Mastering ADF: Solving Task Flow Scope Confusion
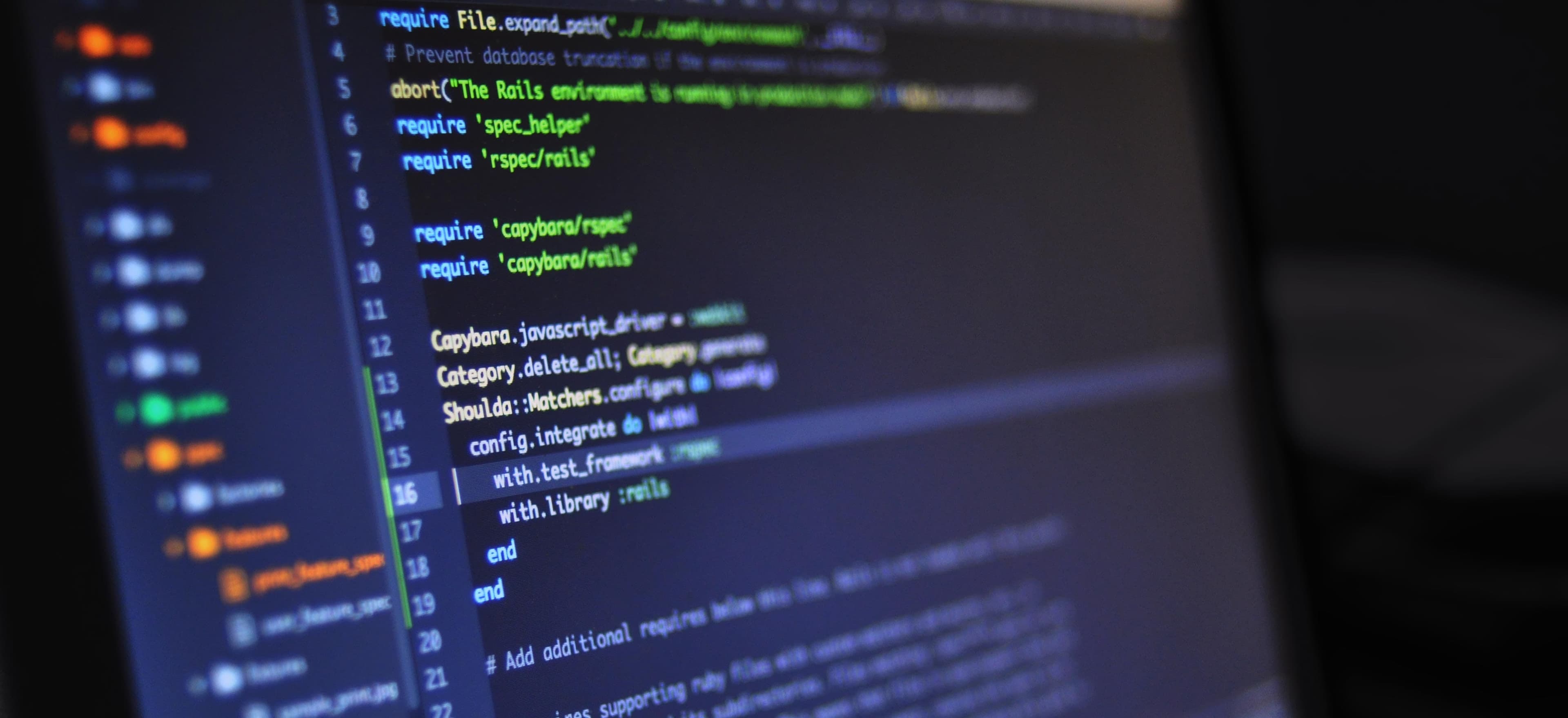
- Published on
Mastering ADF: Solving Task Flow Scope Confusion
When working with Oracle Application Development Framework (ADF), developers often encounter challenges related to task flow scope, leading to confusion and frustration. Understanding and effectively managing task flow scope is crucial for building robust, maintainable ADF applications. In this blog post, we will delve into the complexities of task flow scope in ADF and provide practical solutions to common issues, empowering you to master this aspect of ADF development.
Understanding Task Flow Scope
Task flow scope in ADF determines the lifespan and accessibility of managed beans and task flow parameters within the context of a task flow. ADF provides four types of task flow scope:
-
View Scope: This scope persists during the lifespan of a task flow view activity. It allows data to be maintained across postbacks within the task flow.
-
Backing Bean Scope: This scope is similar to the view scope, but is tied to the backing bean associated with the view. It maintains data specific to the backing bean’s lifespan.
-
Page Flow Scope: This scope persists as long as the task flow is active. It allows data to be accessible across multiple activities within the task flow.
-
Application Module Scope: This scope persists as long as the application module is active. It allows data to be shared across multiple task flows that use the same application module.
Task Flow Scope Pitfalls
One of the primary challenges in dealing with task flow scope in ADF is managing the lifecycle and data persistence of managed beans and task flow parameters across various scopes. Incorrectly managing scope can lead to issues such as data corruption, lack of synchronized state, and memory leaks, ultimately resulting in unreliable application behavior.
Additionally, developers often encounter confusion when deciding which scope to use for managed beans and task flow parameters, leading to inefficiencies and inconsistencies in the application’s architecture. Without a clear understanding of task flow scope, developers may resort to trial and error, further exacerbating the confusion and complexity.
Practical Solutions
To address the complexities and challenges associated with task flow scope in ADF, it is essential to adopt best practices and leverage proven solutions. Let’s explore some practical approaches to effectively manage task flow scope in ADF applications.
1. Clear Scope Allocation
When designing task flows in ADF, it’s crucial to allocate the appropriate scope to managed beans and task flow parameters. By clearly defining the scope based on the data lifespan and accessibility requirements, developers can ensure efficient data management and minimize potential scope-related issues.
2. Utilize Page Flow Scope for Cross-Activity Data
Leveraging the page flow scope is beneficial when data needs to be accessed across multiple activities within a task flow. By using this scope strategically, developers can maintain synchronized state and avoid redundant data initialization, leading to improved performance and maintainability.
public void onPageFlowScopeDataAccess() {
String sharedData = (String)ADFContext.getCurrent()
.getPageFlowScope().get("sharedData");
// Access and manipulate shared data
}
In the above code snippet, we access shared data stored in the page flow scope, enabling seamless data manipulation across activities.
3. Use Application Module Scope for Global Data
For data that needs to be shared across multiple task flows within an application, leveraging the application module scope is ideal. This allows for centralized data management and ensures consistent access to shared resources.
public void onApplicationModuleScopeDataAccess() {
AppModuleAM appModule = (AppModuleAM) Configuration.createRootApplicationModule("AppModuleAM","AppModuleLocal");
String globalData = appModule.getGlobalData();
// Access and manipulate global data
Configuration.releaseRootApplicationModule(appModule,true);
}
The code above demonstrates accessing global data stored in the application module scope, facilitating seamless data manipulation across multiple task flows.
4. Proper Bean Scope Usage
Utilizing the appropriate bean scope, such as view scope or backing bean scope, is crucial to maintain data integrity and avoid conflicts. By carefully selecting the bean scope based on the view’s lifecycle and data requirements, developers can prevent scope-related issues and streamline data management.
@ManagedBean(name = "exampleBean")
@ViewScoped
public class ExampleBean {
// Bean properties and methods
}
In the code snippet, the managed bean is annotated with @ViewScoped
to ensure its data persists throughout the view’s lifespan, maintaining a consistent state.
5. Implement Explicit Scope Management
Implementing explicit scope management through programmatic control of scope activation and deactivation can provide greater flexibility and control over scope lifespan. This approach enables developers to precisely manage the scope boundaries, ensuring efficient resource utilization and optimized data management.
public void onScopeActivation() {
ADFContext.getCurrent().getPageFlowScope().activate();
// Perform scope-specific operations
}
public void onScopeDeactivation() {
ADFContext.getCurrent().getPageFlowScope().passivate();
// Perform scope-specific operations
}
The above code illustrates explicit activation and deactivation of the page flow scope, allowing developers to customize scope management as per specific requirements.
Final Thoughts
Mastering task flow scope in ADF is fundamental for building resilient and efficient applications. By understanding the intricacies of task flow scope and implementing best practices, developers can mitigate scope-related challenges and build maintainable, high-performance ADF applications.
By following the practical solutions and best practices outlined in this post, you can conquer task flow scope confusion and elevate your ADF development skills to the next level. Embracing effective scope management will not only enhance the robustness of your applications but also contribute to a streamlined development process and improved end-user experience.
In conclusion, empowering yourself with the knowledge and strategies to navigate task flow scope intricacies will undoubtedly position you as a proficient ADF developer, equipped to tackle complex application requirements with confidence and expertise.
For further insights and resources on ADF development, refer to the Oracle ADF documentation and community forums, where you can engage with fellow developers and access a wealth of information and support.
Mastering ADF task flow scope is a significant milestone in your journey towards becoming a proficient ADF developer. Embrace the nuances, incorporate best practices, and embark on a journey of building robust, scalable ADF applications.
Now, go forth and conquer the complexities of task flow scope in ADF!