Mastering Mockito: Avoiding Static Imports in Java 8
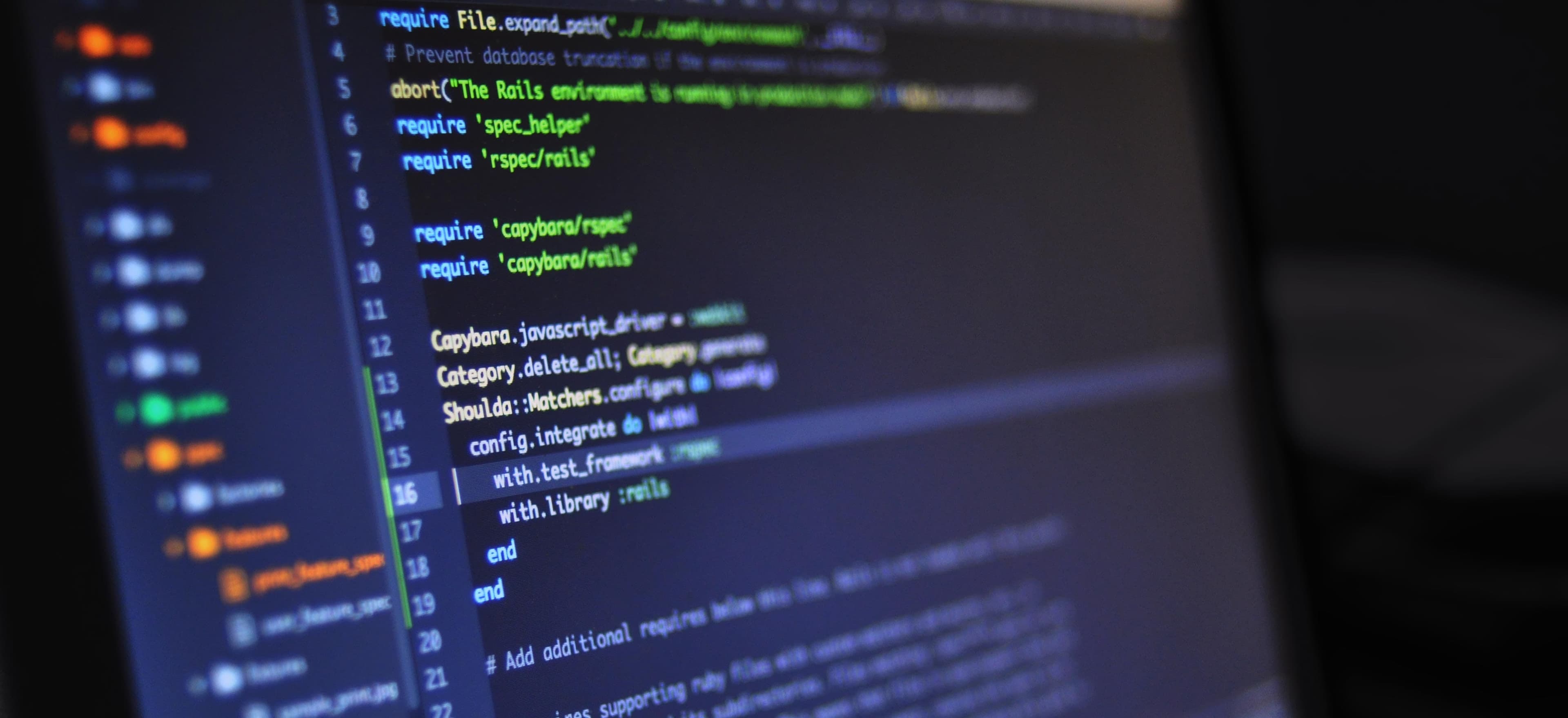
- Published on
Mastering Mockito: Avoiding Static Imports in Java 8
Mockito is a powerful mocking framework in Java that simplifies the testing of Java applications, particularly when dealing with external dependencies and isolated components. While static imports can make your test code cleaner by omitting class names, they can also introduce confusion and reduce readability, especially in larger projects. In this post, we will explore the nuances of avoiding static imports in Mockito when coding with Java 8, along with clear examples and best practices.
Why Avoid Static Imports?
Static imports allow you to use static members from classes without qualifying them with the class name. While this can make your code less verbose, it can also lead to several issues:
- Readability: When multiple static imports are used from different classes, it may become hard to decipher where a method comes from, particularly for those unfamiliar with the codebase.
- Maintainability: If the method's signature changes or the method is moved to another class, you may miss these modifications, resulting in potential runtime errors.
- Navigability: IDEs may not provide sufficient context to understand which utility class a static method belongs to, making it harder for new developers to onboard onto your project.
Therefore, when writing tests with Mockito, embracing fully qualified class names might be the preferred approach for clarity and maintainability.
Setting Up Mockito
Before we start diving into examples, let’s set up a simple Java project with Mockito. If you haven't added Mockito to your Java project yet, ensure that you include it in your Maven or Gradle build configuration.
Maven Configuration
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>5.0.0</version>
<scope>test</scope>
</dependency>
Gradle Configuration
testImplementation 'org.mockito:mockito-core:5.0.0'
Once you have Mockito set up, let’s look at practical usage examples.
Example: Mocking a Simple Service
Let’s create a simple service that our tests can call. Here is a hypothetical UserService
that interacts with a UserRepository
.
User Service
public class UserService {
private UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUserById(Long id) {
return userRepository.findById(id);
}
}
User Repository
public interface UserRepository {
User findById(Long id);
}
Writing Tests Without Static Imports
When writing our tests, we will choose to avoid static imports to maintain clarity. Here's how you might write a unit test for the UserService
class.
UserServiceTest Class
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import org.mockito.Mockito;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class UserServiceTest {
private UserService userService;
private UserRepository userRepository;
@BeforeEach
public void setUp() {
// Creating the mock instance of UserRepository
userRepository = Mockito.mock(UserRepository.class);
userService = new UserService(userRepository);
}
@Test
public void testGetUserById() {
// Given
User expectedUser = new User(1L, "John Doe");
Mockito.when(userRepository.findById(1L)).thenReturn(expectedUser);
// When
User actualUser = userService.getUserById(1L);
// Then
assertEquals(expectedUser, actualUser);
}
}
Why This Matters
- Explicitness: Each Mockito method is richly described by its full name, making it clear that
Mockito.mock(UserRepository.class)
is creating a mock object specific toUserRepository
. - Context: Using fully qualified names keeps you aware of where methods are coming from, mitigating confusion among various static imports.
What About Other Mockito Features?
Summary assertions and verifications using Mockito can also follow the same principle. We can verify interactions with mocks while avoiding static imports.
Verifying Method Interactions
Here’s a revised version of the UserServiceTest
class demonstrating verification.
@Test
public void testGetUserById_callsRepositoryFindById() {
// Given
User expectedUser = new User(1L, "John Doe");
Mockito.when(userRepository.findById(1L)).thenReturn(expectedUser);
// When
userService.getUserById(1L);
// Then
Mockito.verify(userRepository).findById(1L);
}
Insights on Verifying Interactions
- Simplicity: You can quickly discern that
Mockito.verify(userRepository).findById(1L);
checks that the method was called on theuserRepository
object. - Accuracy: Thoroughly clarifying the source of the method being called aids in accurately identifying issues during debugging.
To Wrap Things Up
While static imports can streamline your code, their impact on readability, maintainability, and ease of navigation often outweigh the benefits. By opting for fully qualified names in Mockito, you foster a more explicit codebase that can enhance collaboration within large teams and be easier for newcomers to understand.
For additional information on Mockito and best practices, you may want to explore the official Mockito Documentation and JUnit 5 User Guide.
By refraining from static imports, you'll ensure your unit tests maintain a clear and organized structure, paving the way for easier testing, refactoring, and overall development.
In future posts, we will delve into more advanced features of Mockito, including argument matchers and custom matchers, so stay tuned!