute your Geb Spock tests.
Lessons Learned
In this guide, we've walked through the process of setting up and mastering Geb Spock tests in a Maven project. With the power of Geb's browser automation and Spock's expressive testing syntax, you can create comprehensive functional tests for your web applications. Incorporating Geb and Spock into your Maven projects enables you to automate your testing process efficiently and effectively.
Now that you have a solid foundation, feel free to explore more advanced features and techniques offered by Geb and Spock to further enhance your automated testing capabilities. Happy testing!
Start mastering Geb Spock tests in Maven by setting up a basic project and writing your first Geb Spock test. As you become more comfortable with the Geb Spock framework, you can further enhance your testing capabilities by exploring advanced features and techniques.
To dive deeper into Geb and Spock, consider reading the official documentation:
Now, go forth and automate your testing process with confidence using Geb and Spock in your Maven projects!