Boost Your App: Mastering Android Location Services!
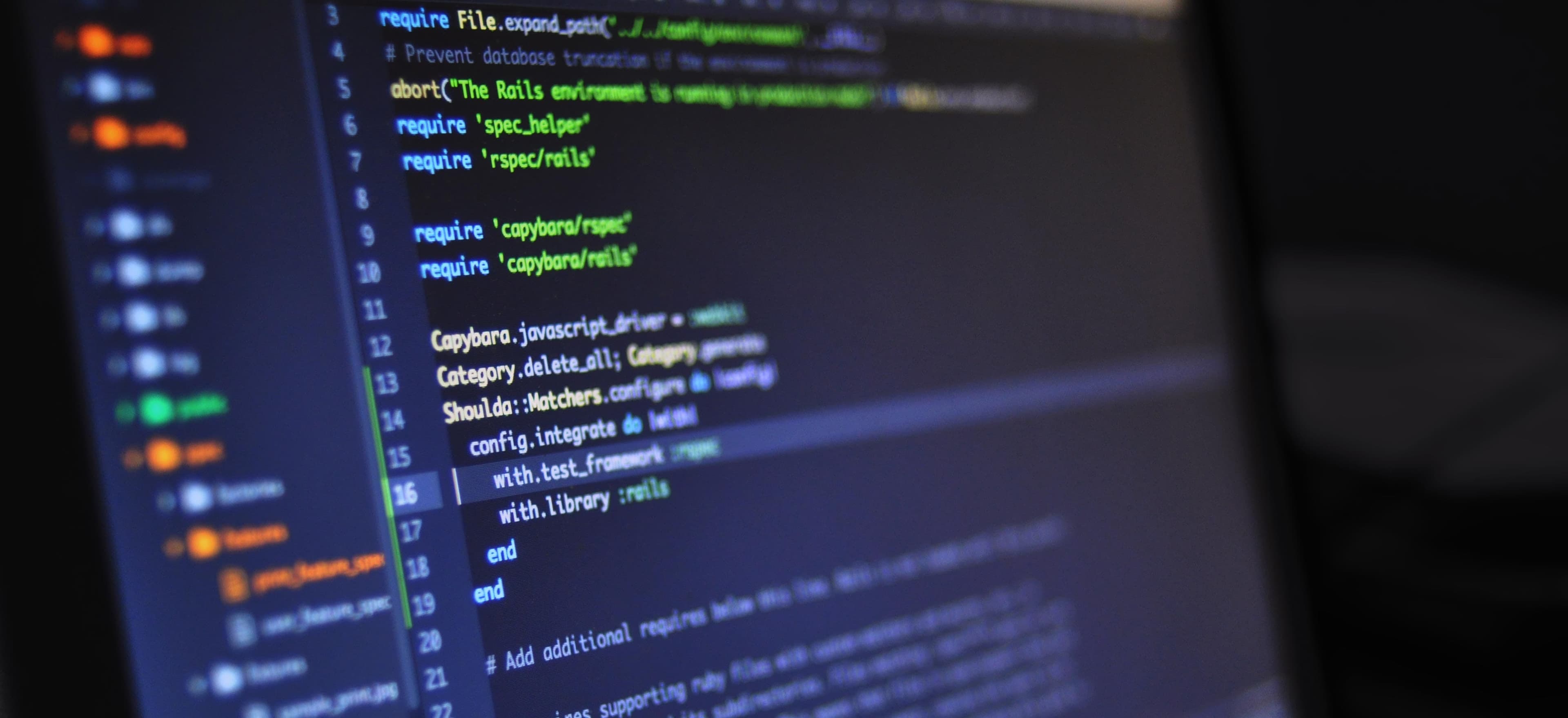
- Published on
Boost Your App: Mastering Android Location Services!
The Roadmap
In the ever-evolving landscape of mobile apps, the integration of location-based services has become ubiquitous. From finding the nearest coffee shop to tracking runs and monitoring fitness goals, location services have revolutionized how users interact with applications. In the realm of Android development, mastering the intricacies of location services is crucial for delivering personalized experiences and enhancing user engagement.
Android Location Services encompass a suite of APIs and tools that empower developers to access, utilize, and respond to location data, enabling them to offer contextually relevant content and features. With the increasing demand for location-aware applications, understanding and effectively implementing Android Location Services is key to producing high-quality, user-centric apps.
Why Use Android Location Services?
The incorporation of Android Location Services brings a multitude of benefits to the table, enriching user experiences and amplifying app functionality. By leveraging location data, apps can:
- Enhance user engagement by providing tailored, location-specific content and services.
- Personalize user experiences by customizing app features based on the user's geographical context.
- Improve app functionality by offering location-based capabilities like navigation, geotagging, and real-time tracking.
App categories across various domains, including travel, fitness, retail, and social networking, significantly benefit from harnessing the power of Android Location Services. Whether it’s suggesting nearby attractions in a travel app or mapping running routes in a fitness app, the seamless integration of location services elevates the app's utility and relevance.
Understanding Location Services in Android
The Location API
At the core of Android Location Services lies the Location API, which is furnished by Google Play Services. This comprehensive API equips developers with tools for accurately retrieving and utilizing location data within their apps. The Location API offers advantages such as:
- Enhanced accuracy through the utilization of multiple sensors and data sources.
- Efficient battery consumption techniques, ensuring that the app's use of location services doesn't unduly drain the device's battery.
Permissions
To access location services in Android, apps must explicitly declare permissions in the Android manifest. The two primary location-related permissions are:
ACCESS_FINE_LOCATION
: Grants access to precise user location, utilizing GPS and other high-accuracy methods.ACCESS_COARSE_LOCATION
: Provides access to approximate user location through less precise methods, such as cell tower triangulation.
It’s vital to prioritize user privacy and transparency when requesting location permissions, ensuring that users are informed about why the app requires access to their location data.
Fetching Location: A Step-by-Step Guide
Step 1: Add Dependencies
The first step in integrating Android Location Services is to include the necessary dependencies in the app's build.gradle
file to access the Location API.
implementation 'com.google.android.gms:play-services-location:17.0.0'
Furthermore, keeping dependencies up-to-date is crucial to leverage the latest features and improvements offered by the Location API.
Step 2: Declare Permissions in Manifest
In the app's manifest file, the required location permissions must be declared:
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
For apps targeting Android 6.0 (API level 23) and above, it’s essential to implement runtime permissions to request access to location services from the user at runtime.
Step 3: Check for Permissions
Apps need to programmatically check for the availability of location permissions and request them if not already granted. This can be achieved using the ActivityCompat
API.
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.ACCESS_FINE_LOCATION}, REQUEST_LOCATION_PERMISSION);
}
Subsequently, the app should handle the permission result based on the user's choice.
Step 4: Accessing Location Services
To interact with location services, an instance of the FusedLocationProviderClient
is required, enabling the app to access the fused location provider.
FusedLocationProviderClient locationClient = LocationServices.getFusedLocationProviderClient(this);
Step 5: Receiving Location Updates
Setting up a location request and receiving location updates is accomplished through the use of a LocationCallback
. This involves defining a LocationRequest
with specific parameters such as update interval and accuracy priority.
LocationRequest locationRequest = LocationRequest.create();
locationRequest.setInterval(10000);
locationRequest.setPriority(LocationRequest.PRIORITY_HIGH_ACCURACY);
locationClient.requestLocationUpdates(locationRequest, new LocationCallback() {
@Override
public void onLocationResult(LocationResult locationResult) {
if (locationResult == null) {
return;
}
for (Location location : locationResult.getLocations()) {
// Update UI with location data
// e.g., location.getLatitude(), location.getLongitude()
}
}
}, Looper.getMainLooper());
The adaptability of location request parameters such as update interval and priority allows developers to tailor the usage of location services as per the app's specific requirements.
Best Practices for Using Android Location Services
Optimizing battery life and respecting user privacy are critical aspects of effectively utilizing Android Location Services. Here are some best practices:
-
Optimizing Battery Life:
- Adjust location request parameters, such as update interval and priority, to balance the need for accuracy with minimizing battery consumption.
- Cease location updates when the app no longer requires the data, thereby conserving battery life.
-
Respecting User Privacy:
- Clearly communicate to users the reasons for requesting access to their location.
- Ensure that location data is handled securely and responsibly, with adherence to relevant data protection regulations.
Troubleshooting Common Issues
Integrating and leveraging Android Location Services can sometimes lead to challenges. Some common issues and their resolutions include:
-
Delays in Receiving Location Updates:
- Verify that the app has the necessary permissions to access location data.
- Check for proper configuration of the location request, including intervals and priority settings.
-
Handling Permissions Correctly:
- Validate that both static and runtime location permissions are correctly declared and utilized.
Wrapping Up
Android Location Services play a pivotal role in crafting engaging, personalized, and location-aware applications. By harnessing the power of the Location API, developers can enrich their apps with features that respond contextually to user locations, ultimately elevating the user experience. From fetching location data to optimizing battery usage and respecting user privacy, the journey of mastering Android Location Services is essential for any modern app developer.
In conclusion, the integration of Android Location Services presents immense potential for app development, enabling the creation of dynamic, location-aware experiences. Embracing these tools and adhering to best practices fosters an environment where apps can seamlessly adapt and respond to the ever-changing contexts of their users, resulting in richer, more personalized interactions.
Links and Resources
For further exploration and deeper understanding of Android Location Services, refer to the following resources:
By delving into the official documentation and designated resources, developers can gain comprehensive insights into the nuances of Android Location Services, thus empowering them to create robust and innovative location-enabled applications.